How To Open A Link In A New Tab In React?
React provides a simple way to build web applications. Often, those applications require adding links to other Internet resources. This brings the question of how to open a link in a new tab. Luckily it is easy to accomplish.
In React, to open a link in a new tab, you can:
- Create a hyperlink element with a blank target.
- Use the window.open function with a blank target.
This article will analyze both solutions and show real-life examples for each.
Let's get to it 😎.
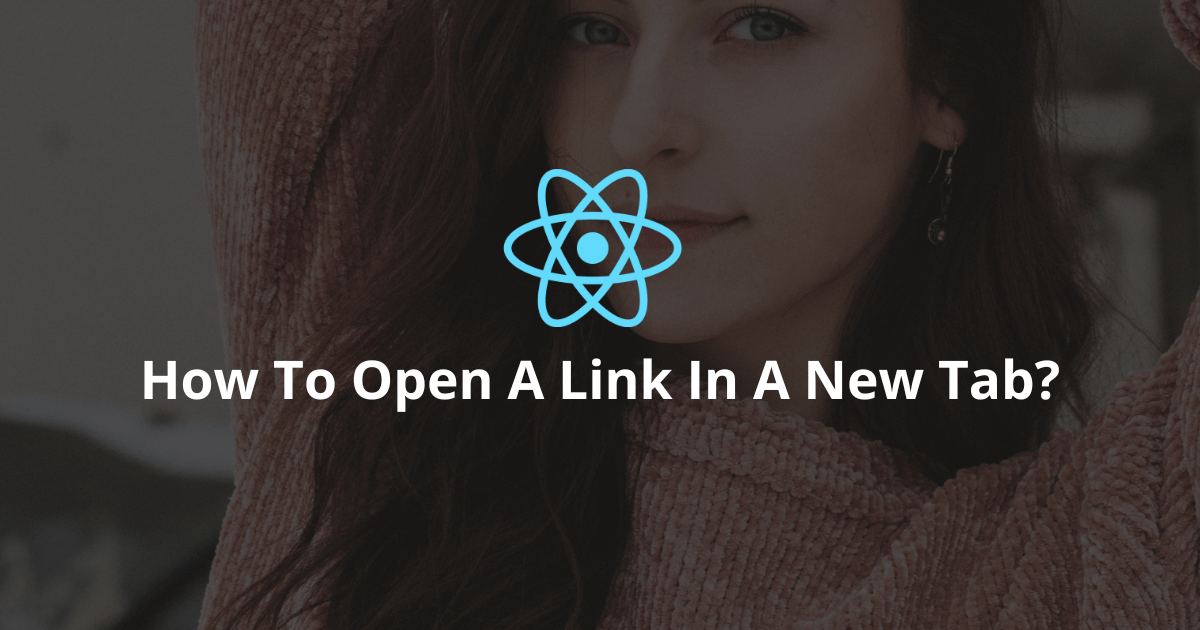
Method #1 - Create a hyperlink using a blank target
The easiest way to open a link in a new tab is to create a hyperlink with a blank target.
Here is how to accomplish it:
javascriptimport React from "react";
import { createRoot } from "react-dom/client";
const App = () => {
return (
<a href="https://timmousk.com" target="_blank" rel="noopener noreferrer">
link
</a>
);
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
In this code example, when the user clicks on the hyperlink element, it will open the link in a new tab.
Maybe you have noticed that we added a rel attribute with noopener and noreferrer to the hyperlink element. This prevents potentially hijacking the browser with rogue JavaScript.
Note: The target attribute species where the hyperlink will be opened. Its possible values include _blank, _self, _parent, and _top.
Method #2 - Use the window.open function
The second method to open a link in a new tab in React is to use the window.open JavaScript function.
This approach is practical when you need to open a link conditionally.
Here is how to accomplish it:
javascriptimport React from "react";
import { createRoot } from "react-dom/client";
const App = () => {
const onClick = () => {
window.open("https://timmousk.com", "_blank", "noopener,noreferrer");
};
return <div onClick={onClick}>link</div>;
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
The window.open function accepts three arguments:
- The URL - The path of the resource to load.
- The target - The browser context where the resource will be loaded.
- The window features - A list of window features.
Nowadays, almost every browser has a pop-up blocker enabled that can block a link from being opened. Make sure only to invoke the window.open function with a user action (for example, a click).
Final thoughts
As you can see, opening a link in a new tab is simple in React.
If you have a static link use the hyperlink method.
If you need to run JavaScript logic before opening the link, use the window.open function.
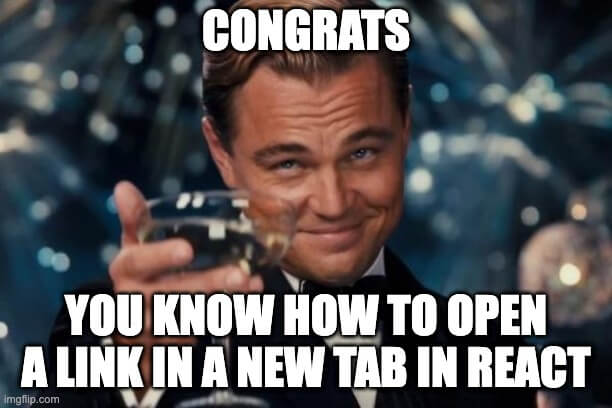
Here are some other React tutorials for you to enjoy: