How Does The Lodash Merge Function Work?
Lodash is the most used JavaScript library in the world. It provides a lot of useful utility functions (for example, the Lodash debounce function, the get function, etc...) that help developers simplify common tasks. One of those useful methods is the merge function.
The Lodash merge function merges two or more objects into one.
Here is an example of the merge function in action:
javascriptimport { merge } from 'lodash';
const obj1 = { startYear: 2000 };
const obj2 = { endYear: 2022 };
const merged = merge(obj1, obj2);
// Outputs: '{ endYear: 2022, startYear: 2000 }'
console.log(merged);
In this article, I will explain everything to know about the Lodash merge function, how and when to use it, as well as answer some of the most common questions.
Let’s get to it 😎.
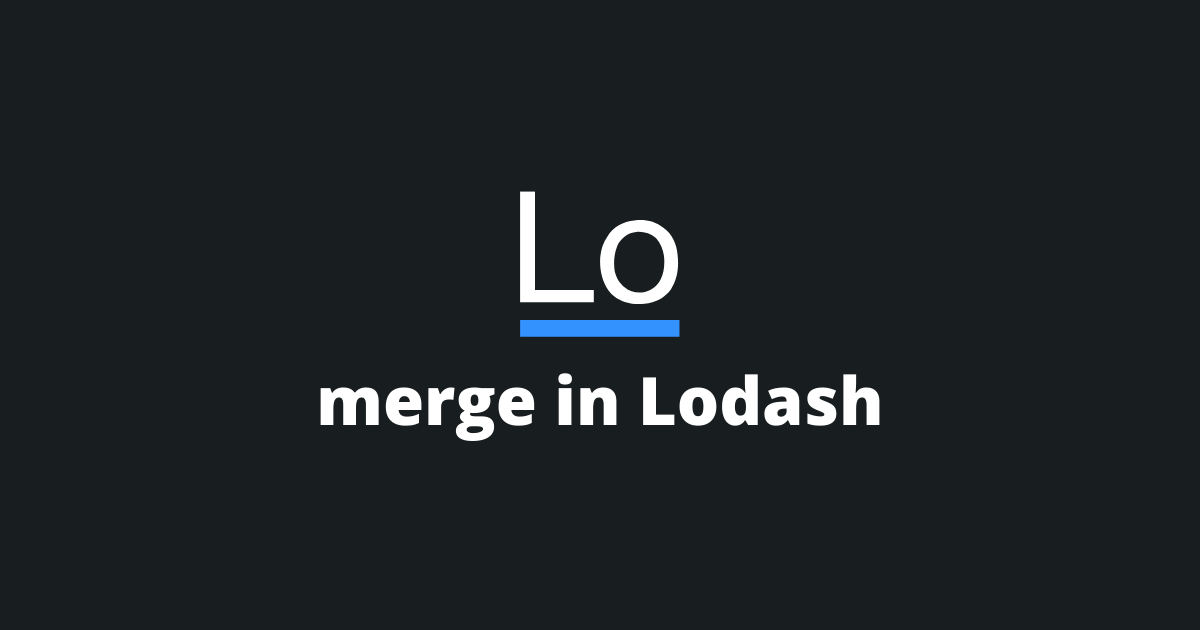
The definition
The merge function creates a merged object from two or more objects.
The syntax
javascript_.merge(object, [sources])
This function accepts two or more parameters.
- The first argument (object) holds the destination object.
- The second and subsequent arguments hold the source objects.
If two or more keys are the same, the generated object will take the value from the rightmost argument.
How to import the merge function?
When it comes to the bundle size, Lodash is a very big package. That's why, I recommend not using the default import, but rather importing the functions that you need individually. There are two ways of importing Lodash functions individually.
The first way is to use the curly braces:
javascriptimport { merge } from 'lodash';
The second way is to use the one-by-one method:
javascriptimport merge from 'lodash/merge';
The one-by-one import will result in the smallest bundle size.
How to use this function?
Here is a small example for you of how to use this function.
javascriptimport { merge } from 'lodash';
const obj1 = { a: 'a', b: 'b' };
const obj2 = { c: 'c', d: 'd' };
const obj3 = { a: 'z', f: 'f' };
// Outputs: '{ a: "a", b: "b", c: "c", d: "d" }
console.log(merge(obj1, obj2));
// Outputs: '{ a: "z", c: "c", d: "d", f: "f" }'
console.log(merge(obj2, obj3));
// Outputs: '{ a: "z", b: "b", c: "c", d: "d", f: "f" }'
console.log(merge(obj1, obj2, obj3));
Differences between merge and assign in Lodash?
As you can see, the merge function is very similar to the assign function, however, they still have some differences between them.
The biggest difference between them is:
- the assign function uses shallow copy.
- the merge function uses deep copy.
A shallow copy creates a newly copied object with references to its original object.
A deep copy creates a newly copied object without references to its original object which makes the copied object truly independent.
There are also smaller differences between them:
- The merge function will copy inherited properties from a class, but the assign function will not.
- The merge function will not override values equal to undefined, but the assign function will.
When to use the merge function?
Use the Lodash merge function if you want the final generated object created from deep copies. Use it to deep merge two or more objects.
Are there alternatives to the merge function?
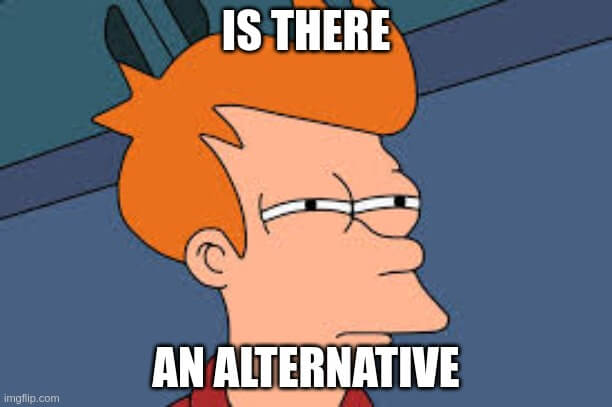
If you do not need the deep copy feature you can use:
- The Object.assign function.
- The ES6 spread operator.
- The Lodash assign function.
However, all of those functions are using shallow copies.
Final thoughts
As you can see the Lodash merge function is very straight and forward and easy to understand.
For my part, I use this function when I need to deep merge two or more objects.
If you are interested in learning more, I have written more tutorials on Lodash on this website.
Don’t hesitate to ask your questions about Lodash in the comments section. It will be a pleasure for me to answer them.
Thank you very much for reading this article.
Please share it with your fellow developers and colleagues.