How To Center A Component In React? (Vertical & Horizontal)
When developing a React web application, a developer often wants to center containers within the user screen. Luckily, React makes it easy to do it with the help of various CSS properties.
To center a component in React, you have multiple options:
- Use the flexbox layout
- Use the absolute position with a transform
This article will analyze those solutions and show how to code them in React.
Let's get to it 😎.
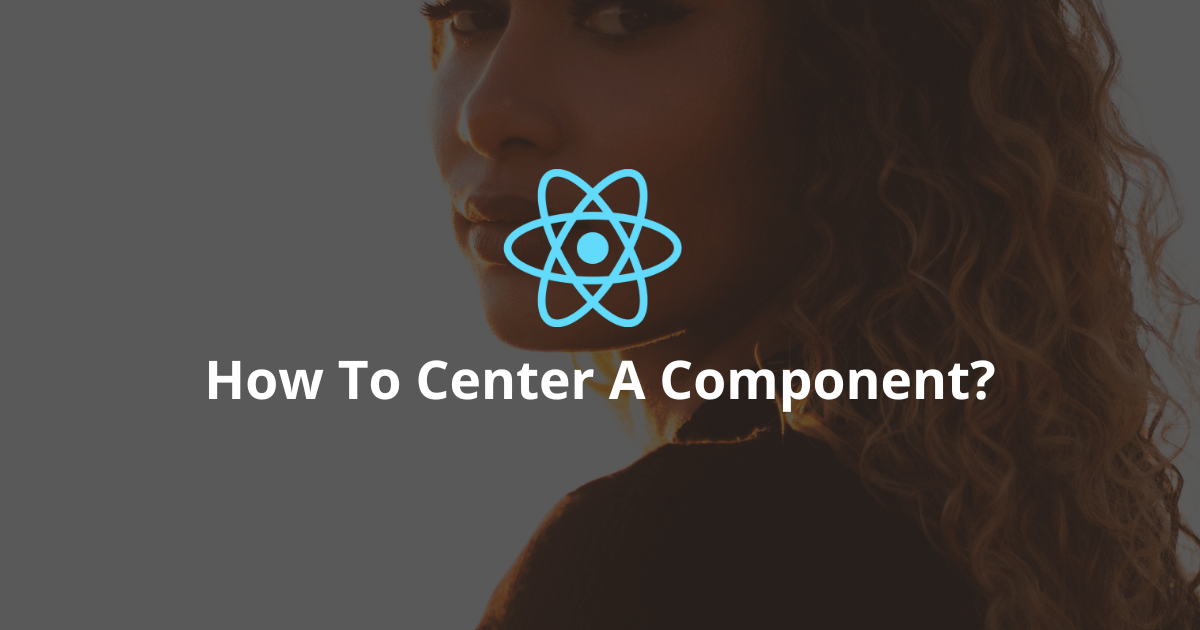
Method #1 - Center a container using the flexbox layout
The easiest method to center a div in React is to use the CSS flexbox layout model.
The flexbox layout is a new CSS3 layout that simplifies layout structure design.
All modern browsers (including IE11) support the flexbox layout.
Here is how to center a div vertically:
javascriptimport { createRoot } from "react-dom/client";
const App = () => {
return (
<div
style={{
display: "flex",
alignItems: "center",
height: "100%"
}}
>
<div style={{ background: "red" }}>content</div>
</div>
);
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
For this to work, we need to include this CSS with the application code:
CSShtml, body, #root { height: 100%; }
Here is how to center a div both horizontally & vertically:
javascriptimport { createRoot } from "react-dom/client";
const App = () => {
return (
<div
style={{
display: "flex",
alignItems: "center",
justifyContent: "center",
height: "100%"
}}
>
<div style={{ background: "red" }}>content</div>
</div>
);
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
And here is the CSS to add:
CSShtml, body, #root { height: 100%; }
Note: I added a red background around the div, making it more apparent that it is centered.
Method #2 - Center a container using the absolute position.
Another method to center a container involves:
- Using the position property with an absolute value.
- Adding the top & left properties to approximately center the container.
- Adding a transform property to move the div to the center.
Here is how to center a div vertically:
javascriptimport { createRoot } from "react-dom/client";
const App = () => {
return (
<div
style={{
position: "absolute",
top: "50%",
transform: "translate(0px, -50%)",
background: "red"
}}
>
content
</div>
);
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
Here is how to center a div both horizontally & vertically:
javascriptimport { createRoot } from "react-dom/client";
const App = () => {
return (
<div
style={{
position: "absolute",
top: "50%",
left: "50%",
transform: "translate(-50%, -50%)",
background: "red"
}}
>
content
</div>
);
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
How to center text in React?
To center text in React, we need to use the CSS text-align property.
Here is how to do it:
javascriptimport { createRoot } from "react-dom/client";
const App = () => {
return (
<div
style={{
textAlign: "center"
}}
>
content
</div>
);
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
Final thoughts
As you can see, centering a container in React is simple.
You have multiple ways of achieving it: using a flexbox, an absolute position div, or a div table.
Which one you select is a matter of preference.
For my part, I mostly use the flexbox layout method.
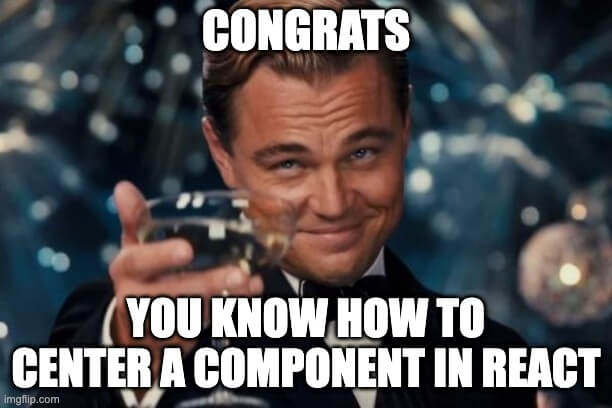
Here are some other tutorials for you to enjoy: