How To Pass A Function As A Prop In React?
React code is usually separated into components, independent bits of code that you can reuse. To pass data from component to component, developers can pass data as props. Sometimes, developers need to pass a function as a prop. Luckily, it is easy to achieve in React.
This article will go through passing a function as a prop in React and answer some of the most common questions.
Let's get to it 😎.
How to pass a function from a parent to a child?
Passing a function as a prop to a child component involves:
- Creating the function handler in the parent component.
- Passing down the function as a prop.
Here is an example of passing a function to a child:
javascriptimport React from "react";
import ReactDOM from "react-dom";
const Child = ({ handleClick }) => {
return <button onClick={handleClick}>Apply</button>;
};
const Parent = () => {
const handleClick = () => {
console.log("parent click");
};
return <Child handleClick={handleClick} />;
};
ReactDOM.render(<Parent />, document.getElementById("container"));
In this example, the Parent component passes the handleClick function to the Child component.
Note: For this example to work, a div with an id of "container" must exist on the page.
Passing props from a parent to a child is easy, but what about passing it the other way around?
How to pass a function from a child to a parent?
To pass a function from a child to a parent, you need to:
- Create a function in the parent component that accepts a callback as an argument.
- Pass the function to the child component as a prop.
- In the child component, create the function you want to pass UP to the parent.
- Call the parent function prop in the child component with the child function as the argument.
- Call the child function within the parent component.
Here is an example of passing a function to a parent:
javascriptimport React from "react";
import ReactDOM from "react-dom";
const Child = ({ handleChild }) => {
const func = () => {
console.log("child function");
};
return (
<button onClick={() => handleChild(func)}>Apply</button>
);
};
const Parent = () => {
const handleChild = (callback) => {
// Here, you have the function from the child.
callback();
};
return <Child handleChild={handleChild} />;
};
ReactDOM.render(<Parent />, document.getElementById("container"));
Note: For this example to work, a div with an id of "container" must exist on the page.
Final thoughts
As you can see, passing functions as props is easy in React.
However, if you have many different props to pass up and down, this can quickly become hard to manage.
In this situation, I recommend using the React Context API or a state management library like Redux or Zustand. A state management library is going to help you simplify managing different props.
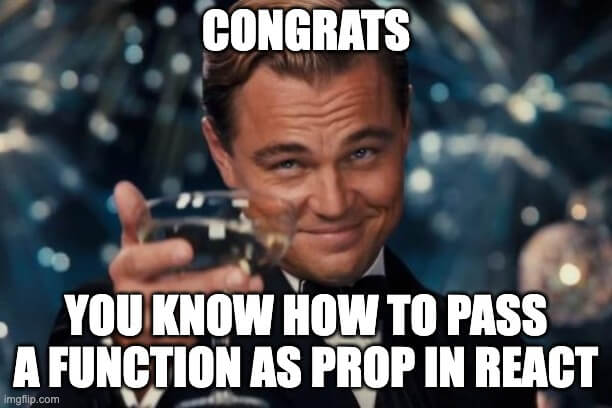
Here are some other React tutorials for you to enjoy: