How does the for loop work in TypeScript?
The for loop is one of the most common operations in programming.
The for loop is used to execute a specific code block a specified number of times. Generally, one of the most common use-cases of this loop is iterating over a dictionary (also called a map) or an array and execute a piece of code.
In this article, you will learn everything to know about this statement, how it works in TypeScript, and some common use-cases for it.
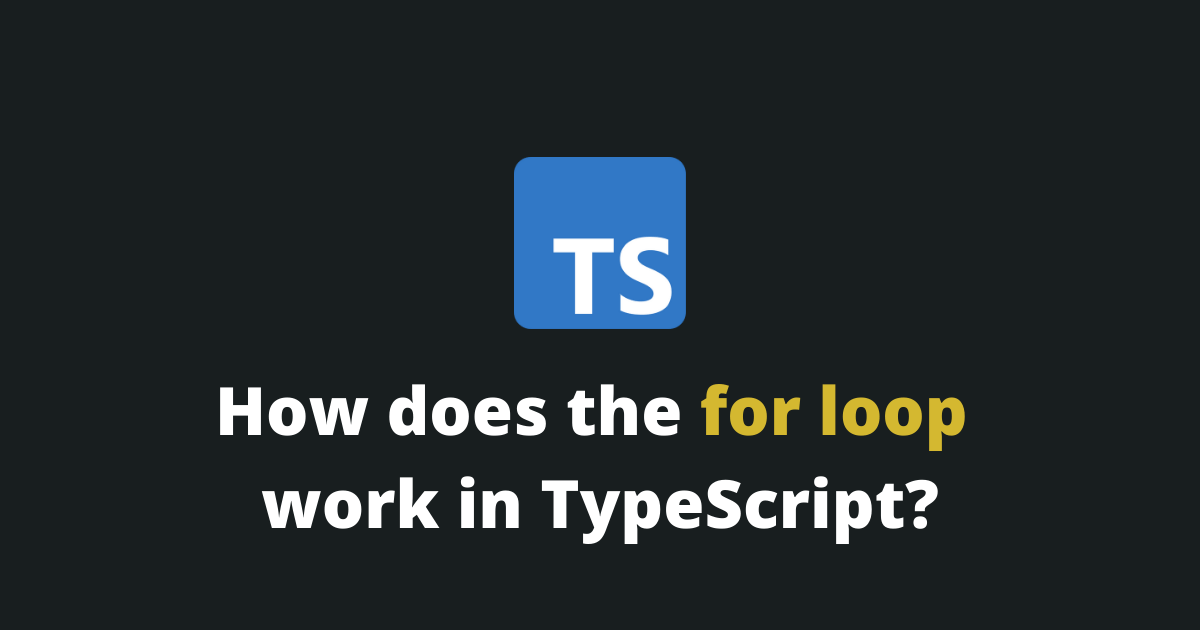
In TypeScript, just like in JavaScript, the for loop executes a code block a specified number of times.
The for statement is a control flow statement (just like the switch statement).
Here is an example of the for loop in action:
javascriptfor (let i = 0; i < 5; i++) {
console.log('Iteration ' + i);
}
Output:
javascript// Iteration 0
// Iteration 1
// Iteration 2
// Iteration 3
// Iteration 4
Syntax of the for loop
The default for loop has 3 statements.
javascriptfor (statement 1; statement 2; statement 3) {
// code to be executed.
}
Statement | Execution | Explanation |
---|---|---|
statement 1 | Only one time in the beginning. | The looping variable is declared here. |
statement 2 | Every time before the execution of the code block. | The condition based on which the for loop will execute a new iteration of the code. |
statement 3 | Every time after the execution of the code block. | The looping variable is updated here. |
Those are the steps when a for loop executes:
- Statement 1 executes. This is where we declare the looping variable.
- Statement 2 executes. If the condition is false the for loop exits.
- The code block executes.
- Statement 3 executes.
- Go back to step 2.
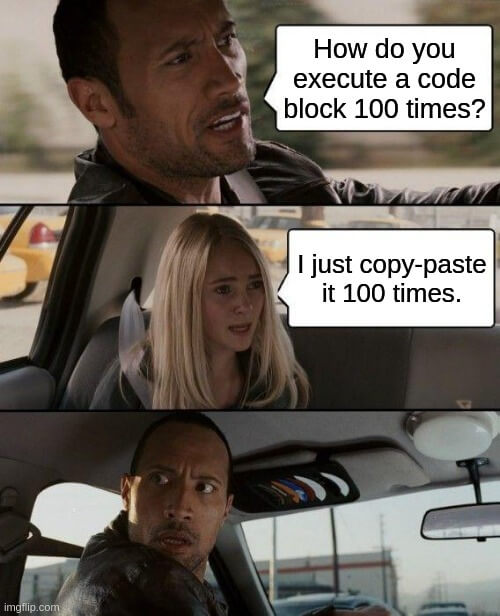
How to loop through an array with the for loop.
When you need to loop through an array with the for loop, you need to:
- Initialize the looping variable with 0.
- Ensure the looping variable is less than the array length (in the condition statement).
- Increment the looping variable by 1.
javascriptlet arr = [ 1, 2, 3, 4, 5 ];
for (let i = 0; i < arr.length; i++) {
console.log('Array Item: ' + arr[i]);
}
Output:
javascript// Array Item: 1
// Array Item: 2
// Array Item: 3
// Array Item: 4
// Array Item: 5
Sometimes, one specific iteration can throw an error, use the try catch statement to catch the error and continue iterating.
How does the break statement work?
The break statement terminates a for loop execution.
javascriptfor (let i = 0; i < 5; i++) {
if (i === 2) {
break;
}
console.log('Iteration ' + i);
}
Output:
javascript// Iteration 0
// Iteration 1
As you can see, in this example, we terminate the for loop's execution after the index reaches 2, so that iterations 2-5 are not executed.
How does the continue statement work?
The continue statement skips (or jumps) to the next code iteration of the for loop.
javascriptfor (let i = 0; i < 5; i++) {
if (i === 2) {
continue;
}
console.log('Iteration ' + i);
}
Output:
javascript// Iteration 0
// Iteration 1
// Iteration 3
// Iteration 4
In this case, we skip the code block's execution when the index reaches 2.
How does the for..in statement work?
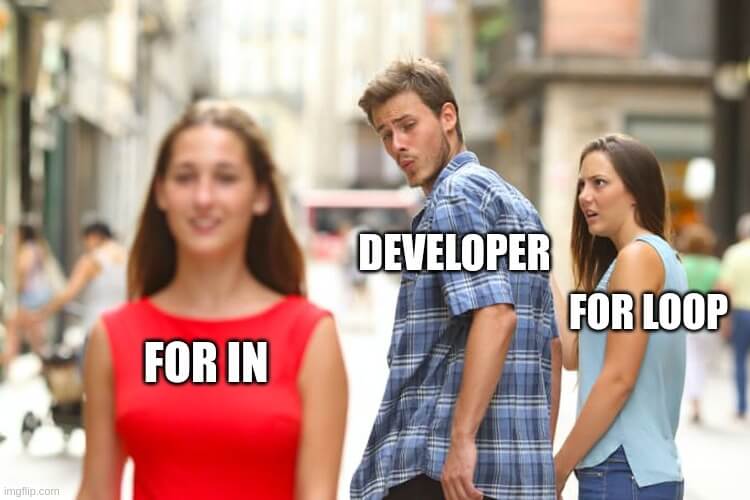
The for..in statement loops through the enumerable properties of an object.
You can use it to iterate over a map.
javascriptconst person = {
age: 26,
name: 'Tim'
};
for (let prop in person) {
console.log(prop);
}
// Outputs: 'age'
// Outputs: 'name'
How does the for..of statement work?
The for..of statement loops through the values of an iterable object.
javascriptconst animals = ["cat", "dog", "cow"];
for (let i of animals) {
console.log(i);
}
Output:
javascript// cat
// dog
// cow
P.S. The object must be iterable for this to work. (Array, Map, Set, String, etc...)
Is there an alternative to the for loop?
One of the alternatives of the for loop is to use the built-in forEach function. Generally, the forEach() function is slower than the for loop but is more readable because it doesn't involve any variable setup.
typescriptconst animals = ["cat", "dog", "cow"];
animals.forEach((animal) => {
console.log(animal);
});
In this case, we are using a function to iterate over an array.
Final Thoughts
Understanding the for loop is detrimental because without this statement it is impossible to be a developer. While the for loop is a basic statement, you must master it well, before moving to more difficult concepts.
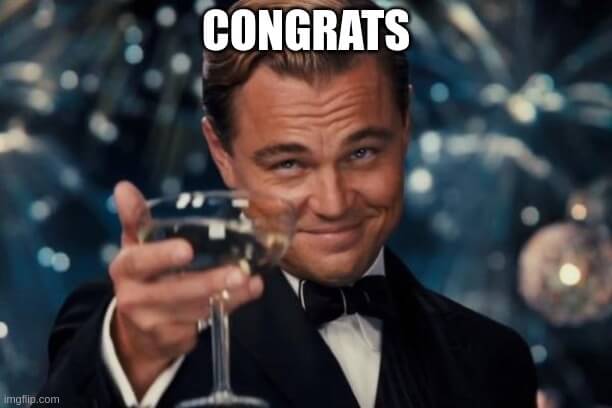
Thank you for reading this article, please share it!