How Does The ReturnType Utility Type Work In TypeScript?
TypeScript offers many utility types to help developers work better with types. One of them is the ReturnType utility type. But what is it, and how does it work?
The ReturnType utility type helps you create a new type from a function's return type.
This article explains the ReturnType utility type in TypeScript with code examples.
Let's get to it 😎.
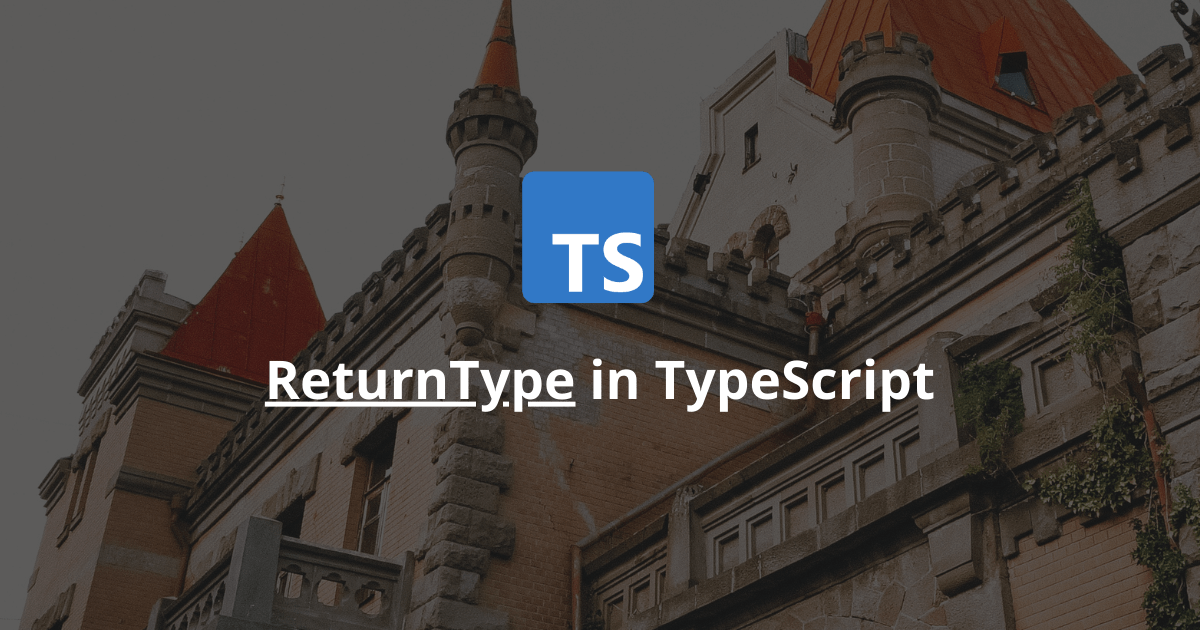
What is the ReturnType type?
The ReturnType utility type, released in TypeScript version 2.8, lets a developer construct a new type from the return type of a function.
This type works similarly to the Parameters utility type.
Here is an example:
typescriptconst getData = (name: string, age: number) => {
return { age, name };
}
// Type is: { age, name }
type NewType = ReturnType<typeof getData>;
As you can see, the NewType contains the age and name properties AND matches the getData function's return type.
This utility type is useful when you want to reuse a function's return type that is either:
- Not defined
- Very complicated
- Defined in an external library
- etc.
What about async functions?
If you try using the ReturnType utility type with an async function, the function's return type will be inside a promise.
Here is an example:
typescriptconst getData = async (name: string, age: number) => {
return { age, name };
}
// Type is: Promise<{ age, name }>
type NewType = ReturnType<typeof getData>;
To unwrap the promise from the return type, you need to use the Awaited type. This type works similarly to the await keyword.
Here is an example:
typescriptconst getData = async (name: string, age: number) => {
return { age, name };
}
// Type is: { age, name }
type NewType = Awaited<ReturnType<typeof getData>>;
As you can see, the NewType type is unwrapped from the promise.
How to get the return type of a generic function?
The ReturnType utility type also works with generic functions.
Here is an example:
typescriptconst makeBox = <T,>(value: T) =>{
return { value };
};
// Type is { value: number }
type NewType = ReturnType<typeof makeBox<number>>;
You can also omit passing a generic type argument to the makeBox function. In that case, the value's type will be unknown.
typescriptconst makeBox = <T,>(value: T) =>{
return { value };
};
// Type is { value: unknown }
type NewType = ReturnType<typeof makeBox>;
If you don't want the generic argument to be unknown, set a default value for the generic argument, like so:
typescriptconst makeBox = <T extends number>(value: T) =>{
return { value };
};
// Type is { value: number }
type NewType = ReturnType<typeof makeBox>;
Final thoughts
As you can see, the ReturnType utility type is useful when you want to get a function's return type.
Use this type well in your next TypeScript project!
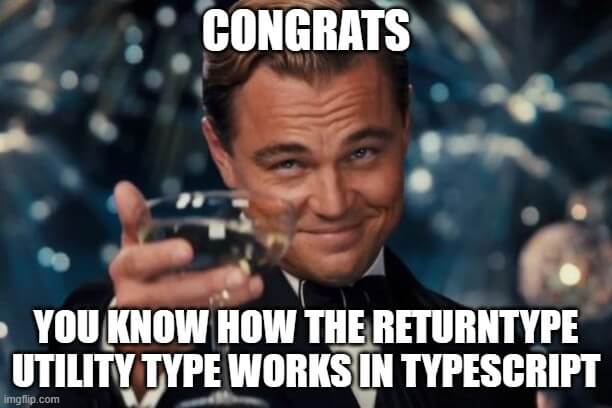
Here are some other TypeScript tutorials for you to enjoy: