How To Make An Optional Property In TypeScript?
When developing a TypeScript project, developers often need to make a property optional inside an interface, type, or class. Luckily, this is easy to do.
To mark a property as optional in TypeScript, you can place a question mark after the property name.
This article explains optional properties in TypeScript and shows multiple code examples.
Let's get to it 😎.
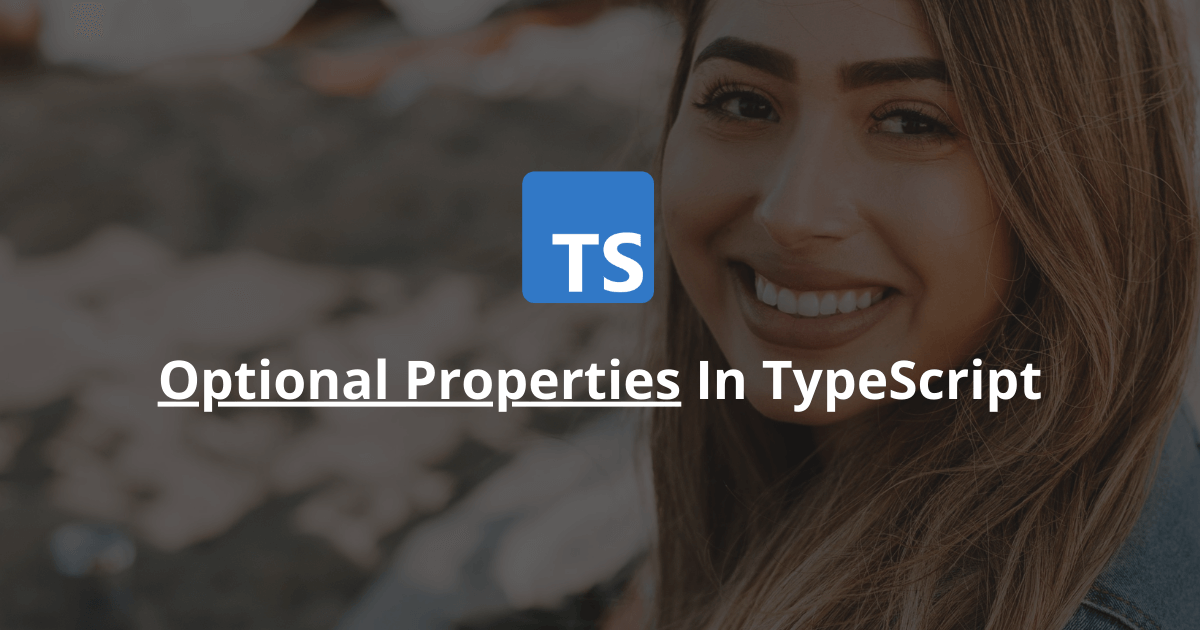
Making a property optional
By default, a property in TypeScript is required. If you don't pass a value to a required property, TypeScript's compiler will throw an error.
Let's have an example:
typescripttype Animal = {
name: string;
age: number;
}
const cow: Animal = {
name: 'Cow',
age: 4
};
Both the name AND the age properties are required. You will get an error if you omit to pass a value for one of them.
If you want to make one of those properties optional, add a question mark after the property name you want to make optional.
Here is an example:
typescripttype Animal = {
name: string;
age?: number;
}
const cow: Animal = {
name: 'Cow'
};
The age property is now optional, AND when we don't pass a value to it, the compiler doesn't complain. If you try to access the age property, you will get undefined.
P.S. You can pass undefined to any optional property in TypeScript. Indeed, TypeScript treats an optional property as a union type of its type AND undefined.
Making a property optional inside an interface
To mark a property as optional inside an interface, place a question mark after the property name.
Here is an example:
typescriptinterface IPerson {
name: string;
age?: number;
}
In this example, the age property is optional.
Making a property optional inside a class
To mark a property as optional inside a class, place a question mark after the class property name.
Here is an example:
typescriptclass Animal {
private name: string;
private age?: number;
public constructor(name: string, age?:number) {
this.name = name;
this.age = age;
}
}
In this example, the age class property is optional.
Final thoughts
As you can see, marking a property as optional is simple in TypeScript.
Just add a question mark after the property name.
If you need to make all properties inside a type optional, use the partial utility type.
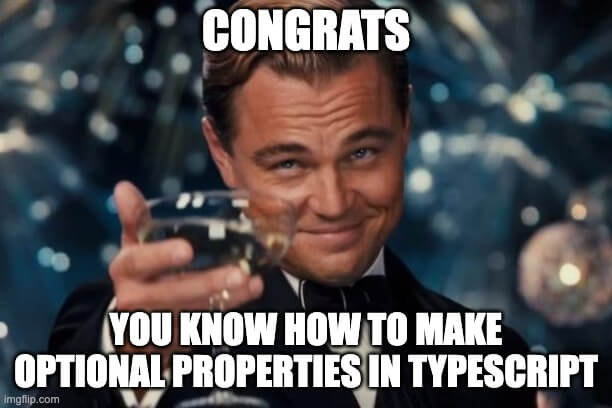
Here are some other TypeScript tutorials for you to enjoy: