How To Clear An Input In React?
Unlike other frameworks, React doesn't provide support for two-way data binding with form inputs. In React, form management is more complicated because you need to manage it yourself. This brings the question of how to clear an input in React.
To clear an input in React, you can:
- Set the input state's value to empty (for a controlled input).
- Clear the input using a reference (for an uncontrolled input).
This article will explore clearing a controlled VS an uncontrolled input and show real-life examples.
Let's get to it 😎.
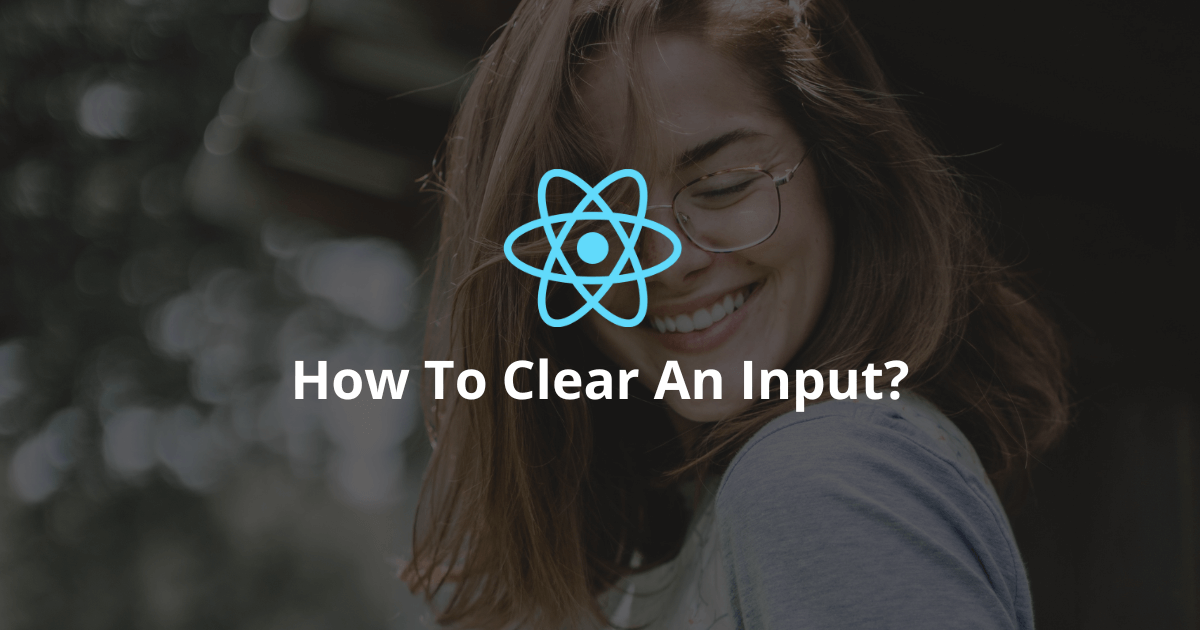
In React, you can manage an input's state using two ways:
1. Controlled
The input's state is managed by a parent component that uses state and callbacks.
2. Uncontrolled
The input stores its state internally, and React uses a reference to query it.
Which one of those components you use will dictate what method you need to choose to clear an input.
How to clear a controlled input?
The easiest way to clear a controlled input is to set the state that controls the input's value to empty.
Here is how to accomplish it:
javascriptimport React, { useState } from "react";
import { createRoot } from "react-dom/client";
const App = () => {
const [value, setValue] = useState("");
const onInput = (e) => setValue(e.target.value);
const onClear = () => {
setValue("");
};
return (
<>
<input value={value} onInput={onInput} />
<button type="button" onClick={onClear}>
clear
</button>
</>
);
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
As you can see, in this code example, we have two elements: an input and a button.
The state manages the input's value using the input event callback and the value property.
When the button gets clicked, the input's state gets cleared.
How to clear an uncontrolled input?
The easiest way to clear an uncontrolled input is to set the input's value to empty using its reference.
Here is how to accomplish it:
javascriptimport React, { useRef } from "react";
import { createRoot } from "react-dom/client";
const App = () => {
const ref = useRef(null);
const onClear = () => {
ref.current.value = "";
};
return (
<>
<input ref={ref} />
<button type="button" onClick={onClear}>
clear
</button>
</>
);
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
As you can see, in this code example, we first create a reference using the useRef hook and pass it to the input.
Then, in the button click event callback, we clear the value using the current property of the reference.
How to clear an input after a form submit?
To clear an input after a form submit, you need to set the state to empty in the submit event callback.
Here is how to accomplish it:
javascriptimport React, { useState } from "react";
import { createRoot } from "react-dom/client";
const App = () => {
const [state, setState] = useState("");
const onInput = (e) => setState(e.target.value);
const onSubmit = () => {
setState("");
};
return (
<form onSubmit={onSubmit}>
<input value={state} onInput={onInput} />
</form>
);
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
Another way to clear an input is to use the form reset function:
javascriptimport React, { useState } from "react";
import { createRoot } from "react-dom/client";
const App = () => {
const [state, setState] = useState("");
const onInput = (e) => setState(e.target.value);
const onSubmit = (e) => {
e.target.reset();
};
return (
<form onSubmit={onSubmit}>
<input value={state} onInput={onInput} />
</form>
);
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
This way is excellent when used with uncontrolled inputs.
Final thoughts
Whether you are using a controlled or an uncontrolled input, clearing an input is simple in React.
Both methods have advantages and weaknesses; ultimately, your choice depends on your requirements.
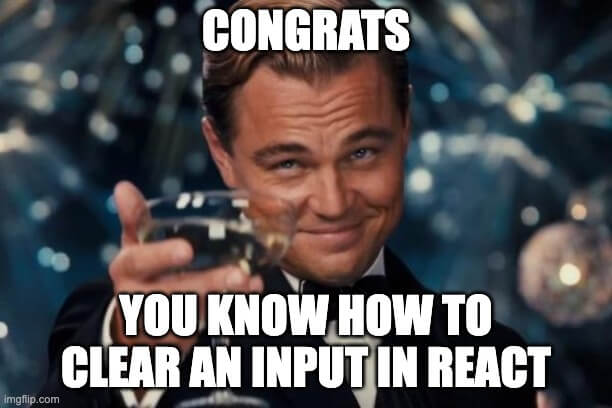
Here are some other React tutorials for you to enjoy: