How To Loop Through An Array Of Objects In React?
A common task for web developers when working on a React web application is to loop through an array of objects and render components for each item. Luckily, React with JavaScript offer different simple ways to archive it.
To loop through an array of objects in React, you have different options:
- Use the map function
- Use the forEach function
- Use a for loop
This article will go through various methods of looping through an array of objects in React and show multiple examples.
Let's get to it 😎.
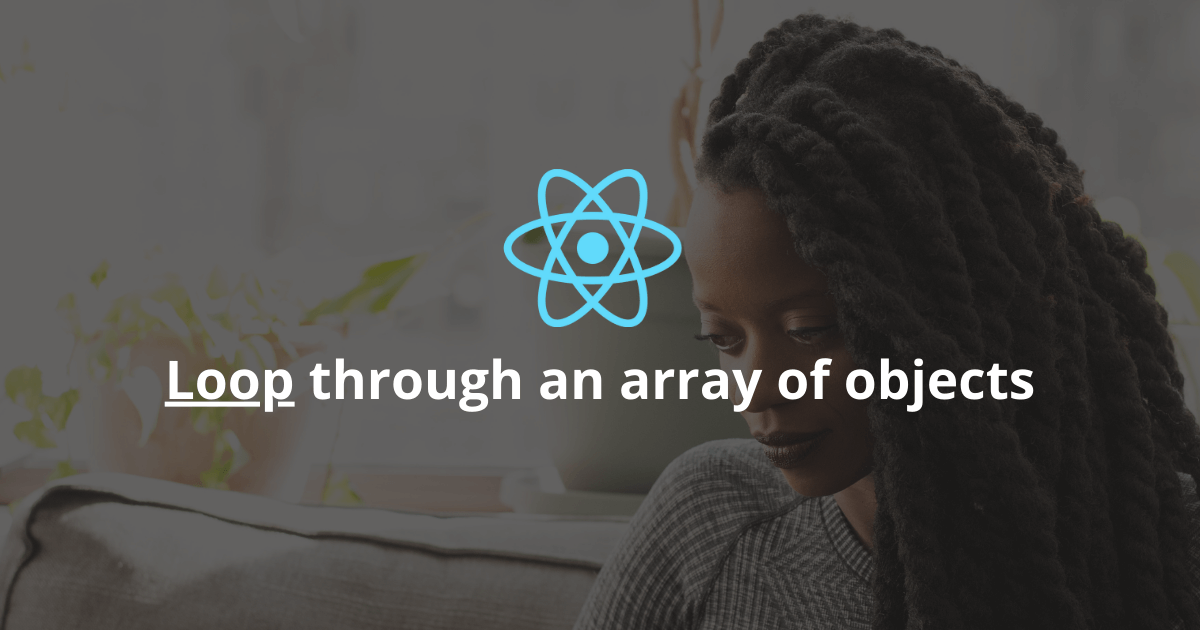
Method #1 - Loop using the map function
The first method to loop through an array of objects involves using the JavaScript map function with a callback that returns the React component.
Each component you generate from the array of objects must have a unique key. This key is mandatory when you are working with lists in React. It helps spot changes inside the list.
Here is how to use the map function to loop through an array in React:
javascriptimport React from "react";
import ReactDOM from "react-dom";
const animals = [
{
type: "cat",
age: 3
},
{
type: "dog",
age: 2
}
];
const App = () => (
<>
{animals.map((animal, index) => (
<div key={index}>
<div>Type: {animal.type}</div>
<div>Age: {animal.age}</div>
</div>
))}
</>
);
ReactDOM.render(<App />, document.getElementById("container"));
In this example, we are mapping through the "animals" array and creating a component for each item.
Note: Usually, using the index as the key isn't recommended, but since the array is not going to change, it isn't an issue.
Method #2 - Loop using the forEach function
Another method to loop through an array of objects is using the forEach function to create a list of React components. Then, we return this list to render it.
This function works similarly to a for loop.
Here is an example of how to achieve it:
javascriptimport React from "react";
import ReactDOM from "react-dom";
const animals = [
{
type: "dog",
age: 2
},
{
type: "cat",
age: 3
}
];
const App = () => {
const list = [];
animals.forEach((animal, index) => {
list.push(
<div key={index}>
<div>Type: {animal.type}</div>
<div>Age: {animal.age}</div>
</div>
);
});
return list;
};
ReactDOM.render(<App />, document.getElementById("container"));
One advantage of this method is you can externalize the logic of building the list into a separate function. That way, you have a code separated into smaller pieces, AND you can reuse the function in other components.
Method #3 - Loop using a for loop
The final method is building a list of components with the for loop.
Here is an example of how to achieve it:
javascriptimport React from "react";
import ReactDOM from "react-dom";
const animals = [
{
type: "dog",
age: 2
},
{
type: "cat",
age: 3
}
];
const App = () => {
const list = [];
for (let i in animals) {
list.push(
<div key={i}>
<div>Type: {animals[i].type}</div>
<div>Age: {animals[i].age}</div>
</div>
);
}
return list;
};
ReactDOM.render(<App />, document.getElementById("container"));
You can also use other for loop variations to archive this task, like the for of loop.
Final thoughts
As you can see, looping through an array of objects in React is easy.
You can use different JavaScript methods (the forEach function or the map function) or a for loop to achieve it.
Each of those ways is similar, so you can choose according to your preferences.
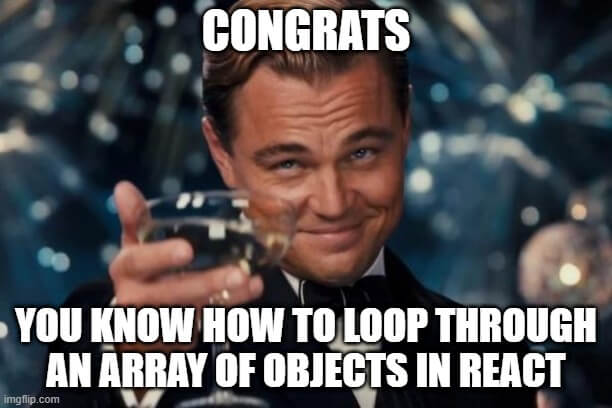
Here are some other React tutorials for you to enjoy: