How does the TypeScript Pick type work?
TypeScript provides a lot of utility types that are going to help you do common type transformations. One of those types is the TypeScript Pick type.
The Pick type is used when you want to construct a type by picking keys from a specific interface.
In this article, you will learn everything to know about the Pick type, as well as some of the use cases of this type.
What is the Pick type?
The Pick TypeScript type is used to simplify type generation by dynamically creating a type from an interface and a set of keys.
Here is an example of the Pick type in action.
typescriptinterface IPerson {
firstName: string;
lastName: string;
age: number;
}
type PersonFullName = Pick<IPerson, 'firstName' | 'lastName'>;
const person: PersonFullName = {
firstName: 'Tim',
lastName: 'Mousk'
};
As you can see, by using the Pick type, we create a new type that only contains the firstName and lastName properties.
To select the desired properties we use the union type '|' operator.
Did you notice that we are using an interface with a type? I've written an article about the differences between a type vs an interface, that will explain why.
The Pick type was released in TypeScript version 2.1.
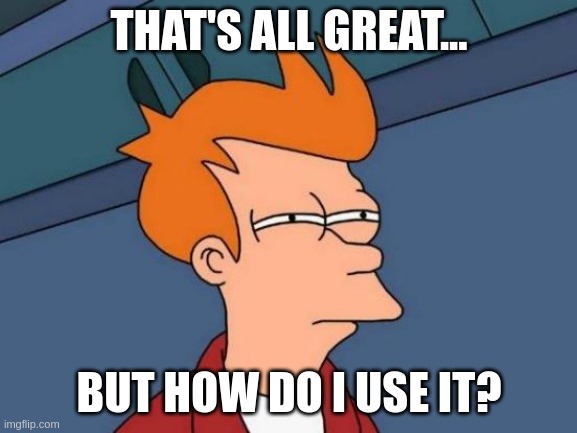
That is all great, you may say... But how do I use it in a real application? 🤔
Let's say, in your application, you have an interface IUser.
typescriptinterface IUser {
userName: string;
firstName: string;
lastName: string;
password: string;
role: string;
age: number;
}
And, you want to create an authentication function that accepts an object that uses some fields from the IUser interface. This is very easy to do with the Pick type.
typescripttype PersonCredentials = Pick<IUser, 'userName' | 'password'>;
Then, if for example, you change a property in the IUser interface, the TypeScript Intellisense will show an error, since the property does not exist anymore.
How to use Pick on a nested object?
Let's suppose you have a nested interface like this.
typescriptinterface IPerson {
firstName: string;
lastName: string;
age: number;
address: {
country: string;
city: string;
street: string;
}
}
How can you pick some keys from this interface? By using indexes.
typescripttype Person = Pick<IPerson, 'firstName' | 'lastName'> & {
address: Array<Pick<IPerson['address'], 'city' | 'street'>>
}
How to use Pick and make the properties optional?
Let's suppose you want to pick some properties from an interface but, want to make them all optional. You can do this by creating a custom type that will use the Omit and Partial built-in TypeScript types.
typescriptinterface IPerson {
firstName: string;
lastName: string;
age: number;
}
type CopyWithPartial<T, K extends keyof T> = Omit<T, K> & Partial<T>;
type PartialPerson = CopyWithPartial<IPerson, 'firstName' | 'lastName'>;
const person: PartialPerson = { firstName: 'Tim' };
Read more: The keyof operator in TypeScript
Conclusion
In conclusion, the Pick type is a nice-to-know feature that will help you make your TypeScript code clearer.
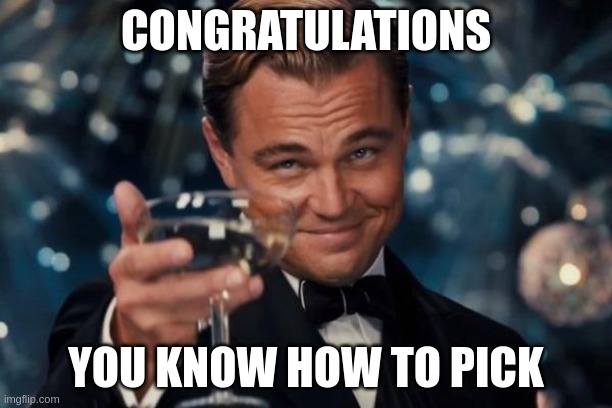
Thank you for reading this article. Please share it.