How Does An Arrow Function Work In TypeScript?
Nowadays, an arrow function is the default and easiest way to define a function in TypeScript and JavaScript. But how does it work exactly?
Here is an example of an arrow function in TypeScript:
typescriptconst sum = (x: number, y: number): number => x + y;
This article explains how arrow functions work in TypeScript and shows many code examples.
Let's get to it 😎.
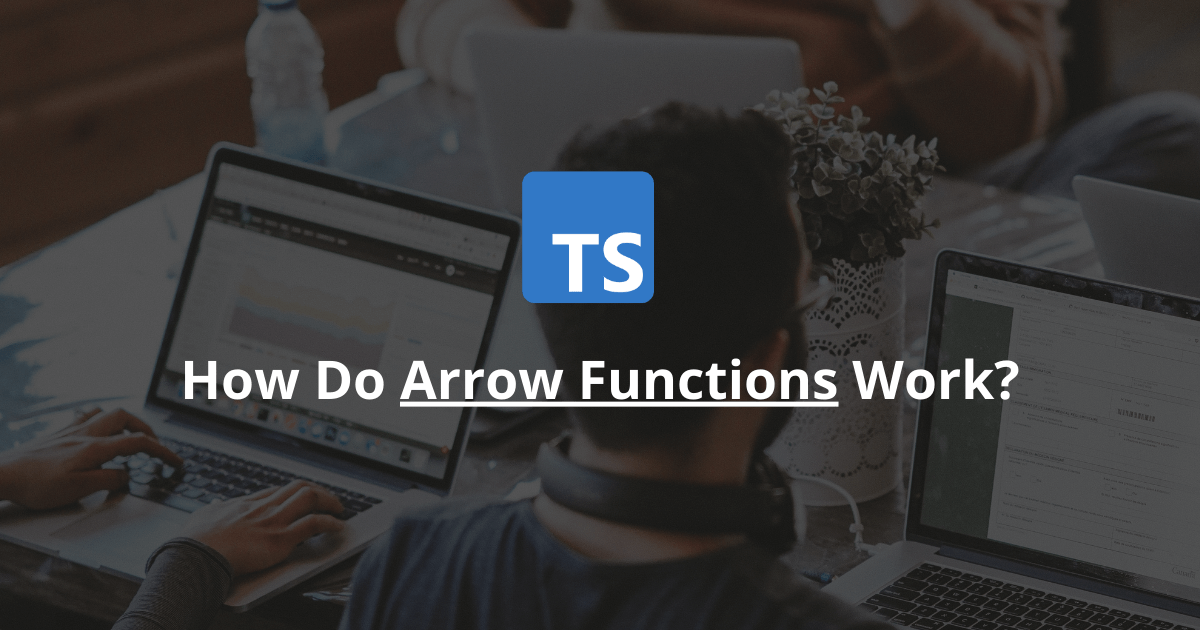
The definition
Initially introduced in ES6, arrow functions allow developers to write functions with shorter syntax.
They are sometimes called lambda, fat arrow, or anonymous functions.
We do not need to use the function keyword when defining an arrow function.
They are many reasons to use the arrow function.
Here are some of them:
- Shorter and more straightforward syntax.
- Lexical scoping of this keyword.
- Lexical capturing of arguments.
You can use default parameters with arrow functions if you need to set default values for the function's parameters.
In TypeScript, arrow functions can also be generic. A generic arrow function can accept any argument type and allows developers to create more reusable components.
Arrow functions also allow developers to use the quick object return.
The syntax
Here is the syntax of an arrow function:
typescript(param1, [ ...paramN ]) => expression
The syntax of the arrow function contains three parts:
- The parameters that the function may or may not have.
- The arrow notation.
- The expression which represents the function's body.
Difference between an arrow function vs. a regular function
Both are valid ways to define functions in TypeScript and JavaScript. However, they have significant differences.
The most obvious difference is their notation:
typescript// Function expression
const output = function (str: string): void {
console.log(str);
}
// Arrow function
const output2 = (str: string): void => {
console.log(str);
}
The most significant difference between an arrow and a regular function is their execution context (this).
- Inside a regular function, the this value is dynamic. It means that its value depends on how the function is invoked.
- Inside an arrow method, the this value always equals the outer function, making it easier to understand.
Other differences include:
- Arrow methods cannot be overridden inside a child class, but regular functions can.
- Arrow functions cannot be called using the new keyword, but regular functions can.
- Arrow functions provide support for implicit return, and regular functions don't.
How to return an object from an arrow function?
Arrow functions allow returning an object literal without using the return keyword. To do a quick object return, surround the object literal with parentheses.
Here is an example:
typescriptinterface IAnimal {
name: string;
type: string;
}
const getAnimal = (name: string, type: string): IAnimal => ({
name,
type
});
P.S. Quick object return only works when the arrow function only contains a return statement.
How to set the return type of an arrow function?
The return type of an arrow function is set the same way as for a regular function. The developer needs to define the return type explicitly or let the TypeScript compiler infer the return type.
Here is an example:
typescriptinterface IPerson {
name: string;
age: number;
}
const getPerson = (name: string, age: number): IPerson => ({
name,
age
});
In this example, the getPerson function returns the IPerson interface.
How to define an arrow function in a class?
In TypeScript, you can define an arrow function inside a class as a property.
Here is an example:
typescriptclass Dog {
public constructor(
private name: string
) { }
eat = (): void => {
console.log(`${this.name} is eating.`);
}
}
// Outputs: 'Flappy is eating.'
new Dog('Flappy').eat();
In this example, we define the eat function as a class property.
An arrow method inside a class is useful when using the function as a callback. Property methods pass the correct context to the function. Otherwise, you need to bind this to the function.
Note: Here we are using string interpolation to concat the strings.
Final thoughts
As you can see, arrow functions are simple to use in TypeScript.
Most of the time, I recommend using arrow functions unless:
- You are using a library like jQuery that uses the dynamic context of this.
- You want to use arguments.
- You want to use object methods.
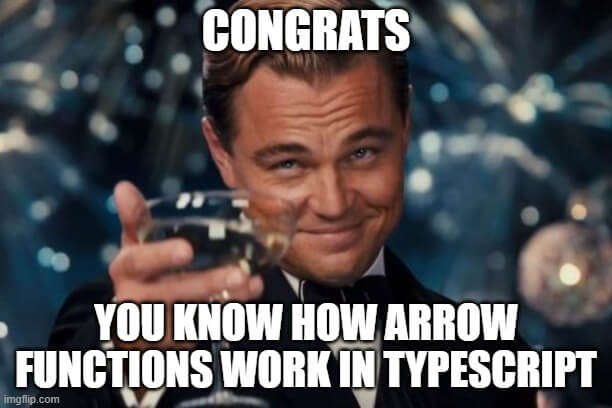
Here are some other TypeScript tutorials for you to enjoy: