How To Use The Lodash OrderBy Function?
Lodash is a utility library written in JavaScript that helps developers work with strings, numbers, arrays, and other types of objects. It provides a lot of utility functions (for example, the Lodash filter function or the pick function) to solve common tasks. One such function is the orderBy function.
The Lodash orderBy function sorts an array of objects according to the specified params and sort orders.
javascriptimport { orderBy } from "lodash";
const arr = [
{ name: "Matvei" },
{ name: "Oleg" },
{ name: "Anton" }
];
const sorted = orderBy(arr, ["name"], ["asc"]);
// Outputs: [{name:"Anton"},{name:"Matvei"},{name:"Oleg"}]
console.log(sorted);
By the end of this article, you will know how to sort an array in Lodash, how to specify the sort order, and many more.
Let’s get to it 😎.
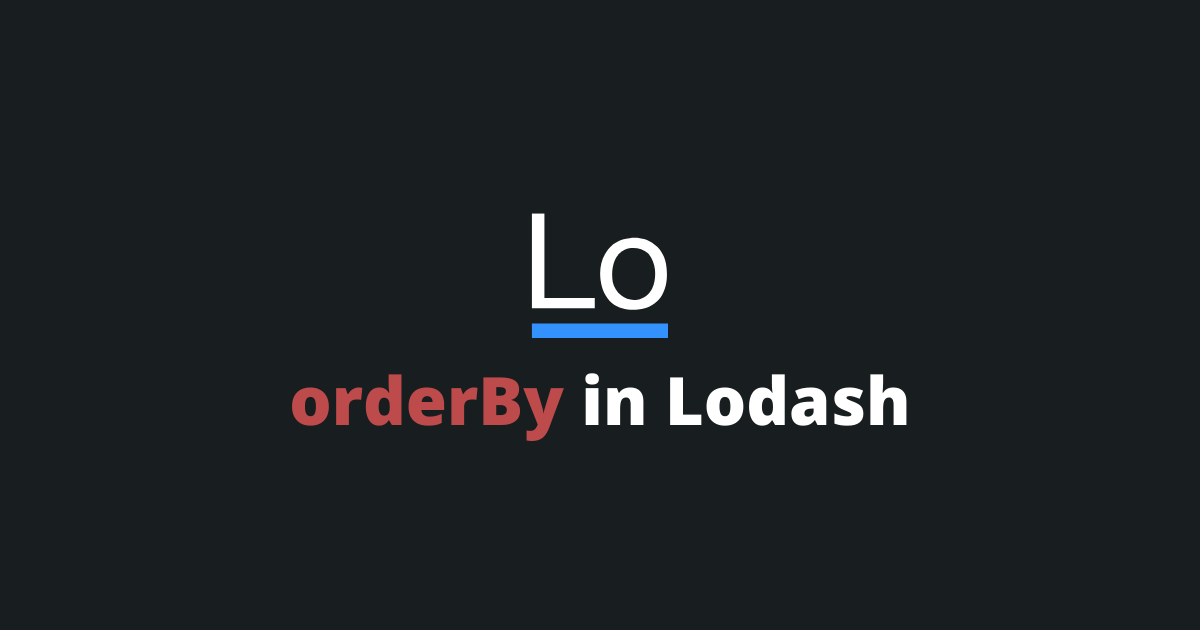
The definition
The Lodash orderBy function sorts an array of elements according to the specified params and orders.
The orderBy function has the same behavior as the Lodash sortBy function but permits specifying the sort orders.
Notice: The values will be sorted in ascending order if no sort orders are specified.
The syntax
javascript_.orderBy(collection, params, orders)
The collection parameter holds a collection of elements to iterate over.
The params parameter holds an array of values (function or string) to sort by.
The orders parameter holds an array of sort orders ('asc' or 'desc').
Notice: Both the params and the orders arrays should be the same length.
How to install the Lodash orderBy function with npm?
There are two ways to add the orderBy function to your project.
1. You can add the whole Lodash library to your project.
javascriptnpm i lodash
2. If you only want to use the orderBy function, you can install this package.
javascriptnpm i lodash.orderby
Then, you can import this function inside your TypeScript or JavaScript file.
How to use the orderBy function?
Here are a few examples of the orderBy function in action:
javascriptimport { orderBy } from "lodash";
const persons = [
{ name: "M", age: 28 },
{ name: "A", age: 45 },
{ name: "Z", age: 21 }
];
// Sorts the persons by name DESC
const sorted = orderBy(persons, ["name"], ["desc"]);
// [{name:"Z",age:21},{name:"M",age:28},{name:"A",age:45}]
console.log(sorted);
// Sorts the persons by age DESC
const sorted2 = orderBy(persons, (p) => p.age, ["desc"]);
// [{name:"A",age:45},{name:"M",age:28},{name:"Z",age:21}]
console.log(sorted2);
How to sort an array by dates?
To perform a sort on an array of elements with string dates, you can use the Date built-in object to cast the strings into dates in combination with the Lodash orderBy function.
javascriptimport { orderBy } from "lodash";
const arr = [
{ date: "2022-05-03" },
{ date: "2021-05-01" },
{ date: "2022-09-01" }
];
const res = orderBy(arr, [(o) => new Date(o.date)], ["desc"]);
// [{date:"2022-09-01"},{date:"2022-05-03"},{date:"2021-05-01"}]
console.log(res);
How to orderBy case insensitive?
To perform a case insensitive sort on an array of elements, you can use the JavaScript toLowerCase function in combination with the Lodash orderBy function.
javascriptimport { orderBy } from "lodash";
const arr = [
{ name: "Matvei" },
{ name: "matvei" },
{ name: "Matt" }
];
const sorted = orderBy(arr, [(u) => u.name.toLowerCase()]);
// Outputs: [{name:"Matt"},{name:"Matvei"},{name:"matvei"}]
console.log(sorted);
Lodash orderBy vs sortBy
Both functions have the same behavior. The only difference between them is that the orderBy function allows specifying the sort orders.
I recommend using the orderBy function because sortBy will be removed in the fifth version of Lodash.
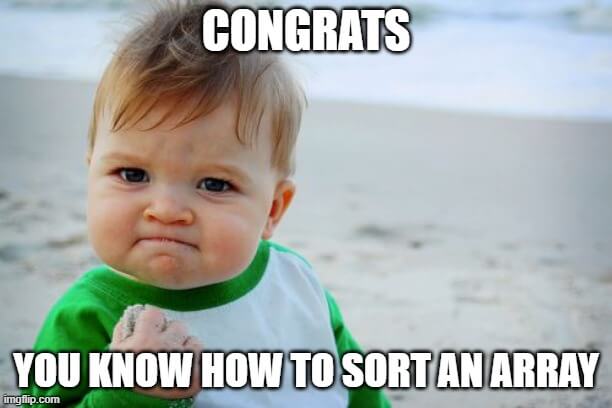
Final thoughts
As you can see, orderBy is a very handy function that makes sorting very easy and painless.
I use this function all the time in my projects.