How To Use Named Parameters In TypeScript?
Named parameters exist in multiple languages such as C# or PHP, and developers from those languages may expect that TypeScript also provides this feature. Unfortunately, this language does not provide true named parameters. However, it is still possible to achieve a similar result using destructuring.
To use named parameters in TypeScript, you can use destructuring like so:
typescriptinterface IArticle {
title: string;
content: string;
}
const logArticle = ({ title, content }: IArticle): void => {
console.log(title, content)
}
// Outputs: 'my article', 'my content'
logArticle({ title: 'my article', content: 'my content' });
This article will go through everything about using named parameters in TypeScript functions and answer some of the most common questions.
Let's get to it 😎.
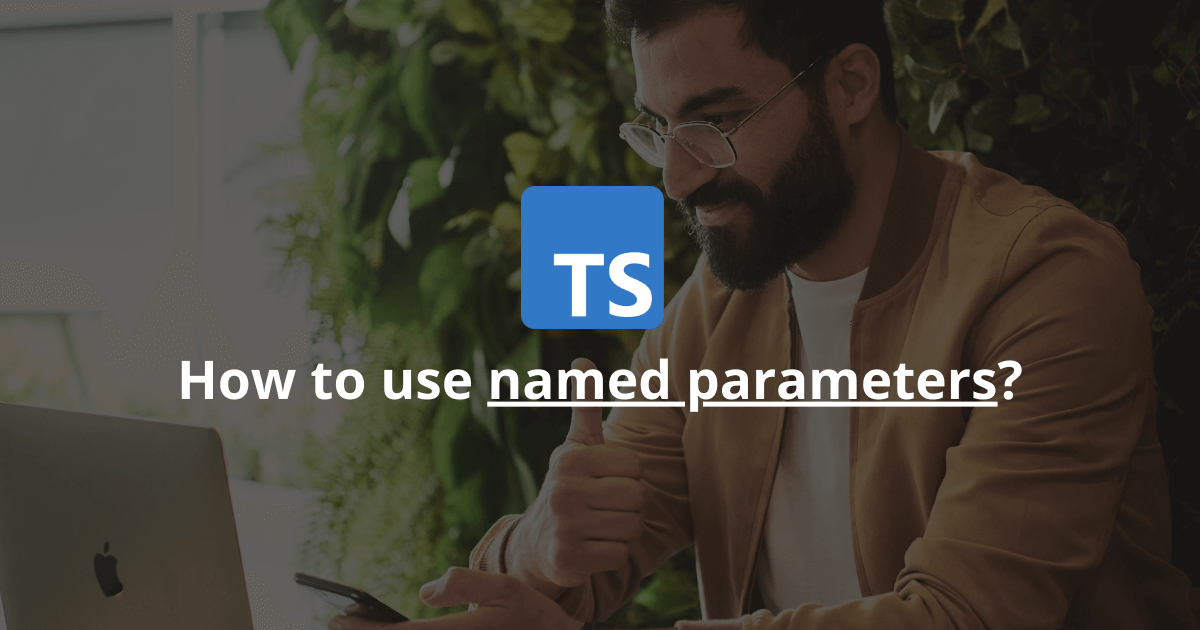
What are named parameters?
Named parameters refer to a feature in some languages to specify the arguments within a function call by name rather than position.
Some languages that support named parameters include PHP, C#, Python, and many others.
Named parameters are used to:
- Increase readability
- Make the code easier to refactor
How to use named parameters with destructuring?
Unfortunately, JavaScript and TypeScript do not support named parameters.
However, we can simulate this feature with a single object parameter and destructuring.
Here is how you can do it:
1. You declare an interface or type object that contains all the accepted arguments of a function:
typescriptinterface IAnimal {
name: string;
age: number;
}
2. You declare the function and use object destructuring on the parameters:
typescriptconst logAnimal = ({ name, age }: IAnimal): void => {
console.log(name, age)
}
3. Finally, you call the function and specify each argument by name:
typescriptlogAnimal({ name: 'Jacky', age: 10 });
And here is a combination of everything:
typescriptinterface IAnimal {
name: string;
age: number;
}
const logAnimal = ({ name, age }: IAnimal): void => {
console.log(name, age)
}
// Outputs: 'Jacky', 10
logAnimal({ name: 'Jacky', age: 10 });
Read more: How do interfaces work in TypeScript?
How to set up a default parameter for a named parameter?
In JavaScript, it is easy to define a default parameter for a named parameter.
Here is an example of how you can do it:
typescriptinterface IAnimal {
name: string;
type?: string;
}
const logAnimal = ({ name, type = 'dog' }: IAnimal): void => {
console.log(name, type)
}
// Outputs: 'Jacky', 'dog'
logAnimal({ name: 'Jacky' });
We first need to set the type property as an optional parameter.
This way, we don't have to pass it when we call the function.
Then, we set up a default value for the type property in the function definition.
Final thoughts
As you can see, true named parameters don't exist in TypeScript.
However, you can achieve the same result in your code using destructuring.
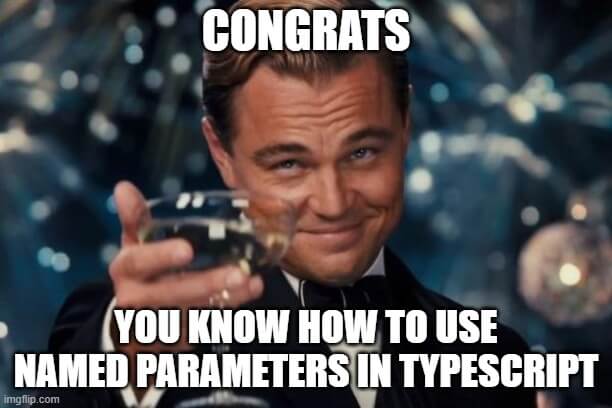
Here are some other TypeScript tutorials for you to enjoy: