How Does The Required Utility Type Work In TypeScript?
In TypeScript, sometimes a developer needs to set the optional properties from an interface as required without changing the original interface. Luckily, TypeScript offers a utility type called Required to help achieve this task.
The Required utility type accepts a type and constructs a new one with all the properties set to required.
This article explains the Required utility type and shows many code examples.
Let's get to it 😎.
The definition
The Required utility type, first introduced in TypeScript version 2.8, accepts a type AND constructs a new one with all the original properties set to required.
Here is the syntax:
typescriptRequired<Type>
You can pass a type or an interface as the Type generic parameter.
This utility type is the opposite of the TypeScript partial utility type.
Example
Let's have an example to understand the Required utility type better.
Let's say you have this interface:
typescriptinterface IPerson {
name: string;
age: number;
id?: number;
}
This interface has one optional property (id).
You want to create a method that accepts this interface, but you want to ensure that all the properties inside it are required.
This is where the Required utility type comes into play.
typescriptconst person: Required<IPerson> = {
name: 'Tim',
age: 27,
id: 1
};
Using the Required utility type, we ensure ALL the properties are populated.
If we try to omit the id field when using this utility type, we will get a TypeScript error.
typescript// We get an error:
// Property 'id' is missing in type
// '{ name: string; age: number; }'
// but required in type 'Required<IPerson>'.(2741)
const person: Required<IPerson> = {
name: 'Tim',
age: 27,
};
Cool, right?
How to require one property?
If you have an interface with many optional properties AND want to make only one required, you can combine the Required and Pick utility types.
To do it, we can create a helper type like this one:
typescriptinterface IPerson {
name: string;
age?: number;
id?: number;
}
type CustomRequire<T, K extends keyof T> = T & Required<Pick<T, K>>;
const person: CustomRequire<IPerson, 'age'> = {
name: 'Tim',
age: 27,
};
In this example, the age property is required, AND the id property is optional.
You can also pass multiple properties to the CustomRequire helper type using a union type to make multiple properties required.
Read more: How does the TypeScript Pick type work?
Final thoughts
As you can see, making properties required using the Required utility type is simple.
Use this utility type alone or with other utility types in your next TypeScript project!
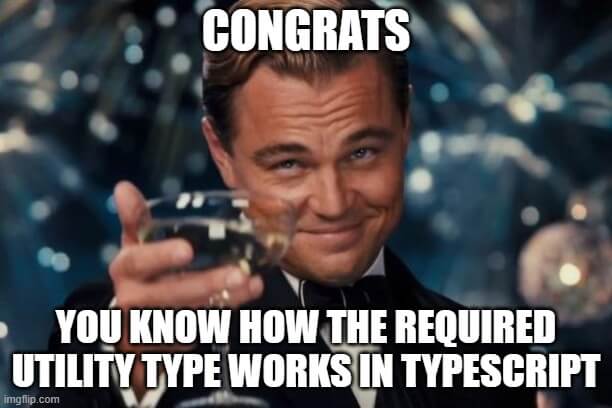
Here are some other TypeScript tutorials for you to enjoy: