How To Set Up A TypeScript Interface Default Value?
In TypeScript, interfaces represent the shape of an object. They support many different features like optional parameters but unfortunately do not support setting up default values. However, you can still set up a TypeScript interface default value by using a workaround.
To set up a default value with an interface, you can use the TypeScript Pick utility type like so:
typescriptinterface IPerson {
name: string;
role: string;
}
const defaults: Pick<IPerson, 'role'> = {
role: 'user'
}
const person: IPerson = {
...defaults,
name: 'Tim'
}
Read the rest of this article to understand precisely how to set up default values with an interface. Let's get to it 😎.
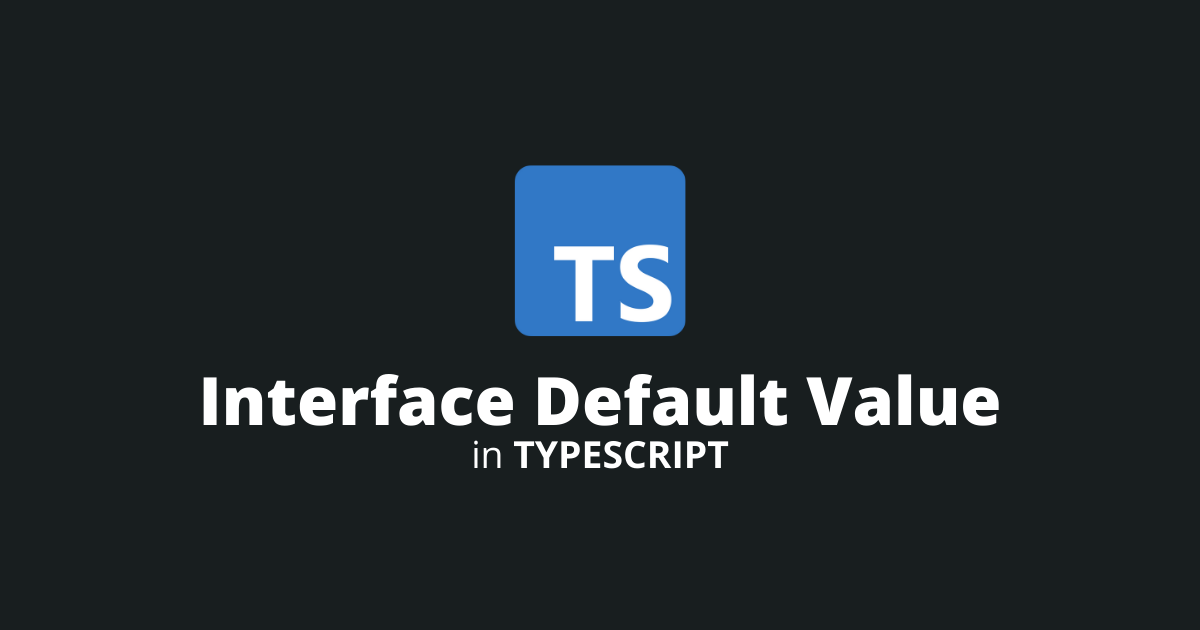
What is an interface?
First and foremost, let's understand what an interface is in TypeScript.
An interface defines the structure of an object. An interface works at compile time and is used for type checking. The TypeScript compiler doesn't covert interfaces to JavaScript.
How to set up default values with an interface?
Since interfaces only work at compile-time, they cannot be used to set up runtime default values.
Luckily, TypeScript provides a workaround for this.
By using the TypeScript pick utility type, we can select properties from an interface and provide default values for them.
First, let's define an example interface:
typescriptinterface IPerson {
firstName: string;
lastName: string;
role: string;
}
Second, let's create a default values object using the Pick utility type.
You need to select the properties you wish to provide defaults for in the Pick type definition. In this example, we choose only the role property:
typescripttype DefaultValues = Pick<IPerson, 'role'>;
const defaultPersonValues: DefaultValues = {
role: 'guest'
}
After, let's create a helper utility type, to make certain properties optional:
typescripttype Optional<T, K extends keyof T> = Pick<Partial<T>, K> & Omit<T, K>;
Note: I've written an extensive guide on the TypeScript keyof operator here.
Then, let's create a new type to make the IPerson properties with default values optional:
typescripttype PersonWithDefaultsOptional = Optional<IPerson, keyof DefaultValues>
Finally, let's create a new person object and only provide the required fields. By using the spread operator, we can then merge the default values with the new values.
typescriptconst newPerson: PersonWithDefaultsOptional = {
firstName: 'Tim',
lastName: 'Mousk'
};
const person: IPerson = {
...defaultPersonValues,
...newPerson
}
Here is the full code for this example.
typescriptinterface IPerson {
firstName: string;
lastName: string;
role: string;
}
type DefaultValues = Pick<IPerson, 'role'>;
const defaultPersonValues: DefaultValues = {
role: 'guest'
}
type Optional<T, K extends keyof T> = Pick<Partial<T>, K> & Omit<T, K>;
type PersonWithDefaultsOptional = Optional<IPerson, keyof DefaultValues>
const newPerson: PersonWithDefaultsOptional = {
firstName: 'Tim',
lastName: 'Mousk'
};
const person: IPerson = {
...defaultPersonValues,
...newPerson
}
Read: A guide on the TypeScript Omit type.
Read: The TypeScript Partial type.
Final thoughts
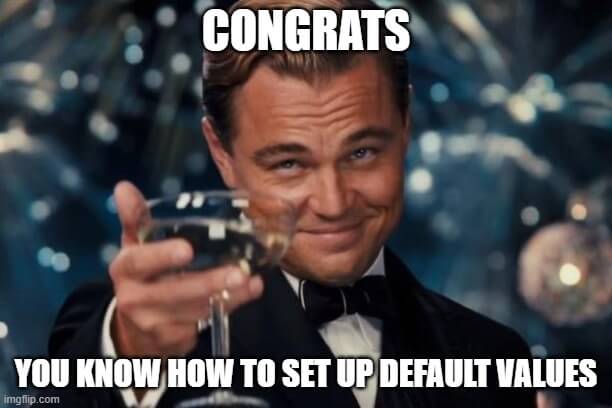
In conclusion, setting up a TypeScript interface default value is easy. Just use the Pick utility type in combination with the ES6 spread operator.
Here is another TypeScript tutorial for you to enjoy: