How To Get An Element By Id In React?
One of the primary benefits of using React is that it simplifies web application development. In React, you do not manipulate DOM elements directly. Instead, you work with components. However, there might be a time when you need direct access to a DOM element. Luckily, it is easy to accomplish.
The simplest way to access an element by id in React is to use a reference with the useRef hook.
This article will go through references in React, the useRef hook, and explain how to get an element by id in React.
Let's get to it 😎.
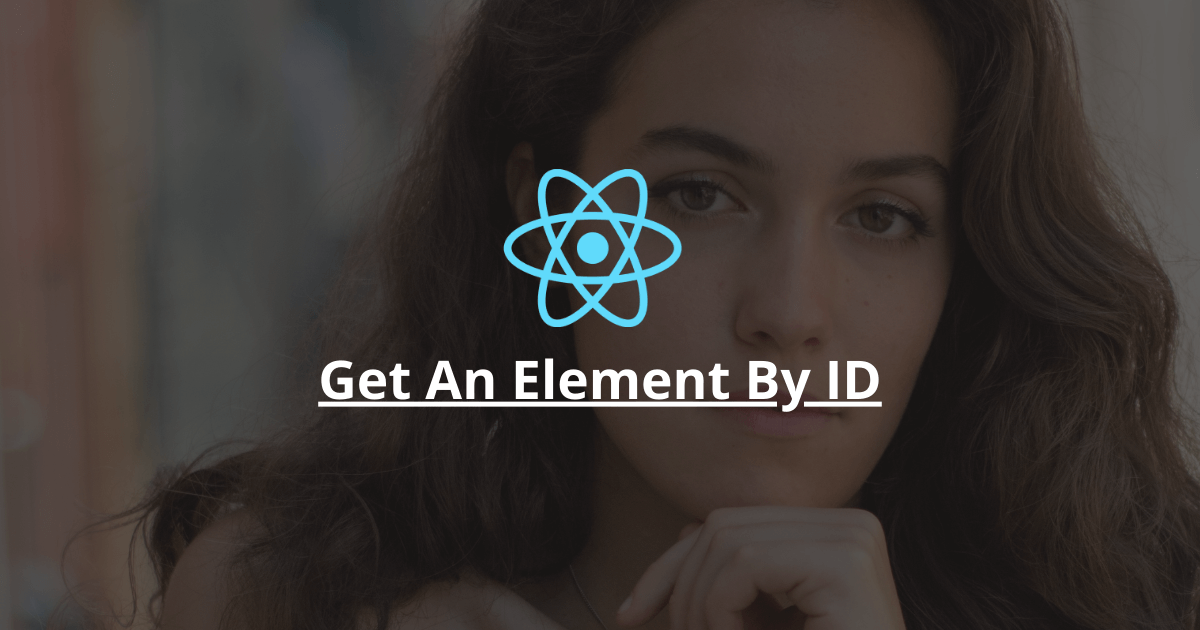
What are refs in React?
A ref can store a reference to a DOM or React element.
It provides a way to change a child component without using props.
Here are some valid use cases for a ref:
- When managing focus, text selection, or media playbacks.
- When managing animations.
- When integrating with a third-party library.
To access the content of the reference, you must use its current property. This property is set after the referenced element renders.
Note: It is essential to know that you should only use refs when what you are doing cannot be accomplished by using props. Also, don't overuse refs because it can cause performance issues.
How to get an element by id?
Even though in React, you can use JavaScript methods like getElementById, they are better alternatives.
It is better to get the DOM element by using a ref.
Here are the steps to follow to get a DOM element:
- You create a reference using the useRef hook and pass it to the DOM element.
- You access the DOM element using the current property of the ref inside a useEffect callback.
Here is an example of how to accomplish it:
javascriptimport React, { useEffect } from "react";
import { createRoot } from "react-dom/client";
const App = () => {
const ref = React.useRef(null);
useEffect(() => {
// The DOM element is accessible here.
console.log(ref.current);
}, []);
return <div ref={ref}></div>;
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
We pass null as the initial value for the useRef hook. This way, we know when the DOM element renders.
Also, we provide an empty dependency array to the useEffect hook. This way, the callback only runs once when the component renders (it acts similarly to a componentDidMount).
How to get a DOM element inside an event?
One common scenario is to access a DOM element when an event happens.
Here is an example of how to accomplish it:
javascriptimport React from "react";
import { createRoot } from "react-dom/client";
const App = () => {
const ref = React.useRef(null);
const handleClick = () => {
// The DOM element is also accessible here.
console.log(ref.current);
};
return (
<>
<button onClick={handleClick}>submit</button>
<div ref={ref}></div>
</>
);
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
In this example, the console outputs the div element when a user clicks the button.
Final thoughts
As you can see, refs make accessing DOM elements in React easy.
They make a good substitute for using the JavaScript getElementById function.
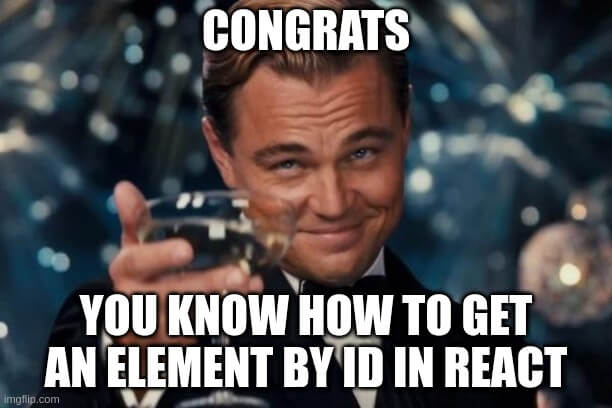
Here are some other React tutorials for you to enjoy: