How To Refresh A Page In React?
When developing your next hit web application project in React, you may need to refresh a page. But how do you do it?
This article explains how to refresh a page in React, shows how to persist the state between reloads, and shows code examples.
Let's get to it 😎.
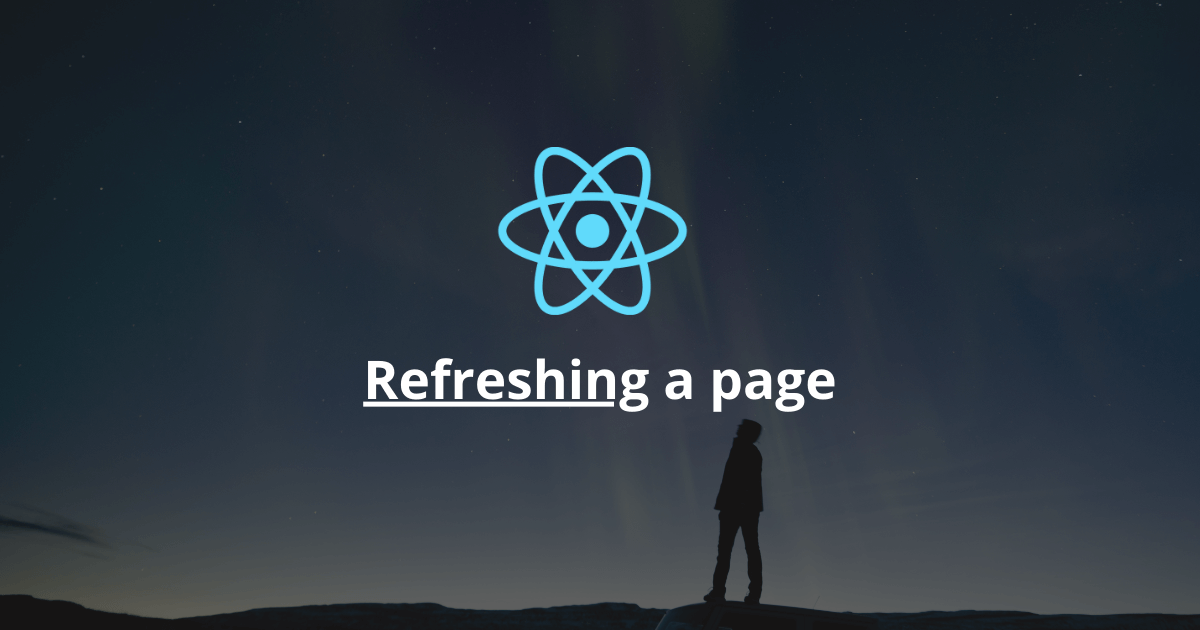
Reloading a page in React
To refresh a page written in JavaScript, you can use the reload function provided by the location object.
Here is the function:
javascriptwindow.location.reload();
This function reloads the current URL and acts like the reload browser button.
To reload a page from a React application, use the same function.
Here is an example:
javascriptimport React from 'react';
export const App = () => {
const refresh = () => window.location.reload(true)
return (
<button onClick={refresh}>Refresh</button>
)
}
When a user clicks on the button, the page will refresh.
Easy right?
But what if you need to maintain the application's state between page reloads?
Maintaining the state after a page refresh
You have multiple options to maintain an application's state after a page refreshes. You can use one of the following methods:
- Use your browser's local storage.
- Use the URL query parameters.
- Utilize an external library (like Redux Persist).
Here is an example of how you can persist the state using local storage and React hooks:
javascriptimport React, {useEffect, useState} from 'react';
export const App = () => {
const [count, setCount] = useState(1);
useEffect(() => {
setCount(JSON.parse(window.localStorage.getItem("count")));
}, []);
useEffect(() => {
window.localStorage.setItem("count", count);
}, [count]);
return (
<div className="App">
<h1>Count: {count}</h1>
<button onClick={() => setCount(count + 1)}>Increment</button>
<button onClick={() => window.location.reload(true)}>Refresh</button>
</div>
);
}
In this example, the count value is persisted inside your browser's local storage.
To test the state persistence, click the first button a few times to increment the counter.
Then, press the refresh button. When the page reloads the old count will appear!
How to refresh a page every 5 seconds?
To reload a page every X seconds, you can use the window.location.reload function inside a timeout.
Here is an example:
javascriptsetTimeout(() => {
window.location.reload();
}, 5000);
Final thoughts
As you can see, refreshing a page is simple in JavaScript and React.
Use this new knowledge well in your next or current React project.
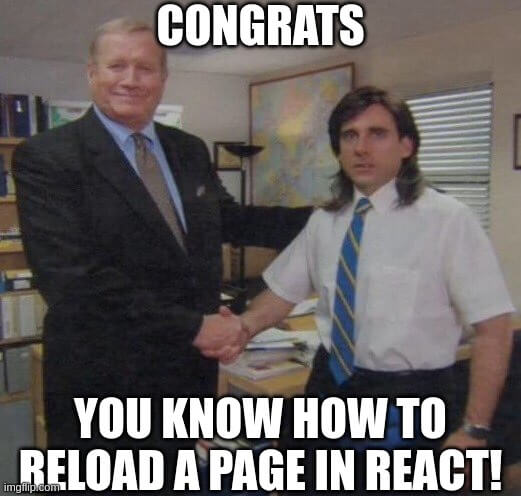
Here are some other React tutorials for you to enjoy: