How To Pass A Parameter Through onClick In React?
React provides a simple way of handling events for front-end developers. A common use case for React developers is passing an extra parameter through the onClick event to the callback function. Luckily, React makes it easy to do.
This article will show different ways to pass a parameter through the onClick event in React with examples.
Let's get to it 😎.
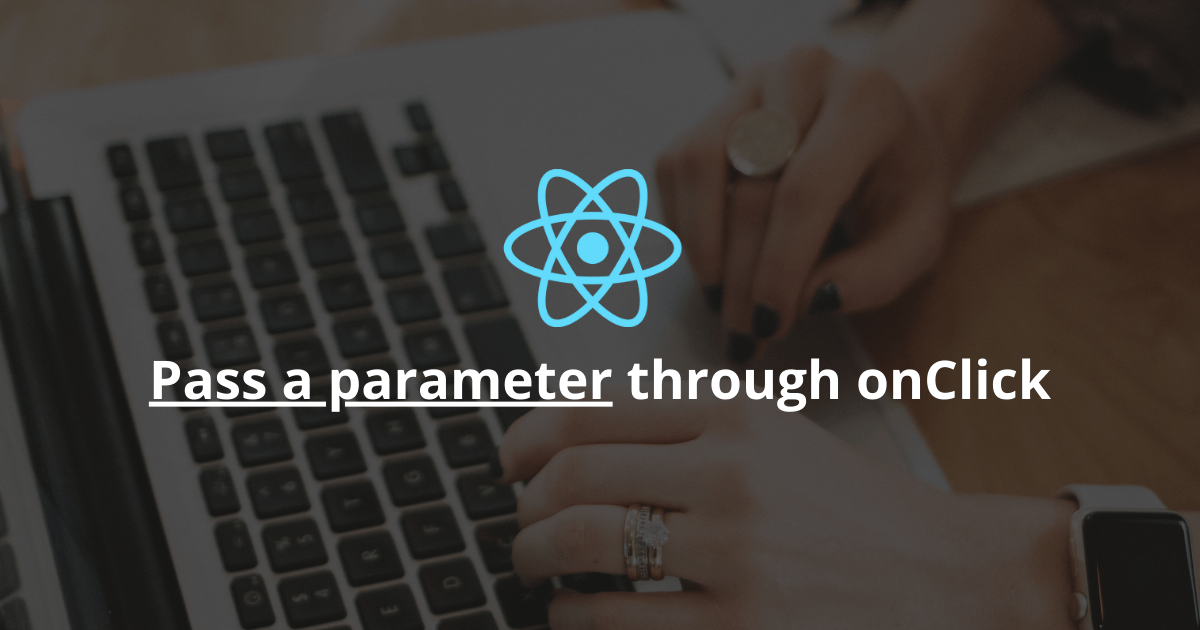
Method #1 - Pass a function that returns a function
One way to pass a parameter through an onClick event is to create a function that returns a function.
Here is how to make it work:
- Create the first function that accepts the parameter you want to pass and return the second function from inside.
- Create the second function that accepts the React mouse event and code all the logic.
Here is a demonstration of how to achieve it:
javascriptimport React from "react";
import ReactDOM from "react-dom";
const App = () => {
const handleClick = (str) => () => {
console.log(str);
};
return (
<button onClick={handleClick("apply clicked")}>
apply
</button>
);
};
ReactDOM.render(<App />, document.getElementById("container"));
Creating a function that returns a function may be considered confusing by some developers.
Another way of using the same method is to pass an inline function as the onClick argument. This function then calls the callback function.
Here is a demonstration of how to achieve it:
javascriptimport React from "react";
import ReactDOM from "react-dom";
const App = () => {
const handleClick = (str) => {
console.log(str);
};
return (
<button onClick={() => handleClick("apply clicked")}>
apply
</button>
);
};
ReactDOM.render(<App />, document.getElementById("container"));
Note: Using this technique, you will not be able to use the mouse event from the click event unless passed explicitly.
Method #2 - Add a custom data attribute
One lesser-known technique to pass an extra parameter through an onClick event in React is to use custom data attributes.
Here is how to make it work:
- Add a custom data attribute with the parameter's value to the element with the onClick handler.
- In the event callback, use the mouse event to get the parameter.
Here is a demonstration of how to achieve it:
javascriptimport React from "react";
import ReactDOM from "react-dom";
const App = () => {
const handleClick = (e) => {
console.log(e.currentTarget.getAttribute("data-value"));
};
return (
<button data-value="apply clicked" onClick={handleClick}>
apply
</button>
);
};
ReactDOM.render(<App />, document.getElementById("container"));
This method is great when you want an element from the DOM also to contain the parameter's value.
Note: currentTarget refers to the element to which the event is attached.
Final thoughts
As you can see, passing an extra parameter to the onClick event is simple in React.
You can always pass an object if you want to pass multiple extra parameters.
For my part, I use the first method since creating custom data attributes is often unnecessary.
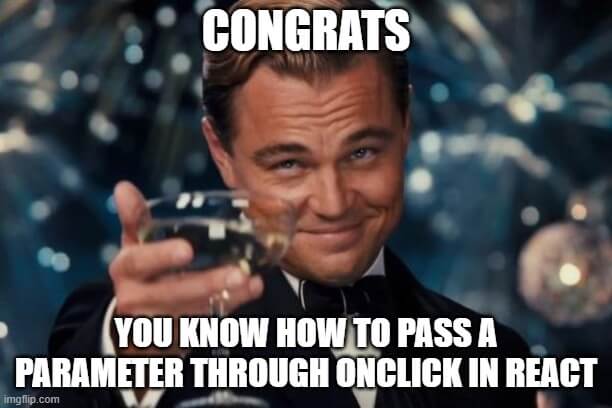
Here are some other React tutorials for you to enjoy: