How Does The Debounce Function Work in Lodash?
Lodash is a very popular JavaScript utility library that helps developers simplify common tasks. It provides a lot of different utility functions (for example, the Lodash find function, the merge function, and many more) that a developer can import into its JavaScript project. One of its most useful functions is the debounce function.
The debounce function creates a delayed function, that executes a function after X milliseconds pass from its last execution.
Here is an example of the debounce function in action:
typescriptimport { debounce } from 'lodash';
const debounced = debounce(() => {
console.log('delayed');
}, 500);
debounced();
// Output after 500ms: 'delayed'
In this article, I will go over, in detail, the debounce function in Lodash, the arguments that it accepts, as well as explain when and how to use it.
Let’s get to it 😎.
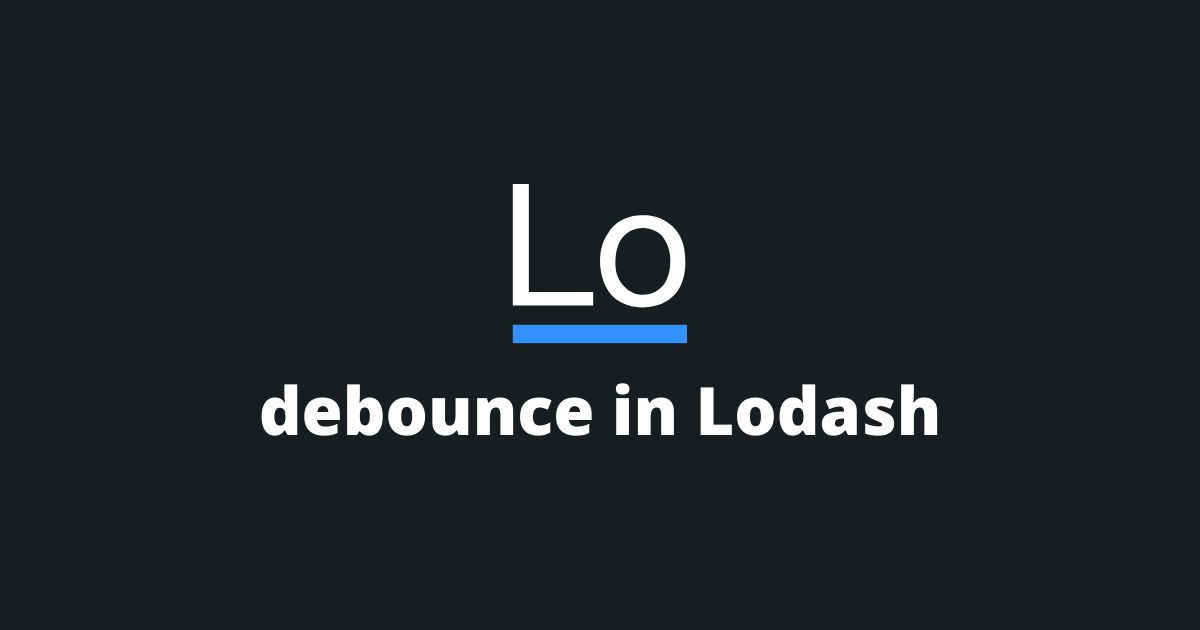
How to install Lodash (with npm/yarn)?
Lodash is a modern utility library that helps developers work with arrays, strings, numbers, and many more.
To install Lodash in your project, you will need to use a package manager, either yarn or npm.
Open a terminal from your project's directory location and type:
bash> npm i -g npm
> npm i --save lodash
Or, add Lodash with yarn:
bash> npm i -g yarn
> yarn add lodash
Now, you are ready and can use the debounce function.
The debounce function
The Lodash debounce function returns a debounced function that when called will execute a function after X milliseconds pass since its last execution.
The syntax
typescript_.debounce(func, [wait=0], [options={}])
Here is an example of the debounce function in action:
typescriptconst logMyName = _.debounce(() => {
console.log('My name is Tim.');
}, 1000)
logMyName();
logMyName();
// Output after 1s: 'My name is Tim.'
As you can see even though we are calling the function two times it only executes one time because the waiting period hasn't elapsed. This function will only be able to run after 1000 milliseconds pass.
Debounce arguments
As you may have noticed by looking at its syntax, the debounce function also accepts different options. Those options are optional.
Here is a table with all the options that you can use:
Data type | Default value | Description | |
---|---|---|---|
leading | boolean | false | Whether to invoke the function right away. |
maxWait | number | - | The maximum number of milliseconds the function can be delayed before being executed. |
trailing | boolean | true | Whether to invoke the function after the delay. |
When to use a debounced function?
Most of the time a debounced function is used to improve browser performance.
For example:
- when you want to wait for the user to finish typing.
- when you want to wait for the user to finish resizing the screen.
- when you want to wait for the user to finish scrolling.
How does debounce work in React?
One of the good things about Lodash is that you can use it with any JavaScript framework.
Here is an example of how to debounce an input in React.
typescriptimport React from 'react';
import ReactDOM from "react-dom";
import { debounce } from 'lodash';
const Input = () => {
const logValue = debounce((value) => {
console.log(value)
}, 1000);
const onChange = (e) => {
logValue(e.target.value);
}
return <input type="text" onChange={onChange} />;
};
ReactDOM.render(<Input />, document.getElementById("container"));
Final thoughts
As you can see, the debounce function is a very simple yet handy function, that you will almost certainly need. You could implement it yourself, but you will end up with different implementations or copy-pasting issues across all of your projects. That's why I recommend using the one provided by Lodash.
Lodash is quite a large package, that's why my advice to you is to use tree-shaking and only import the functions that you need.
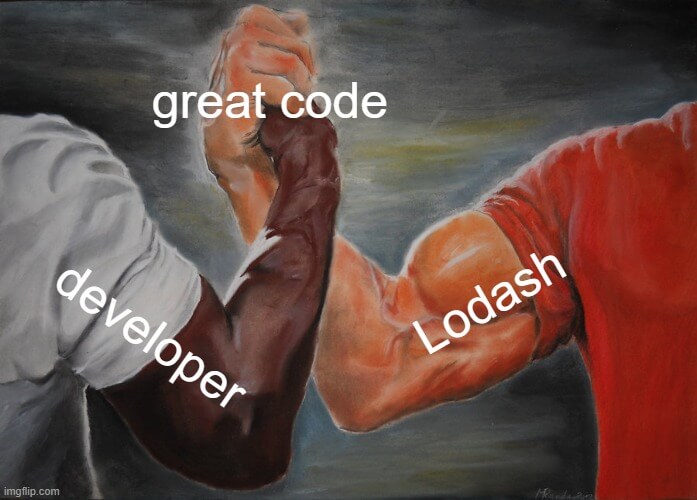
Don’t hesitate to ask your questions about Lodash in the comments section. I always respond 😉.
Please share this article with your fellow coders and friends, and thank you very much for reading it.