How Does The Switch Statement Work In TypeScript?
In TypeScript, just like in most programming languages, the switch statement is part of the control flow statements that a developer can use.
The switch statement executes different code blocks based on a specific condition.
In this article, you will learn:
- How to use the switch statement
- What are the use-cases for the switch statement
- How is the switch statement different in TypeScript
Let's get to it. 😎
How does the switch statement work?
The switch statement is a control flow statement (just like the for loop).
The switch statement evaluates an expression and executes the code block that matched the condition.
Here is the syntax for the switch statement:
javascriptswitch (expression) {
case value1:
// code block 1
break;
case value2:
// code block 2
break;
default:
// default code block
break;
}
Explanation:
- The switch statement evaluates the expression.
- It executes the code block that matched the expression.
- The switch statement breaks out of the control flow with the break keyword associated with each case statement.
- If no case statement matched the condition, the switch statement looks for the optional default statement and executes its code.
Here is an example of the switch statement:
javascriptswitch (name) {
case 'Tim':
console.log('My name is Tim');
break;
case 'Georges':
console.log('My name is Georges');
break;
default:
console.log('I don\'t have a name');
break;
}
As you can see, the switch statement has similar behavior to the if...else statement, which raises a question...
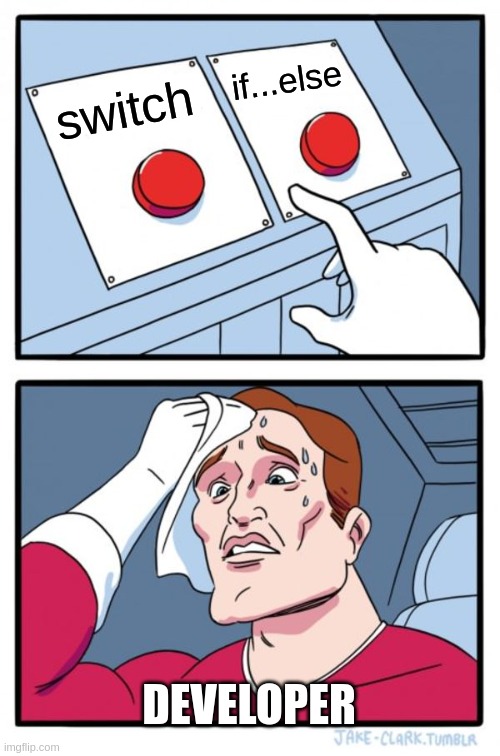
switch vs if else: Which one to use?
Use the switch statement when your expression is based on a single variable. In other cases use the if statement.
Switch | If...Else | |
---|---|---|
Checks for | Only checks for equality | Checks for equality and for logical expressions |
Evaluates | String, Number (only integer) | String, Number (with decimals), Boolean |
Refactoring | Easy | Can be harder |
How to use multiple values in one case statement?
The switch statement offers a great way of grouping multiple case statements into one.
Here is an example of this:
javascriptswitch (month) {
case 12:
case 1:
case 2:
console.log('This is winter');
break;
case 3:
case 4:
case 5:
console.log('This is spring');
break;
case 6:
case 7:
case 8:
console.log('This is summer');
break;
case 9:
case 10:
case 11:
console.log('This is autumn');
break;
default:
console.log('This is not a season');
break;
}
As you can see, in this example, we have grouped case statements by the season of the year.
Depending on the code inside the switch statement, it sometimes can throw an error. Use the try catch statement to catch it and handle it.
Does the switch statement provide a fall-through feature?
Yes, the switch statement provides a default statement that will execute if there is no case match.
The default statement is optional.
How to use the switch statement on an enum?
One of the better features of TypeScript is its addition of the enum feature. It allows the developer to define a set of named constants.
Of course, TypeScript allows you to use an enum with the switch statement.
typescriptenum MyDirection {
Up,
Down,
Left,
Right
}
const getDirection = (direction: MyDirection): void => {
switch (direction) {
case MyDirection.Up:
case MyDirection.Down:
console.log('Up/Down');
break;
case MyDirection.Left:
case MyDirection.Right:
console.log('Left/Right');
break;
}
};
getDirection(MyDirection.Up);
As you can see, in this example, we declare a function that logs a direction.
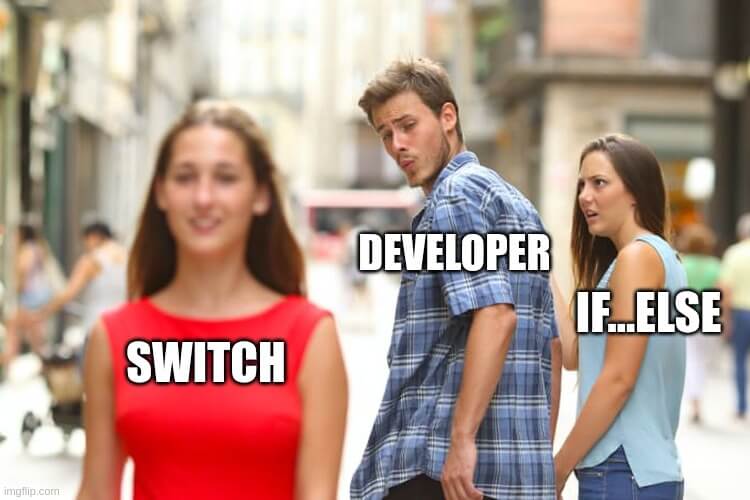
Final Thoughts
In TypeScript, I mostly use the switch statement when I work with enums. It provides a nice clean way of showing different code blocks conditions that is easy for developers to understand and refactor if needed.
I hope you liked the article, and you understand better the switch statement.
Please share it with your fellow developers.