How To Export A Function In React?
Developers use different files to separate various code pieces when developing a React application. Those files interact by exporting and importing each other's classes, functions, and variables. This brings the question of how to export a function in React.
To export a function in React, you can:
- Use a named export
- Use a default export
This article will analyze those methods and show when to use each one of them.
Let's get to it 😎.
ES6 or ECMAScript 6 provides two main ways of exporting a module from a file:
- using a named export
- using a default export
Method #1 - Export a function as a named export
The easiest way to export a React function is by using a named export.
The main advantage of using a named export is the ability to export multiple modules as a list.
Here is a code example of how to export functions:
javascriptimport React from "react";
// This is named export.
export const Element = () => <div>element</div>;
// This is also a named export.
export const getName = () => "Tim";
If you want to export multiple functions simultaneously, you can export the functions as a list of named exports.
javascriptimport React from "react";
const Element = () => <div>element</div>;
const getName = () => "Tim";
export { Element, getName };
Then, inside another file, you can import those functions, like so:
javascriptimport { Element, getName } from 'src/file';
When importing a named export, use the same name as the module's export name.
Method #2 - Export a function as a default export
Another option to export a function is using the default export.
The most significant differences between a default and a named export are:
- A file can only contain one default export.
- A file can contain multiple named exports.
One file can combine a default export with multiple named exports.
When importing a default export, you can use any name you want.
Here is a code example of a default export:
javascriptimport React from "react";
const Element = () => <div>element</div>;
export default Element;
And here is how you import this function into another file:
javascriptimport Element from 'src/file';
Or use any name you want:
javascriptimport MyElement from 'src/file';
Both imports are going to work.
Note: When importing a default export, you do not use curly braces (as opposed to named exports).
Final thoughts
As you can see, exporting a function in React is simple.
You have two methods of doing it, using a named and a default export.
I recommend using the named export because using the default export can cause refactoring problems in your code. Also, since you can use any name you want when importing a default module, your code may become inconsistent (for example, if many developers work on the same repository).
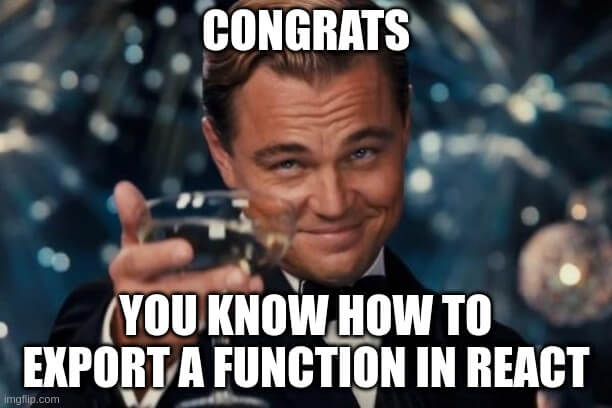
Here are some other React tutorials for you to enjoy: