How To Disable A Button In React?
When working on a React web application, buttons are one of the most common elements a developer will use. Sometimes, a developer may want to enable a button conditionally. This situation brings the question of how to disable a button. Luckily, this is simple to do in React.
To disable a button in React, you need to:
- Toggle the disabled attribute of the button with a state value change.
This article will explore different scenarios of disabling a button in React and show real-life examples.
Let's get to it 😎.
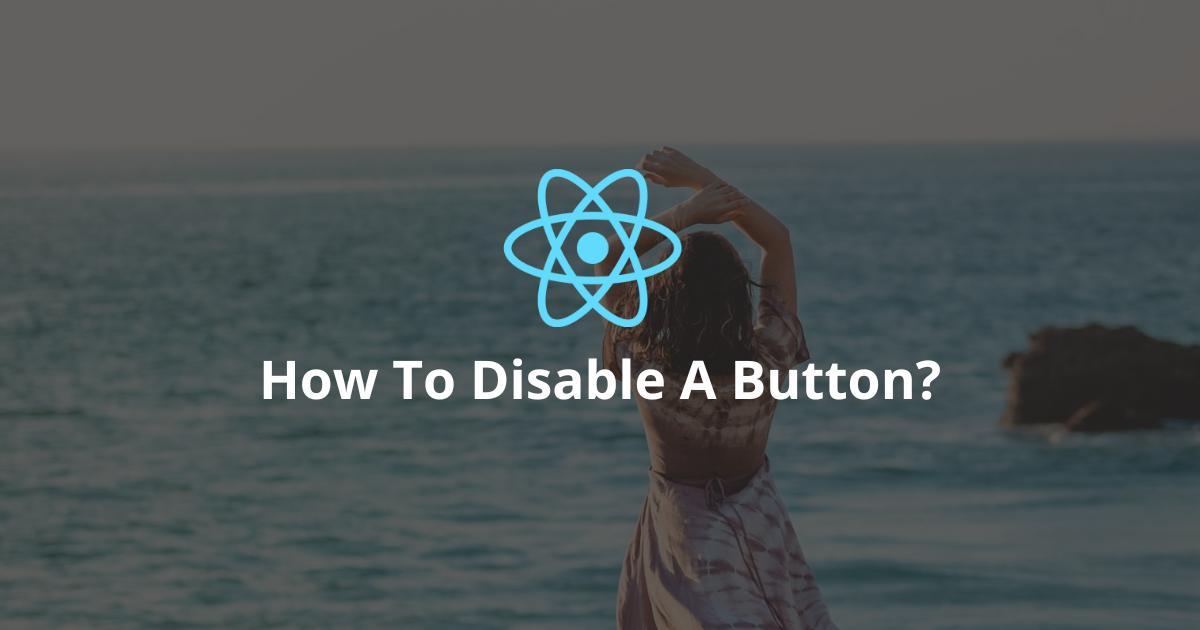
To disable a button in React, pass true to the disabled attribute of the button.
Here is how to do it:
javascriptimport React from "react";
import { createRoot } from "react-dom/client";
const App = () => {
return <button disabled={true}>submit</button>;
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
Note: A user will not be able to use or click a disabled button.
How to disable a button after a click?
To disable a button after a click, you need to:
- Create a state to hold the disabled status of the button.
- Create the click event callback and pass it to the button.
- Inside this callback, set the disabled state to true.
Here is how to do it:
javascriptimport React, { useState } from "react";
import { createRoot } from "react-dom/client";
const App = () => {
const [disabled, setDisabled] = useState(false);
const onClick = () => {
setDisabled(true);
};
return (
<button disabled={disabled} onClick={onClick}>
submit
</button>
);
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
A typical scenario for this example involves using it when submitting a form.
When the user submits the form, we disable the button to prevent multiple submissions.
After the submission ends, we re-enable the button using the same setDisabled function.
How to disable a button on a condition?
To disable a button on a condition, you need to pass a conditional as the disabled value of the button.
Here is an example:
javascriptimport React from "react";
import { createRoot } from "react-dom/client";
const App = ({ isDisabled }) => {
return <button disabled={isDisabled}>submit</button>;
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App isDisabled />);
In this example, the button is disabled only if we pass the isDisabled property as true to the App component.
How to disable a button if an input is empty?
To disable a button if an input is empty, you need to pass the negative value of the input as the disabled value of the button.
Here is how to do it:
javascriptimport React, { useState } from "react";
import { createRoot } from "react-dom/client";
const App = () => {
const [value, setValue] = useState("");
const onInput = (e) => setValue(e.target.value);
return (
<form>
<input value={value} onInput={onInput} />
<button disabled={!value}>submit</button>;
</form>
);
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
As you can see, in this code example, we first create a state that holds the value of the input.
Then, we use that state as the disabled value of the button.
By default, the button is disabled. When the user writes something in the input, the button is enabled.
Note: !value means that if the input's value is empty, the disabled property is true; else, it's false.
Final thoughts
As you can see, disabling a button is no-rocket science in React.
It's the same as disabling a button in HTML.
If you want to do it, simply add a disabled attribute to the button with the value true. And voila!
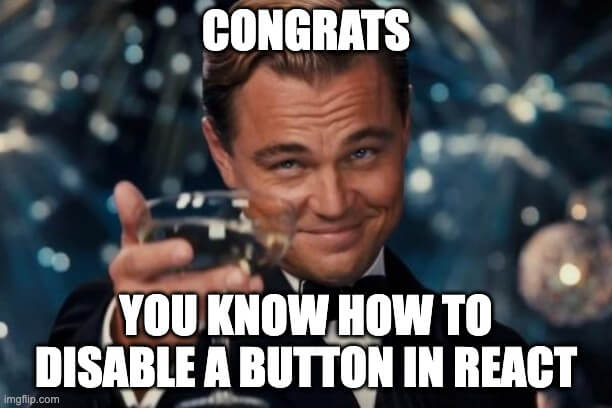
Here are some other React tutorials for you to enjoy: