How To Make An Arrow Function Generic In TypeScript?
Sometimes, a TypeScript developer wants to make an arrow function accept different types. That's where generic functions come into play.
To make an arrow function generic, you need to add a generic parameter to it like this:
typescriptconst getX = <T,>(x: T) => x;
This article shows how to make an arrow function generic in TypeScript with many code examples.
Let's get to it 😎.
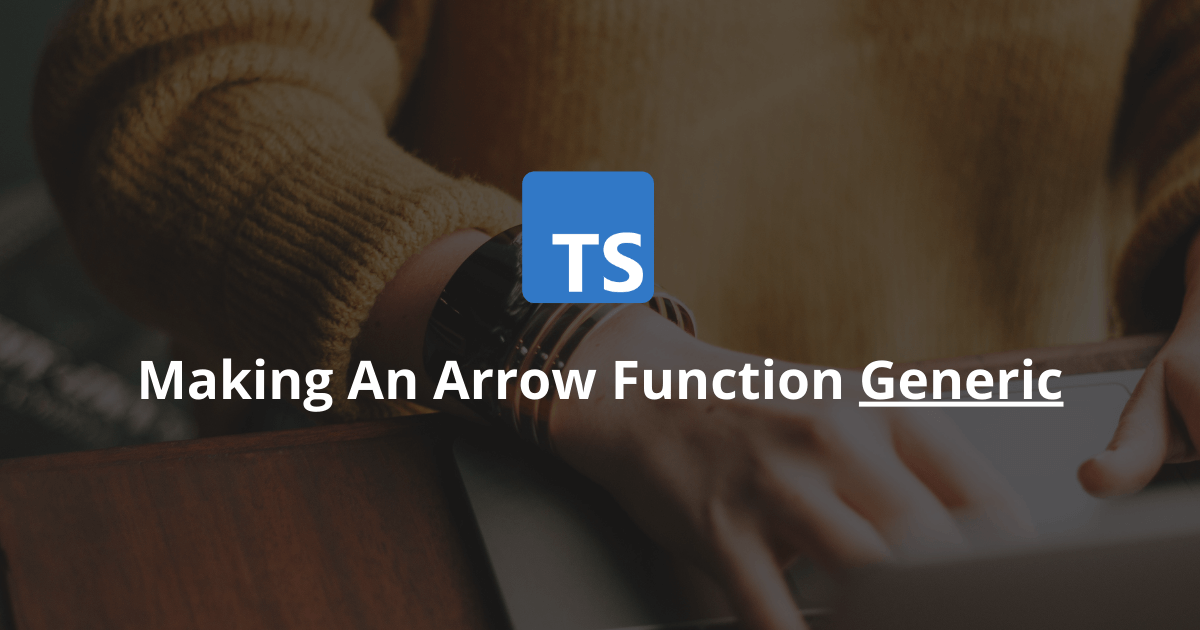
In TypeScript, generics allow developers to make a function, class, or type work with any data type (without restricting it to one type). In short, it makes it simple to write reusable code.
Making an arrow function generic
To make an arrow function generic, you must pass a generic argument before the function parameters.
Let's see this with an example.
We have a small function called getValue that accepts a number and outputs it:
typescriptconst output = (value: number): void => {
console.log(value);
};
// Outputs: 2
output(2);
This function only accepts numbers, but what if we want it to accept other types? We need to make it generic.
Here is how:
typescriptconst output = <T,>(value: T): void => {
console.log(value);
};
// Outputs: 2
output<number>(2);
// Outputs: 'value'
output<string>('value');
The getValue function is now generic and accepts any argument type you pass.
If you want to limit the type of argument this function accepts, you need to add a generic constraint.
For example:
typescriptinterface IAnimal {
type: string;
eat: () => void;
}
class Dog implements IAnimal {
type = 'dog';
eat(): void {
console.log('eating')
}
}
const output = <T extends IAnimal>(value: T): void => {
console.log(value);
};
// Outputs: Dog { type: 'dog' }
output(new Dog());
This function now only accepts an argument that implements the IAnimal interface.
Making an arrow function generic in a .tsx file
To make a generic TypeScript function work in .tsx files, you can add a comma after the last generic parameter.
Here is an example:
typescriptconst output = <T,>(value: T): void => {
console.log(value);
};
Or, you can add a generic constraint, for example:
typescriptconst output = <T extends unknown>(value: T): void => {
console.log(value);
};
Both solutions work, but the first one is clearer.
Final thoughts
As you can see, making an arrow function generic is simple in TypeScript.
Simply add the generic parameters before the function parameters and use those generic types in the function definition.
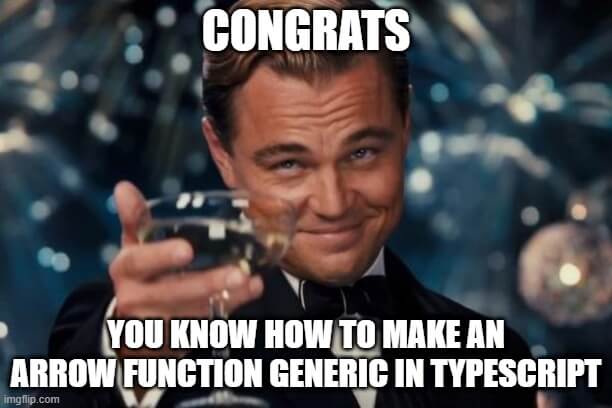
Here are some other TypeScript tutorials for you to enjoy: