How To Make A Sleep Function In TypeScript?
In TypeScript, there could be many scenarios where a developer might want to pause or sleep a function's execution for a specific time. This function used to be hard to implement, but nowadays, promises make it very simple.
To implement a sleep function in TypeScript, create a helper function and use it when needed.
typescriptconst sleep = (ms) => new Promise(r => setTimeout(r, ms));
console.log('1');
// Sleeps for 2 seconds.
await sleep(2000);
console.log('2');
In this article, I will explain different ways of creating a sleep function in TypeScript and how to implement a sleep function in ES5.
Let's get to it 😎.
How to implement a sleep function?
With a promise (for ES6)
Implementing a sleep function in ES6 is simple using the async/await keywords, a promise, and a setTimeout.
A promise represents an asynchronous operation and its result after its completion. It represents a proxy for a value that may be known in the future.
The setTimeout is a built-in JavaScript function that calls a callback after a specified number of milliseconds have passed.
Here is a one-line implementation example of the sleep function:
typescriptconst sleep = (ms) => new Promise(r => setTimeout(r, ms));
Note: This implementation of the sleep function accepts milliseconds (1 second = 1000 milliseconds).
Then you can use this helper function in your code when needed:
typescriptconsole.log('1');
// Sleeps for 4 seconds.
await sleep(4000);
console.log('2');
With a callback (for ES5)
Unfortunately, you cannot use the async/await keywords in ES5 because this version of JavaScript doesn't support promises.
However, you can still simulate a sleep function using a callback and a setTimeout.
typescriptvar testSleepES5 = function () {
console.log('1');
setTimeout(function () {
console.log('2');
}, 4000);
}
testSleepES5();
In this example, we call the second part of the function after a 4 seconds delay.
As you can see, this is less intuitive than using the async/await keywords but still works.
Final thoughts
In conclusion, it is straightforward to implement a sleep helper function using promises. I recommend placing that function inside your utils folder and importing it when needed.
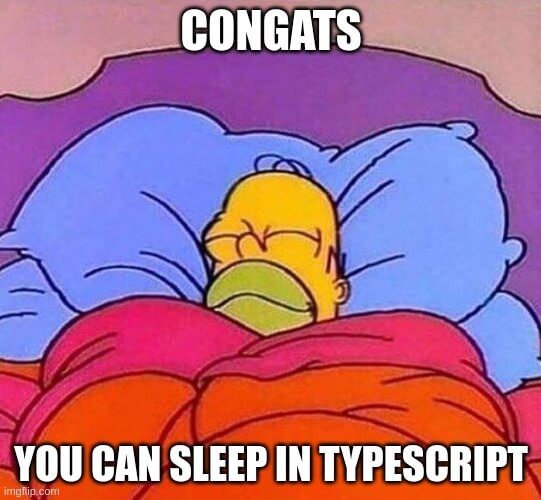
Finally, here are some of my other related tutorials: