How To Use The ForEach Function In React?
JavaScript offers many ways to iterate over an array, for example, the for loop, the map function, and the forEach function, which is the subject of this React guide.
In React, the forEach function is used to iterate over an array of objects and populate an array of elements.
In this article, I explain the forEach function, how to use it in React, and show code examples.
Let's get to it 😎.
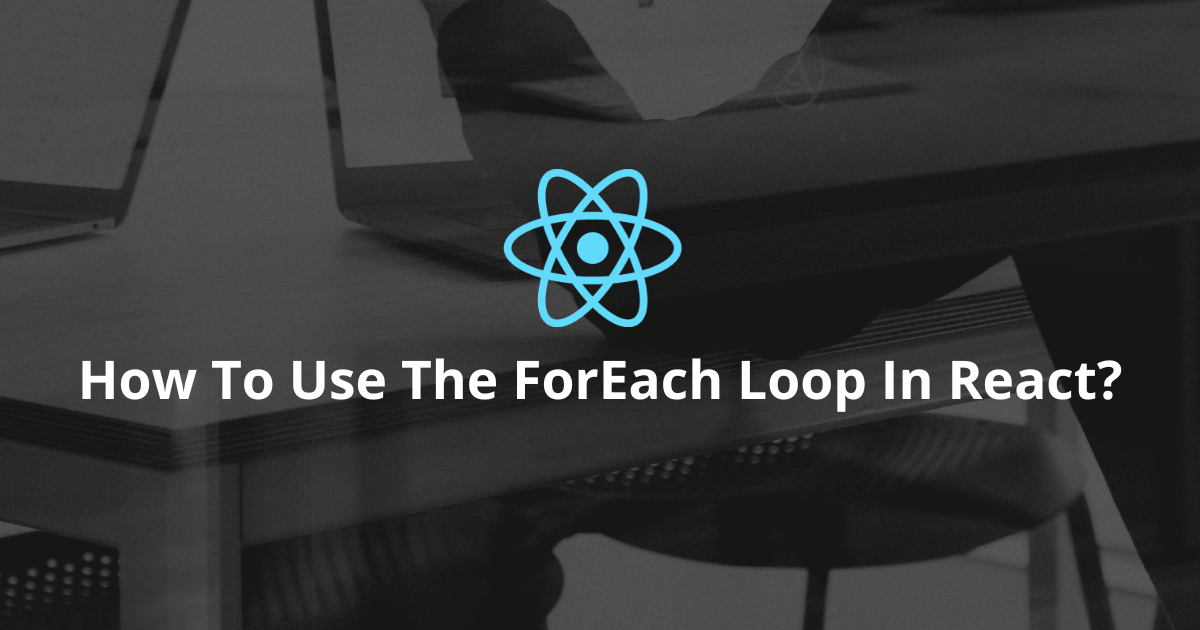
The definition
The forEach function executes a callback on each item of an array.
This function is an ES5 feature supported by all major browsers, including IE9.
Here is a small example:
javascriptconst names = ["Tim", "Jack", "Bob"];
names.forEach((name) => {
console.log(name);
});
// Outputs:
// Tim
// Jack
// Bob
In this example, we iterate over the names and output each name.
Note: This function only works on arrays. If you try to use it on something else, it will give you an error (forEach is not a function).
I wrote a guide on the forEach function on this website (if you want to learn more).
How to use the forEach function in React?
In React, we can use the forEach function to create an array of React elements outside the render function. For example, we can create a helper function that returns an array of components and use it when needed.
Here is a small example:
javascriptimport React from "react";
import { createRoot } from "react-dom/client";
const getNameElements = () => {
const names = ["Tim", "Jack", "Bob"];
const elements = [];
names.forEach((name) => {
elements.push(<div key={name}>{name}</div>);
});
return elements;
};
const App = () => {
const elements = getNameElements();
return elements;
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
We first create a helper function, inside of which we create an array of components with the help of the forEach function.
Then, inside the App component, we output all of those elements.
Alternatively, you can use a for loop inside the helper function.
Note: If you want to iterate directly inside the render, use the map function.
Final thoughts
In conclusion, using the forEach function in React is simple.
We can use it to create an array of components.
If you want to loop in a React JSX, use the map function.
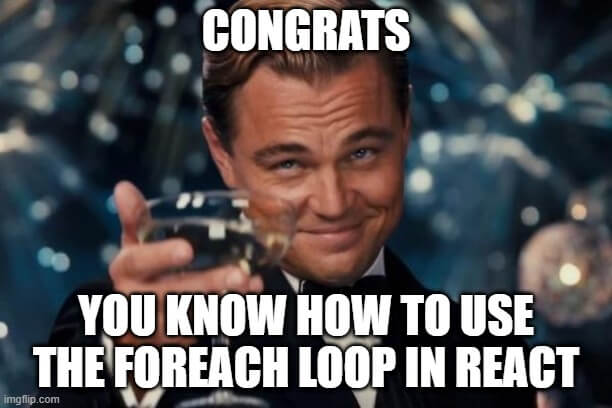
Here are some other React tutorials for you to enjoy: