How Does The Get Function Work in Lodash?
Being one of the most popular utility libraries for JavaScript, it is important for a developer to understand Lodash well. This library gives you access to a lot of very useful methods (for example, the Lodash debounce function, the merge function, etc…) that provide solutions for common tasks. One of those methods is the get function.
The Lodash get function returns the value at the path of an object.
Here is an example of the get function in action:
typescriptimport { get } from 'lodash';
const obj = { 'b': { 'c': [ { 'name': 'Tim' } ] } };
const value = get(obj, 'b.c[0].name');
console.log(value);
// Outputs: 'Tim'
In this article I will explain, in detail, the Lodash get function, how and when to use it, as well as show examples.
Let’s get to it 😎.
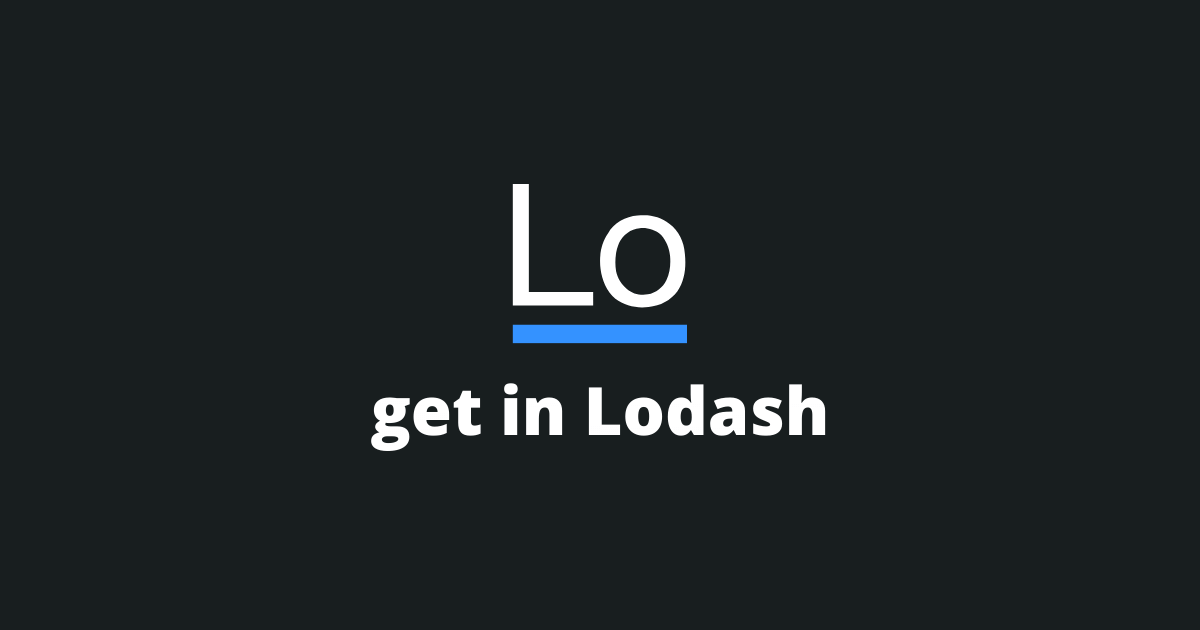
The definition
The get function returns the value at a path of an object.
Syntax
typescript_.get(object, path, [defaultValue])
This function is very useful when you need to return deeply nested values.
Optionally, you can specify a default value, that the function will return if it is unable to resolve the specified path.
How to import the get function?
Lodash is a very big package in size, that's why I recommend using a bundler like Webpack that has tree-shaking enabled. Also, I recommend not using the default import, but rather importing only the methods that you are using.
Here is an example of how to import this function:
typescriptimport { get } from 'lodash';
or like this:
typescriptimport get from 'lodash/get';
The latter will give you the smallest bundle size.
How to use this function?
Here is a small example for you of how to use this function.
typescriptimport { get } from 'lodash';
const configuration = {
general: {
google: {
maps: 'Maps'
},
imgs: [
{
logo: 'Logo'
}
]
}
};
// Outputs: 'Maps'
console.log(get(configuration, 'general.google.maps'));
// Outputs: 'Logo'
console.log(get(configuration, 'general.imgs[0].logo'));
// Outputs: 'ABC'
console.log(get(configuration, 'general.imgs[0].logo123', 'ABC'));
In the last output, as you can see, the function returns the default value, since the path doesn't resolve.
Why use the get function?
The get function simplifies the return of deeply nested values. It also lets you not worry about null and undefined properties.
Is there an alternative to this function?
Yes. If your browser supports it, you can use optional chaining. Optional chaining is a new JavaScript feature that lets you safely access deeply nested properties.
Here is an example of this:
typescriptconst obj = { 'b': { 'c': [ { 'name': 'Tim' } ] } };
// Outputs: 'Tim'
console.log(obj.b?.c?.[0]?.name);
If you are using an older browser that doesn't support optional chaining, you can use it in combination with Babel.
Babel is a toolchain that lets your convert your new JavaScript ES2015+ code into old ES5 JavaScript. It also can add polyfills and has many more features.
You can use Babel with Webpack.
Final thoughts
Here you go…
Now you understand the get function and will be able to use it in your code.
For my part, I use the get function when working with deeply nested objects that have a lot of layers. In those cases, it is easier to use this function rather than using loops and other tricks.
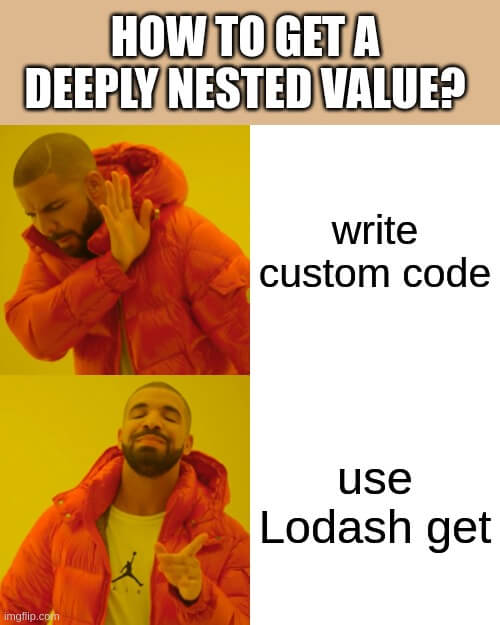
I have written more tutorials on Lodash on this website (if you are interested in learning).
Don’t hesitate to ask your questions about Lodash in the comments section.
Thank you very much for reading this article, please share it with your fellow coders and friends.