How Does The Lodash Filter Function Work?
First launched in 2012, Lodash quickly became the most popular JavaScript utility library. Lodash offers many utility functions (for example, the Lodash pick function, the sortBy function, and many more) to help developers solve routine tasks. One such task is being able to filter an array by a specific condition quickly.
The Lodash filter function filters an array by the predicate and returns the result as a new array.
javascriptimport { filter } from "lodash";
const persons = [
{ name: "Tim", age: 20 },
{ name: "Tim", age: 40 },
{ name: "Matt", age: 21 }
];
// Filters out people with age of 30 and more
const filtered = filter(persons, (p) => p.age < 30);
// Outputs: [{name:'Tim",age:20},{name:'Matt',age:21}]
console.log(filtered);
In this article, you will learn how to use this function, the difference between the Array built-in filter function and the Lodash filter function, and many more.
Let’s get to it 😎.
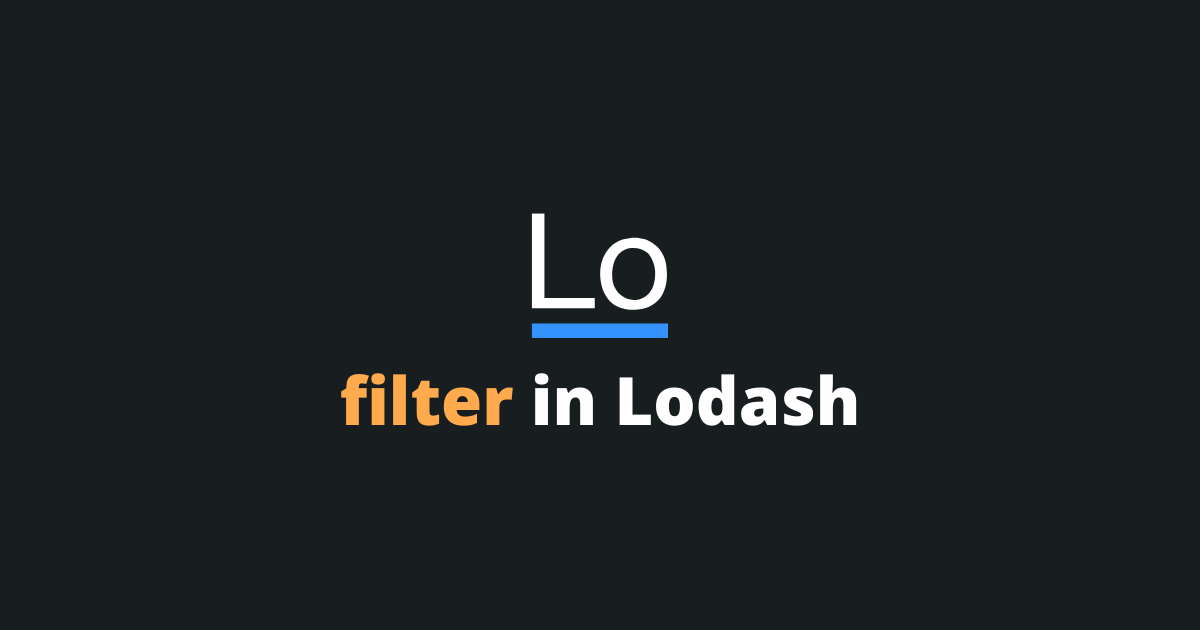
The definition
The Lodash filter function iterates over each element of a collection, checks on each one of them if the predicate returns true, and returns the matched elements inside a new array.
The syntax
javascript_.filter(collection, predicate)
The collection parameter holds the collection (either an array or an object) to iterate over.
The predicate parameter holds the condition to be invoked on each iteration.
How to install the Lodash filter function with npm?
There are two ways to include and use the filter function in your project.
1. If you want to use Lodash's other functions, you can add the whole Lodash library to your project.
javascriptnpm i lodash
2. If you only want to use the filter function, you can add this package.
javascriptnpm i lodash.filter
Then, you can import this function inside your JavaScript or TypeScript file and use it.
How to use the filter function?
Here are a few examples of how to use the filter function.
1. On an array of elements
javascriptimport { filter } from "lodash";
const arr = [10, 3, 7, 2, 9, 15, 2, 4];
const r1 = filter(arr, (v) => v > 5);
// Outputs: [10, 7, 9, 15]
console.log(r1);
2. On an array of objects
javascriptimport { filter } from "lodash";
const arrOfObjects = [
{ name: "Tim", age: 20, role: "admin" },
{ name: "Matt", age: 25, role: "admin" },
{ name: "Alex", age: 19, role: "user" }
];
const r1 = filter(arrOfObjects, (p) => p.age > 20);
// Outputs: [{name:"Matt",age:25,role:"admin"}]
console.log(r1);
const r2 = filter(arrOfObjects, { role: "user" });
// Outputs: [{name:"Alex",age:19,role:"user"}]
console.log(r2);
3. On an object
The filter function also works on an object and returns an array of the matched values.
javascriptimport { filter } from "lodash";
const obj = {
name: "Tim",
age: 20,
role: "admin"
};
const r1 = filter(obj, (v) => v === "Tim");
// Outputs: ['Tim']
console.log(r1);
How to filter by multiple conditions?
To filter by multiple conditions, you must specify the conditions inside the predicate function.
javascriptimport { filter } from "lodash";
const arrOfObjects = [
{ name: "Tim", age: 20, role: "admin" },
{ name: "Matt", age: 25, role: "admin" },
{ name: "Alex", age: 19, role: "user" }
];
const r1 = filter(arrOfObjects, (o) => {
return ["Tim", "Matt"].includes(o.name) && o.age > 20;
});
// Outputs: [{name:"Matt",age:25,role:"admin"}]
console.log(r1);
In this example, we get persons named Matt or Tim, aged 21 and above.
Lodash filter vs. Array filter
The Array filter method is an alternative to the Lodash filter function.
Both functions have pretty much the same behavior.
However, the Array filter function will only work on arrays, not objects.
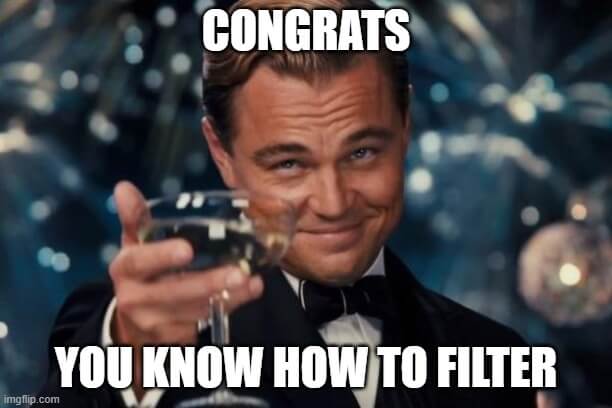
Final thoughts
I use the built-in Array filter function in most cases since it has good browser support and good performance nowadays.
However, if I need to do something complex, I use the filter function in combination with the chain function.