How To Check The Type In TypeScript?
When developing TypeScript projects, developers often need to check the type of an entity. Luckily, this is easy to do.
This article shows how to check the type of:
Let's get to it 😎.
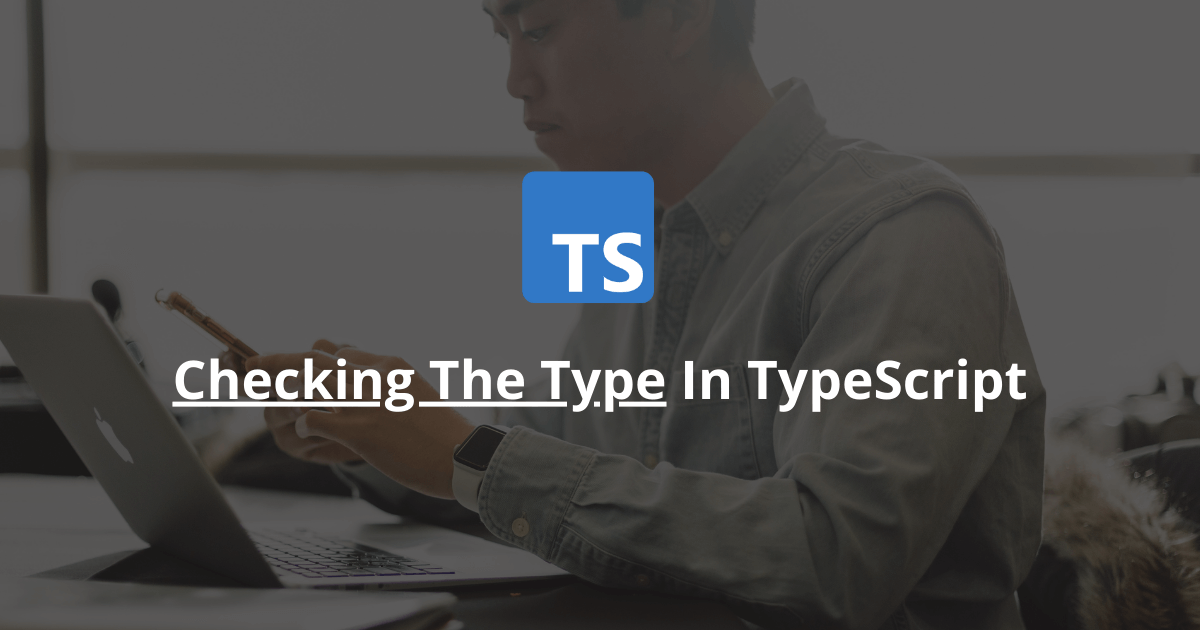
How to check the type of a variable?
To check the type of a variable in TypeScript, you can use the typeof operator.
This operator returns the data type of a variable.
Here are a few examples of the typeof operator in action:
typescript// Outputs: 'string'
console.log(typeof 'dog');
// Outputs: 'boolean'
console.log(typeof false);
// Outputs: 'number'
console.log(typeof 123);
// Outputs: 'object'
console.log(typeof {asd:'asd'});
// Outputs: 'function'
console.log(typeof function () {});
// Outputs: 'function'
console.log(typeof class A {});
// Outputs: 'object'
console.log(typeof null);
// Outputs: 'undefined'
console.log(typeof undefined);
This method is reliable for checking primitive types, but you need to use other methods to check the type of a class or an object.
Note: Here, we are using the JavaScript typeof operator, which is different from the TypeScript typeof operator.
How to check the type of an object?
To check the type of an object, you can use the in keyword to verify if a specific property or method exists inside the object.
Here is an example:
typescriptconst cow = {
giveMilk: () => {
console.log('give milk.')
}
};
const dog = {
bark: () => {
console.log('bark.')
}
};
// Outputs: true
console.log('giveMilk' in cow);
// Outputs: false
console.log('bark' in cow);
In this example, we verify that the object is a cow by checking for the existence of the giveMilk function.
You can also use a tagged or discriminated union to check an object's type.
How to check the type of an interface?
To check the type of a TypeScript interface, you can:
You can read more about checking the type of an interface in this article.
How to check a class type?
To check the type of a class in TypeScript, you can use the instanceof operator.
This operator returns a boolean (true or false) and tests if an object is of the specified object type.
Here is an example:
typescriptclass Dog {
public constructor(
private name: string
) { }
}
const dog = new Dog('doggy');
// Outputs: true
console.log(dog instanceof Dog);
Unfortunately, this method isn't bulletproof and can return false positives.
A safer option to check the class type is to create a class constant with the class name inside the class.
Here is an example:
typescriptclass Dog {
public readonly NAME = 'dog';
public constructor(
private name: string
) { }
}
const dog = new Dog('doggy');
// Outputs: true
console.log(dog.NAME === 'dog');
You can also create a type guard to check for specific methods to test the class type.
Read more: How do readonly properties work in TypeScript?
How to check the type of an array?
To check the type of an array, you need to verify each array's item type individually and look for inconsistent types.
Here is an example:
typescriptconst isArrayOfStrings = (value: unknown): value is string[] => {
return Array.isArray(value)
&& value.every(item => typeof item === "string");
}
// Outputs: true
console.log(isArrayOfStrings(['a','b']));
// Outputs: false
console.log(isArrayOfStrings(['a',2]));
In this example, we verify that each array item is of type string.
Final thoughts
As you can see, checking the type of an entity is easy in TypeScript.
You can choose from multiple options, depending on your situation.
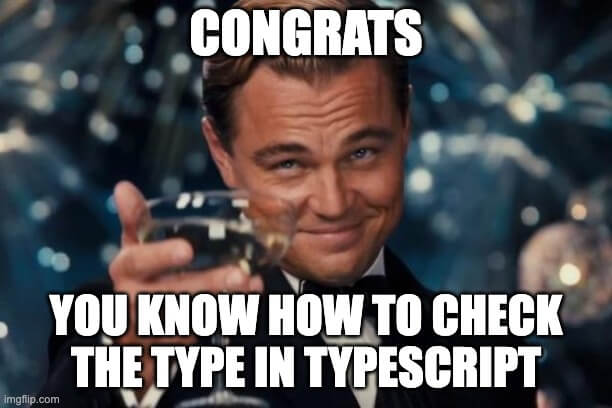
Here are some other TypeScript tutorials for you to enjoy: