How To Use The Spread Operator In TypeScript?
You may have seen a mysterious syntax of three dots when you open a TypeScript file in your day-to-day coding life. This syntax is called the spread operator.
The spread operator allows to spread or expand iterable objects into individual elements.
This article explains the spread operator in TypeScript and shows many code examples.
Let's get to it 😎.
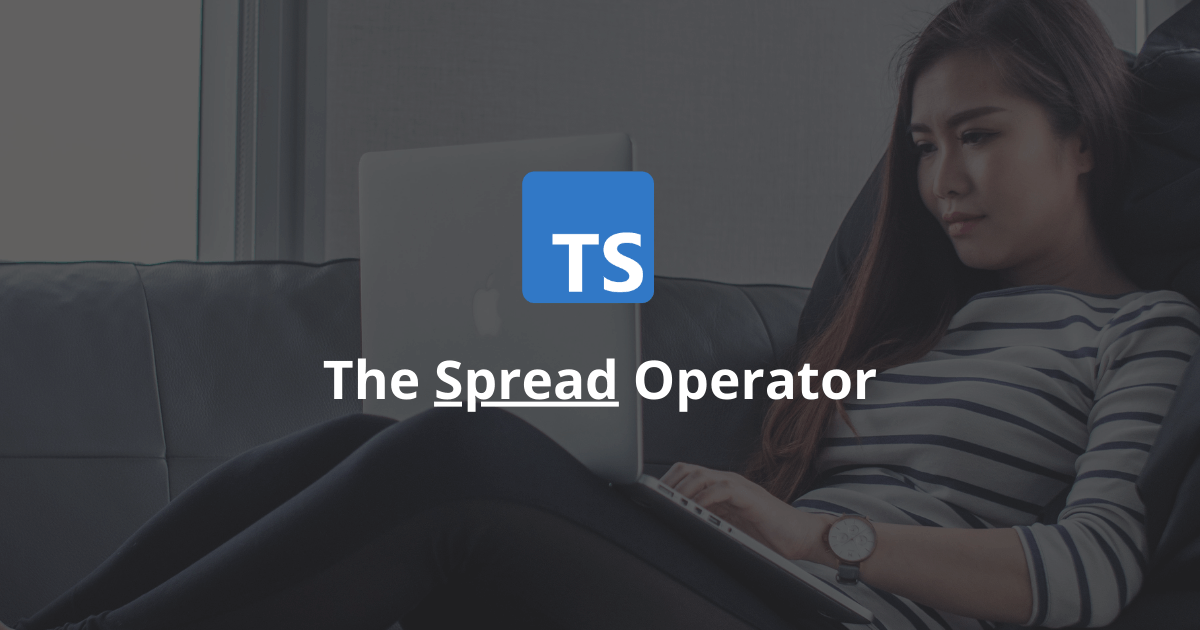
The definition
The spread operator, added with ES6, allows to spread or expand iterable objects into individual elements. The syntax for this operator is three dots (...), also called an ellipsis.
Using the spread operator, a developer can:
- Copy an array/object (shallow copy).
- Merge an array/object.
- Pass an array to a function call as individual parameters.
⚠️ The spread operator is not the same as the rest operator, even though they both use the same syntax of three dots (...). The rest operator is the inverse of the spread operator. Instead of expanding iterable objects into individual elements, it compresses them into one.
Using the spread operator with an array
A developer can use the spread operator to copy an array in TypeScript.
Here is an example:
typescriptconst numbers = [25,49,29];
const copy = [...numbers];
// Outputs: [25, 49, 29]
console.log(copy);
As you can see, copy now contains all the elements from the array called numbers.
⚠️ However, be careful because the spread operator only does a shallow copy. It works fine if you copy primitives, but if you want to copy objects, I advise using a library that can deep-clone.
Also, using the spread operator, you can merge two arrays.
Here is an example:
typescriptconst numbers = [25,49,29];
const numbers2 = [44,19,39];
const merged = [ ...numbers, ...numbers2 ];
// Outputs: [25, 49, 29, 44, 19, 39]
console.log(merged);
This method works well as an alternative to the Array.concat function or the Lodash merge function.
Using the spread operator with an object
Also, you can use the spread operator to define a new object from an existing object.
Here is an example:
typescriptconst obj1 = { a: 1, b: 2 };
const copiedObj = { ...obj1 };
// Outputs:{ "a": 1, "b": 2 }
console.log(copiedObj);
In this example, we define a new object called copiedObject from the existing object called obj1.
You can also merge multiple objects with the spread operator:
typescriptconst obj1 = { a: 1, b: 2 };
const obj2 = { c: 3, d: 4 };
const mergedObj = { ...obj1, ...obj2 };
// Outputs:{ "a": 1, "b": 2, "c": 3, "d": 4 }
console.log(mergedObj);
⚠️ Remember, the spread operator only does a shallow copy, so if you try to clone objects, it will cause bugs.
Using the spread operator with a function call
You can also utilize the spread operator to pass an array as individual parameters in a function call.
Here is an example:
typescriptconst params = [ 2, 3, 4 ] as const;
const add = (a: number, b: number, c: number): number => {
return a + b + c;
}
const result = add(...params);
// Outputs: 9
console.log(result);
In this example, using the spread operator, the params array we pass to the add function spreads as individual parameters.
When spreading an array in a function call, you can get this error: "A spread argument must either have a tuple type or be passed to a rest parameter."
To fix this error, you need to:
- Define the array type as a tuple.
- Use const assertion on the array (like I did in the example).
Final thoughts
As you can see, the spread operator is great when you need to copy an array into a new array, merge two arrays, or copy object properties into a new object.
But remember that the spread operator only works well on primitive values because it does a shallow copy. If you want to clone objects, you can use a library like clone-deep to make it work.
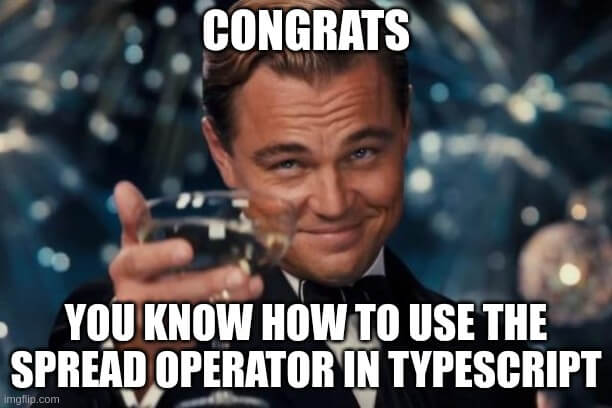
Here are some other TypeScript tutorials for you to enjoy: