How To Initialize An Empty Typed Object In TypeScript?
When developing a web application or TypeScript library, a developer may need to initialize an empty typed object. Luckily, it is easy to achieve.
To initialize an empty typed object in TypeScript, you can:
- Use type assertion
- Create a new class instance
- Use the partial utility type
- Use the record utility type
This article explains different methods to initialize an empty typed object in TypeScript with code examples.
Let's get to it 😎.
Method #1 - Use the type assertion
The first way to initialize an empty typed object is to cast the empty object using the as keyword to the type you need.
Here is an example:
typescriptinterface IPerson {
name: string;
age: number;
};
let person = {} as IPerson;
The issue with that method is that it is misleading.
According to that type, the person object has a name and age property, even though they don't exist.
Method #2 - Create a new class instance
Another method to initialize an empty typed object is to transform the typed object into a class.
Here is an example:
typescriptinterface IPerson {
name: string;
age: number;
};
class Person implements IPerson {
name: string = '';
age: number = 0;
}
let person = new Person();
Read more: Make a class implement an interface
The weakness of this method is that you need to transform an object into a class each time.
Also, with newer versions of TypeScript, you need to initialize each property.
Method #3 - Use the Partial utility type
Another option to initialize an empty typed object is to use the TypeScript partial utility type.
Here is an example:
typescriptinterface IPerson {
name: string;
age: number;
};
let person: Partial<IPerson> = {};
Read more: How do interfaces work in TypeScript?
The issue with this technique is that all of the properties of your object become optional, which is something you may not want.
Method #4 - Use the Record utility type
The most suitable option, in my opinion, is to initialize an empty typed object using the record utility type.
Here is an example:
typescriptinterface IPerson {
name: string;
age: number;
};
let person: IPerson | Record<string, never> = {};
In this case, the person object is either an empty object or a person, so it cannot be both.
Read more: The never type in TypeScript
Final thoughts
As you can see, a developer has multiple ways to initialize an empty typed object.
Compare the disadvantages of each to find the best method for your needs.
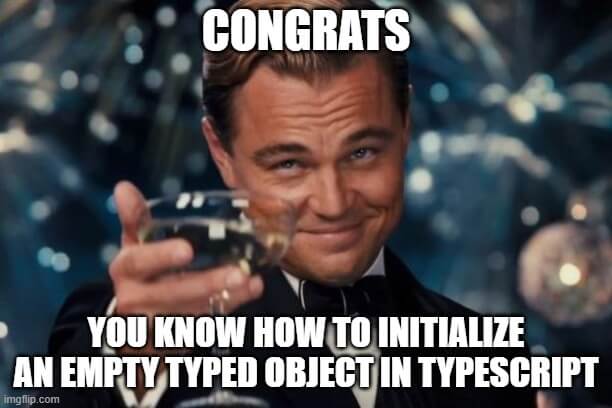
Here are some other TypeScript tutorials for you to enjoy: