How To Create A Back Button With A React Router?
The react-router package is a fantastic library that enables React applications to have different routes that users can view. It has various features; however, one of its most significant ones is its ability to manipulate the browser history inside the application.
Sometimes, it is helpful for users to go back inside the SPA. That's when a back button can help. Luckily, it is easy to do.
To create a back button in React, you need to:
- Use the useNavigate hook when the user clicks on the button
This article will go through the complete solution step-by-step.
Let's get to it 😎.
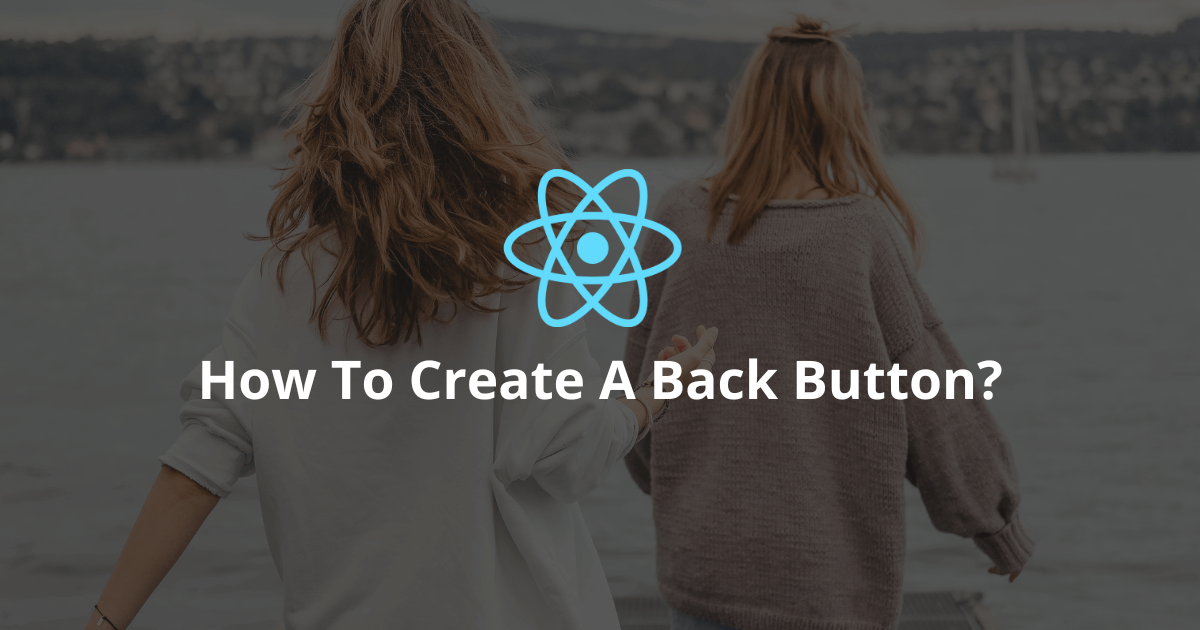
The react-router library is a lightweight package that brings easy routing to React.
You can install it like this:
bashnpm install react-router-dom
How to create a back button with a react-router?
Since the react-router package has an internal history management API, we cannot use the default JavaScript History API.
To create a back button with the react-router package, we need to:
- Add the useNavigate hook to the component where you have the button.
- Call this hook's result with a -1 to go back one page (inside the button's click event callback)
Here are the steps to follow:
1. First, let's add a React application with a few routes.
javascriptimport { createRoot } from "react-dom/client";
import { BrowserRouter, Routes, Route } from "react-router-dom";
const Index = () => <div>Index</div>;
const Contact = () => <div>Contact</div>;
const App = () => {
return (
<Routes>
<Route path="/" element={<Index />} />
<Route path="/contact" element={<Contact />} />
</Routes>
);
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(
<BrowserRouter>
<App />
</BrowserRouter>
);
This application contains two routes: the index and the contact route.
2. Then, let's create a navigation component that is going to enable us to navigate through the application:
javascriptconst Navigation = () => {
const navigate = useNavigate();
return (
<>
<div>
<Link to="/">Home</Link>
</div>
<div>
<Link to="/contact">Contact</Link>
</div>
<button onClick={() => navigate(-1)}>Go back</button>
<button onClick={() => navigate(1)}>Go forward</button>
</>
);
};
This component contains links to navigate between routes.
Also, it contains two buttons:
- The first one, when clicked, brings the history back.
- The second one, when clicked, brings the history forward.
3. Let's combine everything!
javascriptimport { createRoot } from "react-dom/client";
import {
BrowserRouter,
Routes,
Route,
Link,
useNavigate
} from "react-router-dom";
const Index = () => <div>Index</div>;
const Contact = () => <div>Contact</div>;
const Navigation = () => {
const navigate = useNavigate();
return (
<>
<div>
<Link to="/">Home</Link>
</div>
<div>
<Link to="/contact">Contact</Link>
</div>
<button onClick={() => navigate(-1)}>Go back</button>
<button onClick={() => navigate(1)}>Go forward</button>
</>
);
};
const App = () => {
return (
<>
<Navigation />
<Routes>
<Route path="/" element={<Index />} />
<Route path="/contact" element={<Contact />} />
</Routes>
</>
);
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(
<BrowserRouter>
<App />
</BrowserRouter>
);
As you can see, those two buttons manipulate the history of the application.
Note: If you want to go back or forward a few pages, you must put the number of pages inside the navigate function.
Final thoughts
As you can see, manipulating the history of a React web application is simple with the react-router package.
Happy coding!
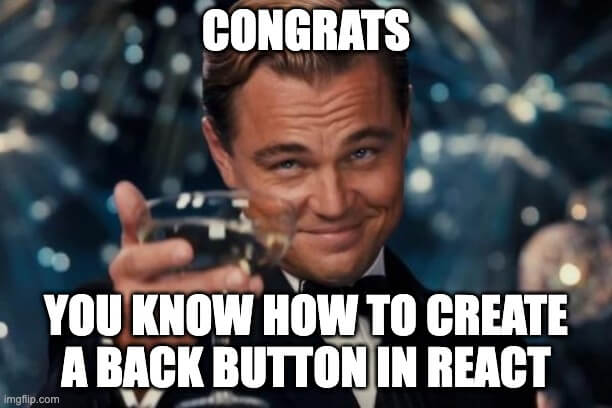
Here are some other React tutorials for you to enjoy: