How Does The NonNullable Type Work In TypeScript?
Sometimes when developing a TypeScript project, developers need to manipulate existing union types and remove null and undefined. Luckily, this task is easy to accomplish using the NonNullable type.
The NonNullable<Type> constructs a new type by excluding null and undefined from an existing type passed as an argument.
This article explains the NonNullable utility type in TypeScript with code examples.
Let's get to it 😎.
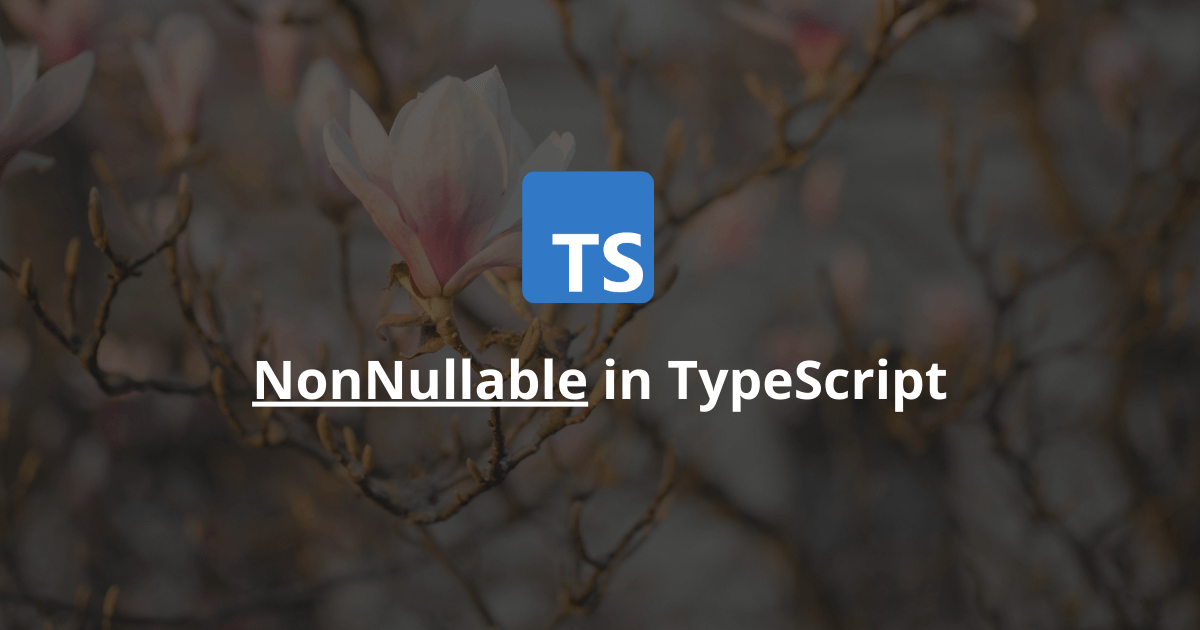
TypeScript offers many utility types, to help developers work and manipulate types. One of those types is the NonNullableutility type.
What is the NonNullable type?
The NonNullable<Type> creates a new type by excluding null and undefined from an existing type passed as an argument.
Confused? Let's review an example to understand this type better.
Let's say you have this type:
typescripttype OldType = string | number | boolean | null | undefined;
To remove null and undefined from that type, we could just copy it and remove undesired types manually, like so:
typescripttype NewType = string | number | boolean;
This method to accomplish this task is inefficient because each time you change something in the OldType you need to change the NewType. Wouldn't it be great if that could be done automatically?
And you can, using the NonNullable utility type.
typescript// Type: string | number | boolean
type NewType = NonNullable<OldType>;
Cool right?
Here is the full example:
typescripttype OldType = string | number | boolean | null | undefined;
// Type: string | number | boolean
type NewType = NonNullable<OldType>;
As you can see, the NewType doesn't contain undefined or null.
Final thoughts
As you can see, the NonNullable utility type is easy to use and important to understand to use TypeScript to its full potential.
TypeScript provides many other utility types, you can find many articles on this website explaining them.
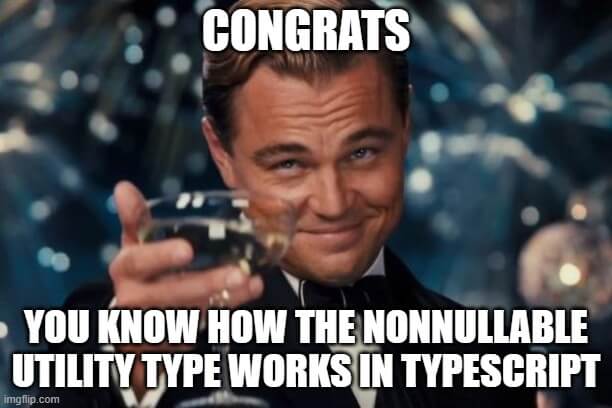
Here are some other TypeScript tutorials for you to enjoy: