How To Get The Current URL In React?
Whether you are creating a single-page application or an e-commerce store, there is often a situation when you need to get the URL of the current page. Luckily, this is easy to accomplish in React.
To get the current URL in React, you can:
- Use the window.location JavaScript object.
- Use the useLocation hook from the react-router-dom library.
This article will analyze both solutions and show examples for each.
Let's get to it 😎.
Method #1 - Using the window.location object
In JavaScript, the window.location object helps a developer manipulate the current URL of the page.
It returns multiple properties. Here are some of them:
- The href - Returns the URL of the current page.
- The protocol - Returns the current page's protocol (HTTP, HTTPS).
- The hostname - Returns the domain name of the current page.
- The pathname - Returns the path of the current page.
- The port - Returns the port of the current page.
Let's analyze how this object works with the current page of this blog:
javascript// Outputs: 'https://timmousk.com/blog/react-get-current-url/'
console.log(window.location.href)
// Outputs: 'https:'
console.log(window.location.protocol)
// Outputs: 'timmousk.com'
console.log(window.location.hostname)
// Outputs: '/blog/react-get-current-url/'
console.log(window.location.pathname)
You can also use this object to get the current URL inside a React web application.
Here is a code example of how to accomplish it:
javascriptimport React from "react";
import { createRoot } from "react-dom/client";
const App = () => {
const href = window.location.href;
return <div>{href}</div>;
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
This example outputs the current URL inside a div element.
Method #2 - Using the useLocation hook
If you are creating a single-page application, you are most likely using the react-router-dom library.
This library is a lightweight package that adds routing to your React application.
When using this library, you can use the useLocation hook to access the current URL instead of using the JavaScript window.location object.
Here is a code example of getting the current URL with the useLocation hook:
javascriptimport React from "react";
import { BrowserRouter, Routes, Route, useLocation } from "react-router-dom";
import { createRoot } from "react-dom/client";
const All = () => {
const location = useLocation();
// The current location.
console.log(location);
return <div>{location.pathname}</div>;
};
const App = () => {
return (
<BrowserRouter>
<Routes>
<Route path="/" element={<All />} />
</Routes>
</BrowserRouter>
);
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
The useLocation hook returns an object containing the information about the current URL.
Here are the properties it returns:
- The hash - Returns the anchor part of the current page.
- The pathname - Returns the path of the current page.
- The search - Returns the query part of the current page.
Note: You can only use the useLocation hook inside a route.
Final thoughts
As you can see, getting the current URL inside a React web application is simple.
If you are using the react-router-dom library, use the hook it provides.
Else, use the window.location JavaScript object.
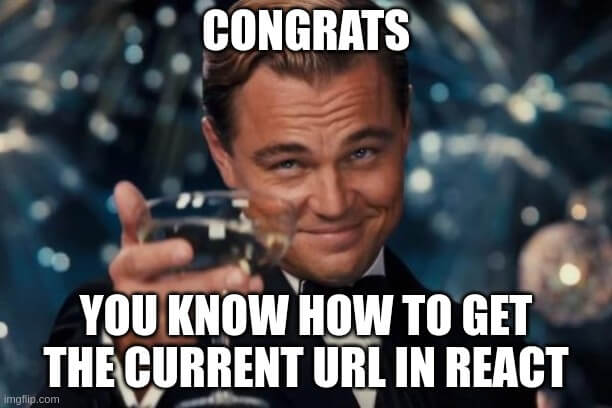
Here are some other React tutorials for you to enjoy: