How Does The Declare Keyword Work In TypeScript?
If you are writing TypeScript code long enough, you have seen the declare keyword. But what does it do, and why use it?
The declare keyword tells the TypeScript compiler that an object exists AND can be used inside your code.
This article explains the declare keyword and shows different use cases with code examples.
Let's get to it 😎.
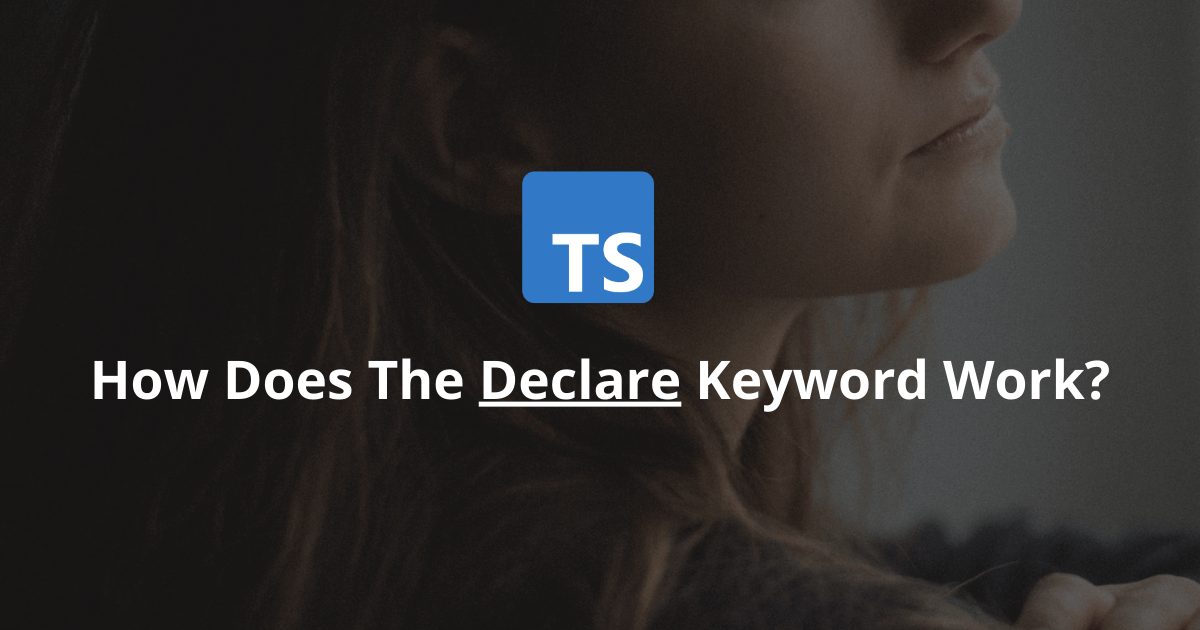
- The definition
- Concrete example
- Using the declare keyword to declare resources
- How to declare a global variable?
- How to declare a global function?
- How to declare a class?
- How to organize types and objects?
- Using the declare keyword to augment a module
- Using the declare keyword to do global augmentation
- Final thoughts
The definition
In TypeScript, the declare keyword tells the compiler that an object exists (and can be referenced inside your code). It declares the object to the TypeScript compiler. In short, it allows a developer to use an object declared elsewhere.
The compiler doesn't compile this statement into JavaScript.
A developer might need to use the declare keyword:
- To use a global variable declared in another file.
- To use a function, variable, or class generated by another file.
- Etc.
A lot of times, the declare keyword is used in TypeScript declaration files (.d.ts).
Using the declare keyword, you can declare:
- A variable (const, let, var).
- A type or an interface
- A class
- An enum
- A function
- A module
- A namespace
When declaring functions or classes, you only declare their structure, not their implementation.
Concrete example
You want to use the Google Analytics script in your TypeScript code. To do that, you must include it on your HTML page.
You may include it like that:
xml<script async src="https://www.googletagmanager.com/gtag/js?id=TAG_ID"></script>
<script>
window.dataLayer = window.dataLayer || [];
function gtag(){dataLayer.push(arguments)};
gtag('js', new Date());
gtag('config', 'TAG_ID');
</script>
The dataLayer array is declared in your HTML. The TypeScript compiler isn't aware of it, so if you want to use it, you need to declare it.
Here is how you declare it:
typescriptdeclare const dataLayer: any[];
You can now use the dataLayer variable in your TypeScript code.
Using the declare keyword to declare resources
Often, TypeScript code needs to import resources, for example, images or scalable vector graphics (SVG).
In those cases, you must create a declaration for each module.
For example, if we want to use PNGs in our code, we can create a declaration like that:
typescriptdeclare module '*.png' {
const src: string;
export default src;
}
In this case, we use the wildcard module declaration, so we don't have to declare each image path individually.
How to declare a global variable?
Enter the variable name and type in a declaration file to declare a global variable.
Here is an example:
typescriptdeclare var CPT: number;
We can now use the CPT variable in our code.
How to declare a global function?
Write the function definition inside a declaration file to declare a global function.
Here is an example:
typescriptdeclare function sayHello(hello: string): void;
We can now use the sayHello function in our code.
How to declare a class?
When declaring a class, you only write the class structure without each function's implementation.
Here is an example:
typescriptdeclare class Animal {
constructor(name: string);
eat(): void;
sleep(): void;
}
We can now instantiate the Animal class in our code.
How to organize types and objects?
To organize multiple types and objects, you can declare one of the following concepts:
- A namespace
- A module
Inside them, declare all your types, objects, classes, etc.
Here is an example of a namespace declaration:
typescriptdeclare namespace AnimalLib {
class Animal {
constructor(name: string);
eat(): void;
sleep(): void;
}
type Animals = 'Fish' | 'Dog';
}
Here is an example of a module declaration:
typescriptdeclare module AnimalLib {
class Animal {
constructor(name: string);
eat(): void;
sleep(): void;
}
type Animals = 'Fish' | 'Dog';
}
Using the declare keyword to augment a module
A developer can also use the declare keyword to augment a module.
For example, we can add a new property to an existing interface contained inside a module.
Here is an example of this:
typescriptimport {
Palette as MuiPallete
} from '@mui/material/styles/createPalette';
declare module '@mui/material/styles/createPalette' {
interface Palette extends MuiPallete {
custom: {
prop1: string;
}
}
}
Here, we augment the Palette interface from the Material UI styles module and add the custom object.
Read more: Extend an interface in TypeScript
Using the declare keyword to do global augmentation
A developer can also use the declare keyword to add declarations to the global scope.
For example, here is how you can add a new method to the String JavaScript object:
typescriptdeclare global {
interface String {
toSmallString(): string;
}
}
String.prototype.toSmallString = (): string => {
// implementation.
return '';
};
In this example, we add a new function called toSmallString to the String prototype.
Final thoughts
As you can see, the declare keyword is useful when using variables created elsewhere.
Even though this concept is not well known, it is essential to understand it well to be a well-rounded TypeScript programmer and to be able to help your peers.
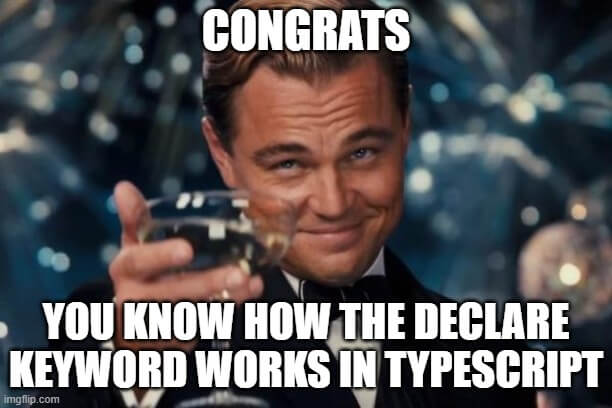
Here are some other TypeScript tutorials for you to enjoy:
- The definition
- Concrete example
- Using the declare keyword to declare resources
- How to declare a global variable?
- How to declare a global function?
- How to declare a class?
- How to organize types and objects?
- Using the declare keyword to augment a module
- Using the declare keyword to do global augmentation
- Final thoughts