How To Add A Conditional ClassName In React?
React offers multiple ways to style its components. One of the most uncomplicated is to use classes like you would with HTML/CSS. In React, you add CSS classes using the className property. But how do you add a conditional className in React?
To add a conditional className in React, you can:
- Add it using a ternary operator
- Add it using an if statement
- Add it using the classnames npm library
This article will go through different methods to add a conditional class in React and show various examples.
Let's get to it 😎.
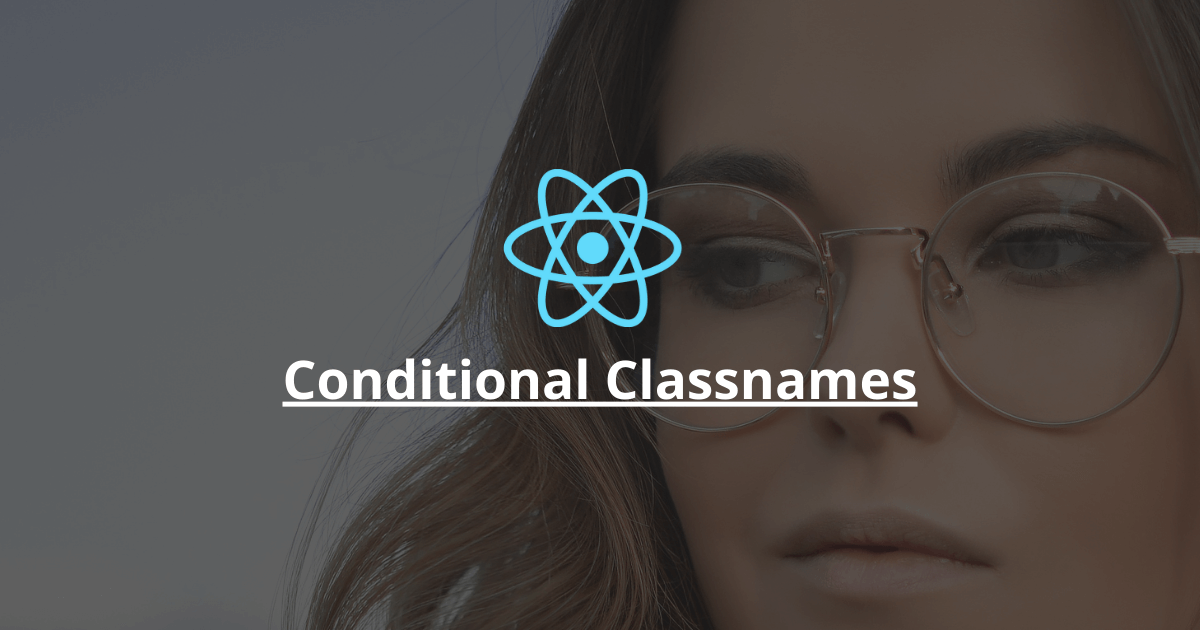
Method #1 - With a ternary operator
The easiest way to add a class conditionally is to use a ternary operator.
Here is an example of how to achieve it:
javascriptimport React from "react";
import ReactDOM from "react-dom";
const App = ({ active }) => {
return <div className={active ? "active" : ""}></div>;
};
ReactDOM.render(<App active={true} />, document.getElementById("container"));
In this example, the App component returns a div. This div can have a class called "active", but only if the component has an active property.
Method #2 - Using an if statement
Another way to conditionally add a class is to use an if statement. This method is handy when adding multiple classes.
Here is an example of how to achieve it:
javascriptimport React from "react";
import ReactDOM from "react-dom";
const App = ({ active, error }) => {
let classNames = [];
if (active) {
classNames.push("active");
}
if (error) {
classNames.push("error");
}
return <div className={classNames.join(" ")}></div>;
};
ReactDOM.render(<App active error />, document.getElementById("container"));
In this example, we populate an array of classes conditionally. Then we join this array and output it as a string.
As you can see, this method can quickly become hard to maintain.
Luckily, a solution exists!
Method #3 - With the classname library
Another solution to add conditional classes in React is to use the classnames library.
Classnames is a popular library that simplifies conditional classes in React.
Here is an example of how to use it:
javascriptimport React from "react";
import ReactDOM from "react-dom";
import classNames from "classnames";
const App = ({ active }) => {
const className = classNames({
active: active
});
return <div className={className}></div>;
};
ReactDOM.render(<App active />, document.getElementById("container"));
The classNames library will output the active class only if the active property is true.
How to add multiple conditional classes in React?
To add multiple conditional classes in React, you can also use the classnames library.
Here is an example of how to achieve it:
javascriptimport React from "react";
import ReactDOM from "react-dom";
import classNames from "classnames";
const App = ({ active, error }) => {
const className = classNames({
active: active,
error: error
});
return <div className={className}></div>;
};
ReactDOM.render(<App active />, document.getElementById("container"));
Final thoughts
As you can see, adding conditional classes in React is simple.
I recommend using the classNames library to create your conditional classes. Without it, your code will be harder to read and maintain.
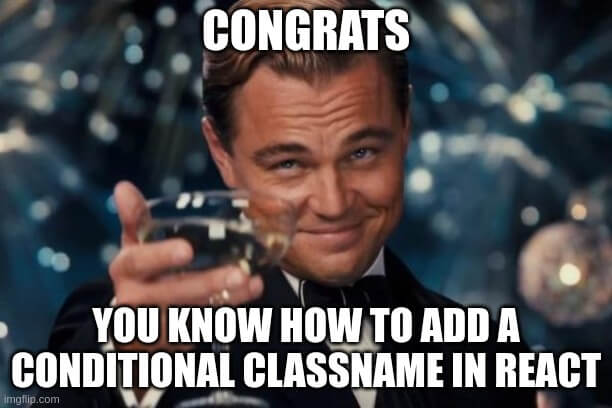
Here are some other React tutorials for you to enjoy: