How To Use The Filter Function In React?
Often when developing a React application, a developer needs to filter data. Luckily, JavaScript offers a simple way to do it.
In React, the easiest way to filter data is to use the built-in JavaScript filter function.
This article will go through everything about this function and show how to use it with common React use cases.
Let's get to it 😎.
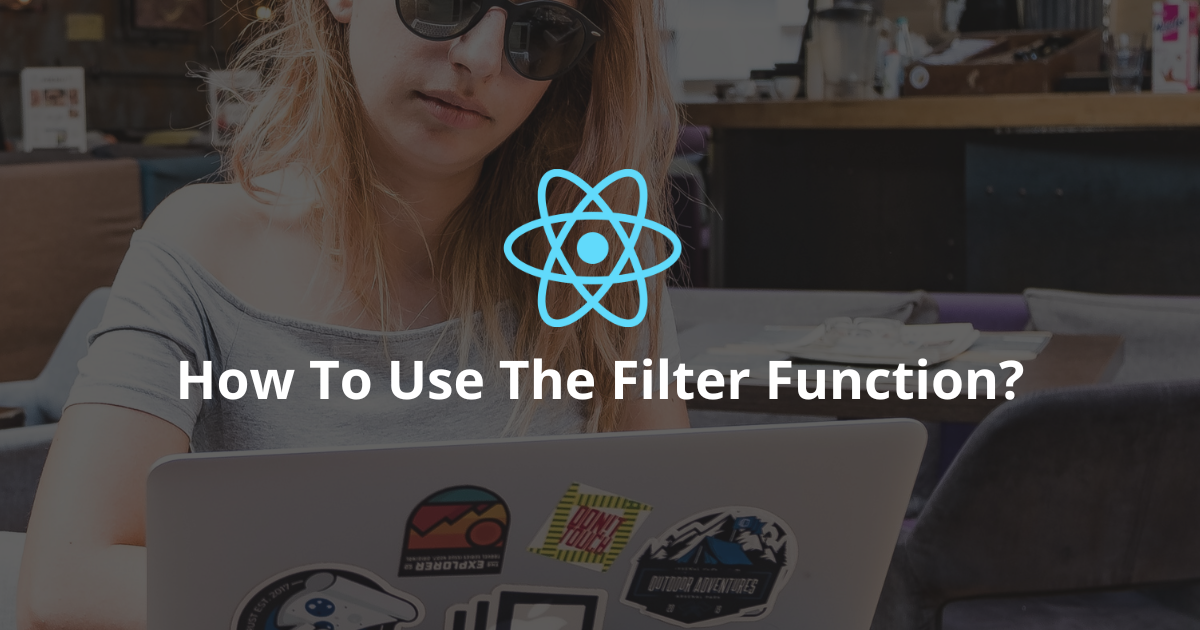
The definition
In JavaScript, the filter function is a built-in array function that creates a shallow copy of an array with elements filtered based on a specific condition. It does not alter the initial array but returns a new one.
The filter function is an ES5 feature, accessible on all modern browsers, including Internet Explorer.
Note: In JavaScript, a shallow copy of an object means that the copied object properties share the same references as the original object's properties.
Here is the syntax of the filter function:
javascriptarray.filter(function(currentValue, index, currentArray), this)
Here are the parameters it accepts:
- A function that runs for each item and returns if the element should be in the final array.
- An optional value for this.
How to filter an array in React?
Since React is a UI library, you can access all the built-in JavaScript functions when using it, so filtering an array involves using the filter function.
Example: You have an array of countries but only want to keep the countries that start with the first letter of the alphabet.
Here is how you can do it:
javascriptimport { createRoot } from "react-dom/client";
const App = () => {
const countries = ["United States", "Argentina", "Canada", "France"];
const filteredCountries = countries.filter(
(country) => country[0].toLowerCase() === "a"
);
return filteredCountries.map((country) => (
<div key={country}>{country}</div>
));
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
First, we filter the array of countries, and we only select the countries that begin with the letter a.
Then, we output divs for each item inside the filtered array using the map function.
Note: You must provide a unique key for each component when using the map function in a React render.
How to filter a list of objects?
The filter function offers you the capability to filter a list of objects.
Example: You have an array of people and only want to keep people older than forty.
Here is how you can do it:
javascriptimport { createRoot } from "react-dom/client";
const App = () => {
const people = [
{
name: "Tim",
age: 27
},
{
name: "Bob",
age: 34
},
{
name: "Jack",
age: 50
}
];
const filteredPeople = people.filter((person) => person.age > 40);
return filteredPeople.map((person) => (
<div key={person.name}>{person.name}</div>
));
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
First, by using the filter function, we create a new array containing people over forty. Then, using the map function, we output their names.
How to filter with multiple conditions?
The filter function also gives you the ability to filter by multiple conditions. Inside the callback that you provide to this function, you can specify how many conditions you want.
Example: You have a list of people and only want to keep people older than 19 with the admin role.
Here is how you can do it:
javascriptimport { createRoot } from "react-dom/client";
const App = () => {
const people = [
{ name: "Tim", age: 20, role: "admin" },
{ name: "Matt", age: 25, role: "user" },
{ name: "Alex", age: 19, role: "user" }
];
const filteredPeople = people.filter((person) => {
return person.age > 19 && person.role === "admin";
});
return filteredPeople.map((person) => (
<div key={person.name}>{person.name}</div>
));
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
In this example, first, we filter the array using the filter function. In its callback, we add all the conditions by which we want to filter. Then, we iterate through the filtered list and output each person's name.
Final thoughts
As you can see, filtering is easy in React.
Alternatively, you can use the Lodash filter function. It has the advantage of working on arrays AND objects.
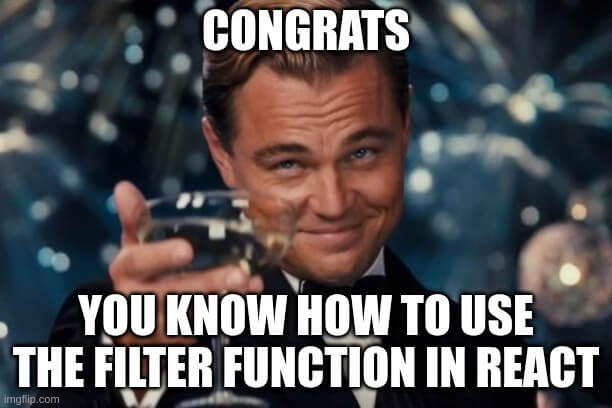
Here are some other React tutorials for you to enjoy: