How Does The Lodash SortBy Function Work?
Lodash, first launched in 2012, has quickly become the most popular JavaScript utility library. It provides a lot of very useful functions (for example, the Lodash get function, the find function, etc...) to help developers solve common problems. One of those functions is the sortBy method.
The Lodash sortBy function sorts an array of elements using the iteratees in ascending order.
Here is an example of the sortBy function in action:
javascriptimport { sortBy } from "lodash";
const persons = [
{ name: "Tim" },
{ name: "Alex" },
{ name: "Matt" }
];
const sorted = sortBy(persons, ["name"]);
// Outputs: [{name:"Alex"},{name:"Matt"},{name:"Tim"}]
console.log(sorted);
In this article, I will go over, in detail, everything to know about the sortBy function, as well as answer some of the most common questions.
Let’s get to it 😎.
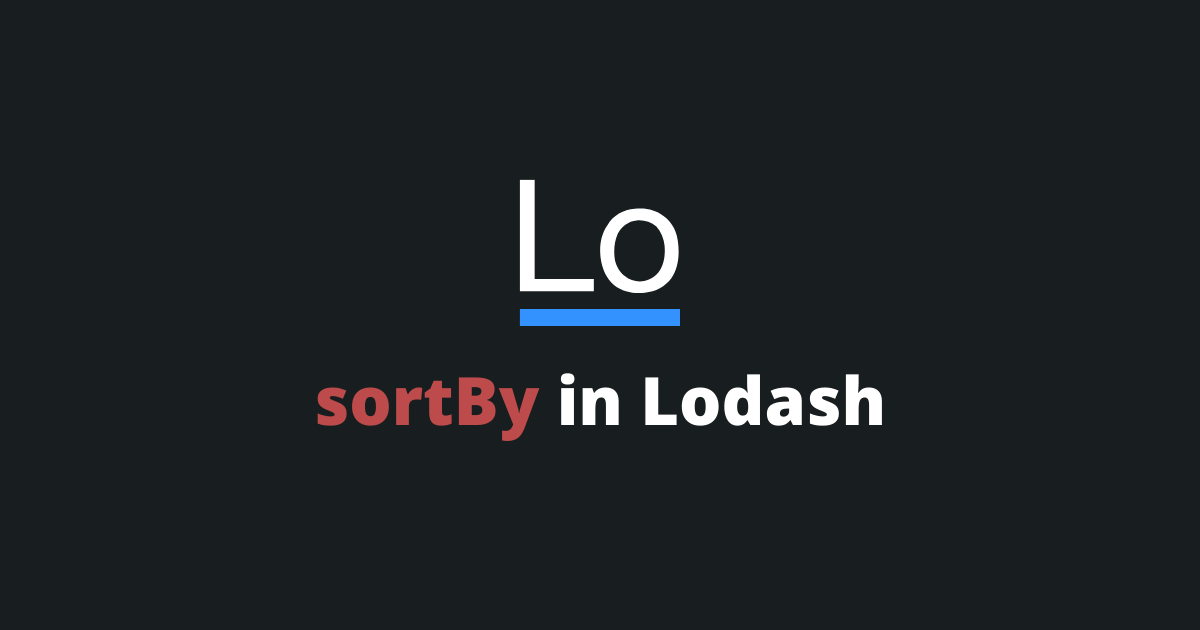
The definition
The Lodash sortBy function returns a new sorted array of elements in ascending order. The array is sorted using the params array.
The syntax
javascript_.sortBy(collection, [params])
The collection parameter holds a collection to iterate over.
The params parameter holds the array of values to sort by. The values can be either of type function or type string.
This method uses a stable sort. This means that if two elements are equal, their original position is preserved.
How to import the sortBy function?
Since Lodash is a very big package and takes a lot of your application's bundle size, my recommendation is to import Lodash functions individually.
Here is how to import the sortBy function using curly braces:
javascriptimport { sortBy } from 'lodash';
Here is another way of importing Lodash functions using the one-by-one method:
javascriptimport sortBy from 'lodash/sortBy';
The one-by-one method will result in the smallest bundle size.
How to use the sortBy function?
Here are a few examples of how to use this function.
javascriptimport { sortBy } from "lodash";
const persons = [
{ name: "Tim", age: 20 },
{ name: "Tim", age: 40 },
{ name: "Matt", age: 21 }
];
// Sorts the persons by name ASC
const sorted = sortBy(persons, ['name']);
// Sorts the persons by age ASC
const sorted2 = sortBy(persons, (p) => p.age);
How to sort by multiple fields?
To sort an array of elements in Lodash by multiple fields, you just need to add all of those fields in the params array.
Here is an example of this:
javascriptimport { sortBy } from "lodash";
const persons = [
{ name: "Tim", age: 40 },
{ name: "Tim", age: 20 },
{ name: "Matt", age: 21 }
];
const sorted = sortBy(persons, ['name','age']);
// Outputs: [
// {name:"Matt",age:21},
// {name:"Tim",age:20},
// {name:"Tim",age:40},
// ]
console.log(sorted);
How to sort in descending order?
To sort an array of elements in Lodash in descending order you need to use the Lodash orderBy function.
Here is an example of this:
javascriptimport { orderBy } from "lodash";
const persons = [
{ name: "Tim" },
{ name: "Alex" },
{ name: "Matt" }
];
const sorted = orderBy(persons, ['name'], ['desc']);
// Outputs: [{name:"Tim"},{name:"Matt"},{name:"Alex"}]
console.log(sorted);
Final thoughts
As you can see the sortBy function is very straight and forward and does exactly what its name sounds like.
For my part, I prefer using the equivalent orderBy function because it gives the ability to specify the sort order. It is a very useful ability to have, especially when sorting by multiple fields.
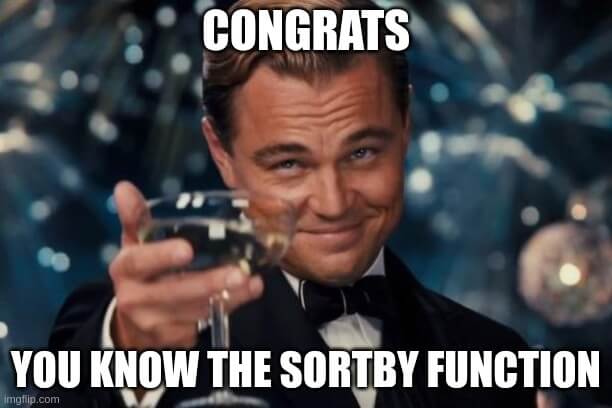
I have written more tutorials on Lodash on this website (if you are interested in learning more).
Don’t hesitate to ask your questions about Lodash in the comments section. I always check for new comments and answer/respond to them.
Thank you very much for reading this article.
Please share it with your colleagues and fellow developers.