How Does The Lodash Find Function Work?
Lodash is a popular JavaScript utility library first launched in 2012. It provides a lot of useful functions (for example, the Lodash debounce function, the merge function, and many more) for common tasks that a developer might encounter. One of those helpful methods is the find function.
The Lodash find function returns the first element in a collection that matches a specific condition.
Here is an example of the find function in action:
javascriptimport { find } from "lodash";
const collection = [1, 4, 5, 7, 10, 12, 20];
const match = find(collection, (n) => {
return n > 10;
});
// Outputs: 12
console.log(match);
In this article, I will go over, in detail, everything to know about this function, as well as show real-life examples of how to use this function.
Let’s get to it 😎.
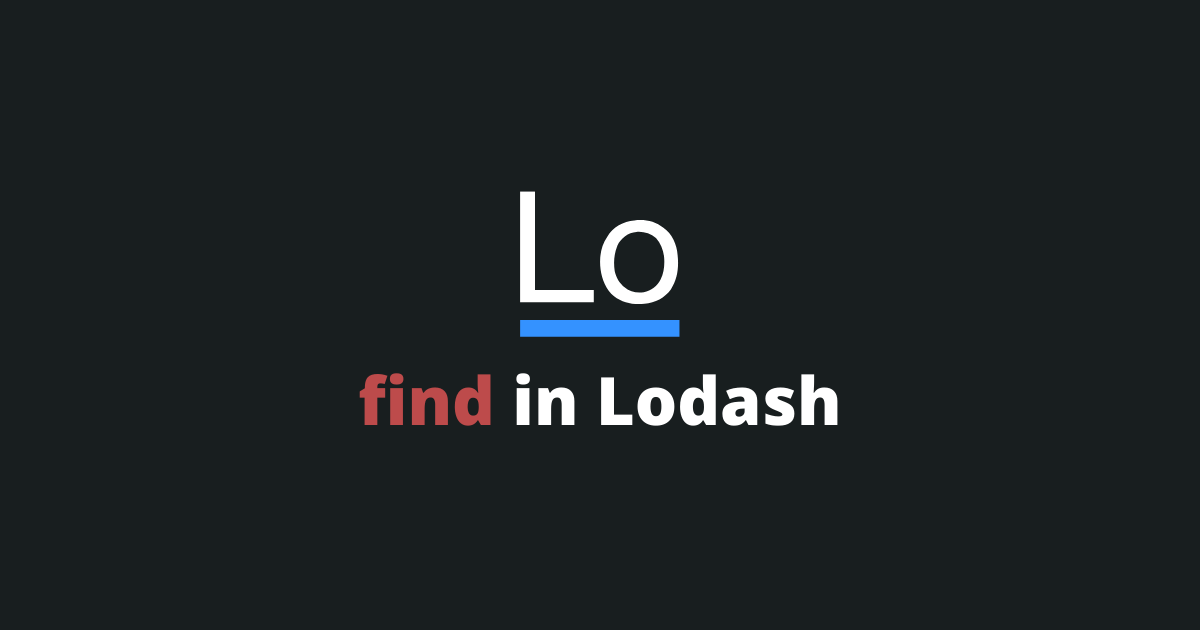
The definition
The Lodash find function iterates over a collection and returns the first element that matches a condition.
The syntax
javascript_.find(collection, [condition], [fromIndex=0])
If no result is found the function will return undefined.
The fromIndex parameter is optional and holds the index from which to start the iteration. The default fromIndex value is zero.
How to import the find function?
Unfortunately, Lodash is quite a big package and takes up a lot of space in the application bundle. That's why I recommend importing the functions that you need individually.
Here is how to import a function using curly braces:
javascriptimport { find } from 'lodash';
Here is another way of importing using the one-by-one method:
javascriptimport find from 'lodash/find';
The latter will result in the smallest bundle size.
How to use the find function?
Here are a few examples of how to use this function.
javascriptimport { find } from "lodash";
const collection = [1, 4, 5, 7, 10, 12, 20];
const collection2 = [
{ name: "Tim" },
{ name: "Mark" },
{ name: "Bob" }
];
const match = find(collection, (n) => n > 5);
// Outputs: 7
console.log(match);
const match2 = find(collection, (n) => n > 100);
// Outputs: undefined
console.log(match2);
const match3 = find(collection2, (n) => n.name[0] === "M");
// Outputs: {name: "Mark"}
console.log(match3);
How to find an object in an array by property?
You can use the find function to find an object by a specific property inside an array.
Here is an example of how to do it:
javascriptimport { find } from "lodash";
const collection = [
{ name: "Tim", age: 26 },
{ name: "Mark", age: 39 },
{ name: "Bob", age: 27 }
];
const match = find(collection, { name: "Tim" });
// Outputs: { name: "Tim", age: 26 }
console.log(match);
How to find an object in a nested object?
To find an object in a nested object using Lodash you can use the filter function.
Here is an example of how to do it:
javascriptimport { filter } from "lodash";
const persons = [
{
name: "Tim",
articles: [
{
key: "lodash-get"
},
{
key: "lodash-debounce"
}
]
},
{
name: "Mark",
articles: [
{
key: "finances"
}
]
}
];
const match = filter(persons, {
articles: [{ key: "finances" }]
});
// Outputs: [{ name: "Mark", articles: [{key: "finances"}] }]
console.log(match[0]);
Differences between find and filter in Lodash?
Both functions are very similar but have a few differences non the less.
The biggest difference between the find vs filter methods is:
- The find function returns one single item that matches a condition.
- The filter function finds all the items that match a condition in a collection. It also returns the result in an array.
Final thoughts
As you can see the find function does exactly what its name sounds as.
It finds the first element in a collection that matches a certain condition.
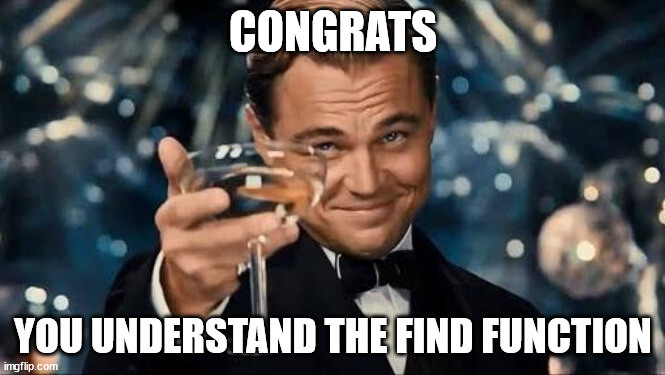
For my part, I do not use this function, I prefer to use the built-in JavaScript find function. However, it requires a polyfill if you plan on supporting Internet Explorer.
I have written more tutorials on Lodash on this website (if you are interested in learning more).
Don’t hesitate to ask your questions about Lodash in the comments section. I always check for new questions and respond to them.
Thank you very much for reading this article.
Please share it with your colleagues and fellow coders.