How To Redirect To Another Page In React?
When working on a React web development project, a developer may need to redirect to another page. But, how do you do it?
This article explains how to redirect to an external URL in React and shows multiple code examples.
Let's get to it 😎.
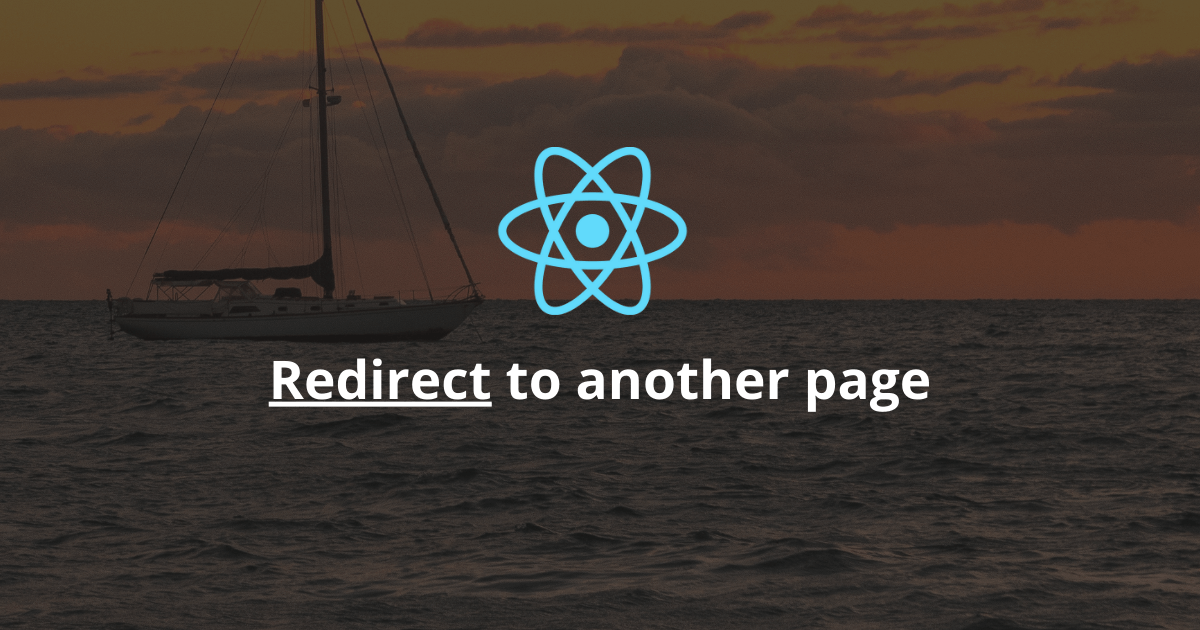
Redirecting to an external URL
To redirect a JavaScript application to an external URL, you can use the window.location.replace function.
Here is an example:
javascriptwindow.location.replace('https://timmousk.com/');
This code redirects to my blog (the chosen URL is just a coincidence of course).
To redirect to another page inside a React application, you can use the same function inside a callback function or an effect.
Here is an example:
javascriptimport React, { useEffect } from "react";
import { createRoot } from "react-dom/client";
const App = () => {
useEffect(() => {
const timeout = setTimeout(() => {
window.location.replace('https://timmousk.com/');
}, 2000);
return () => clearTimeout(timeout);
}, []);
return <>Will redirect in 2 seconds...</>;
}
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
In this example, we redirect to an external URL two seconds after the React application creation.
Alternative way
Instead of using the window.location.replace method, you can use the window.location.href function to change the active page URL.
Here is an example:
javascriptimport React, { useEffect } from "react";
import { createRoot } from "react-dom/client";
const App = () => {
const redirect = () => {
window.location.href = 'https://timmousk.com/';
}
return <button onClick={redirect}>redirect</button>;
}
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
In this example, we redirect to another URL when the user clicks on the button.
How to redirect using react-router?
The react-router package is one of the most popular routers for React. It offers all the necessary features (like nested routes, scroll restoration, skeleton UI using suspense, error handling, etc.) that a React developer might need.
If you need to redirect to a different path when using the react-router package, use the useNavigate method.
Here is an example:
javascriptimport { createRoot } from "react-dom/client";
import {
BrowserRouter, Routes, Route, useNavigate
} from "react-router-dom";
const Index = () => {
const navigate = useNavigate();
const redirect = () => {
navigate('/new-page')
}
return <button onClick={redirect}>redirect</button>
};
const NewPage = () => <div>Contact</div>;
const App = () => {
return (
<>
<Routes>
<Route path="/" element={<Index />} />
<Route path="/new-page" element={<NewPage />} />
</Routes>
</>
);
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(
<BrowserRouter>
<App />
</BrowserRouter>
);
In this code, we have two routes.
When we click on the index's page button we navigate to the new page.
Easy right?
P.S. If you need to replace the current URL using the useNavigate method, you need to pass an object as the second parameter with the replace property set to true.
Final thoughts
As you can see, redirecting to an external URL is easy in React.
Simply use the window.location.replace or window.location.href JavaScript methods!
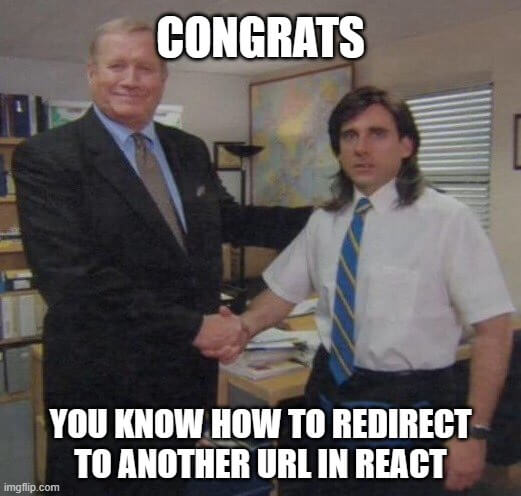
Here are some other React tutorials for you to enjoy: