How To Map An Array Of Objects In React?
One of the most typical tasks when working on a React application is rendering a list of objects as components. The easiest way to map an array of objects in React is to use the JavaScript map function.
This article will go through using the map function and show different examples of mapping an array of objects in React.
Let's get to it 😎.
How does the map function work?
The map JavaScript function creates a new array from the results of the provided callback function.
This callback function gets called for each element of the array.
Here is a small example:
javascriptconst array = [1, 2, 3];
const newArray = array.map((x) => x * 2);
// Outputs: [ 2, 4, 6 ]
console.log(newArray);
In this example, the map function returns a new array with the doubled numbers from the original collection.
How to use the map function in React?
You can render a list of multiple components using the map function in React.
Here is an example of accomplishing it in React:
javascriptimport React from "react";
import ReactDOM from "react-dom";
const App = () => {
const array = [1, 2, 3];
return (
<>
{array.map((x) => (
<div key={String(x)}>{x}</div>
))}
</>
);
};
ReactDOM.render(<App />, document.getElementById("container"));
When rendering a list of components, you must provide a unique key for each element.
The key must be a string that uniquely identifies the item from the list.
React uses keys to spot changes inside a list of components.
Note: Only use the object index as the key when you do not have a stable unique identifier.
How to map over an array of objects in React?
To render a list of components from an array of objects in React, you have two options:
- Use the map function
- Use a loop or the forEach function
Method #1 - Using the map function
Here is an example of mapping over an array of objects:
javascriptimport React from "react";
import ReactDOM from "react-dom";
const array = [
{
name: "Tim",
age: 27
},
{
name: "Bob",
age: 32
}
];
const App = () => (
<>
{array.map((item, index) => (
<div key={index}>
<div>Name: {item.name}</div>
<div>Age: {item.age}</div>
</div>
))}
</>
);
ReactDOM.render(<App />, document.getElementById("container"));
Since we do not have a stable unique identifier for each element, we use the item's index as the key. Another alternative would be to use a combination of the name and the age to create a unique key.
Method #2 - Using the forEach function
Another option to render a list of objects in React is to create an array of components using a forEach function and then render it.
Here is an example of how to accomplish it:
javascriptimport React from "react";
import ReactDOM from "react-dom";
const array = [
{
name: "Tim",
age: 27
},
{
name: "Bob",
age: 32
}
];
const App = () => {
const list = [];
array.forEach((item, index) => {
list.push(
<div key={index}>
<div>Name: {item.name}</div>
<div>Age: {item.age}</div>
</div>
);
});
return list;
};
ReactDOM.render(<App />, document.getElementById("container"));
This approach is practical when you do not want to render the list immediately. You can, for example, externalize the logic into a separate function that you can reuse.
Note: In this case, we are using the forEach method, but you can also use a for loop instead.
Final thoughts
As you can see, mapping over an array of objects in React is simple.
You have multiple choices: the map function, the forEach function, and the for loop.
Which one you choose is primarily a question of preference.
Always provide a unique key for each element inside the list. Otherwise, you may encounter unexpected behavior.
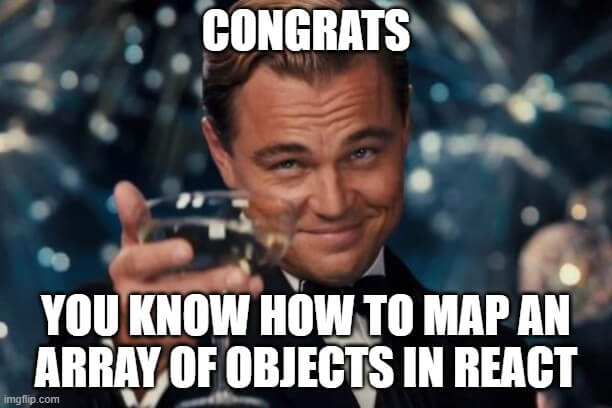
Here are some other React tutorials for you to enjoy: