How Does The Lodash Pick Function Work?
Lodash is a popular JavaScript utility library that offers a lot of handy functions (for example, the Lodash sortBy function or the get function) to help developers work with arrays, strings, numbers, and other types. One of Lodash's most valuable functions is the pick function.
The Lodash pick function creates a new object composed of the picked properties.
javascriptimport { pick } from 'lodash';
const person = {
firstName: 'Tim',
lastName: 'Mousk',
year: 1995,
};
// Outputs: { firstName: "Tim", lastName: "Mousk" }
console.log(pick(person, ['firstName', 'lastName']));
When you will finish reading this article you will know everything about the pick function, know how to pick from a nested object, understand alternatives, and many more.
Let’s get to it 🙃.
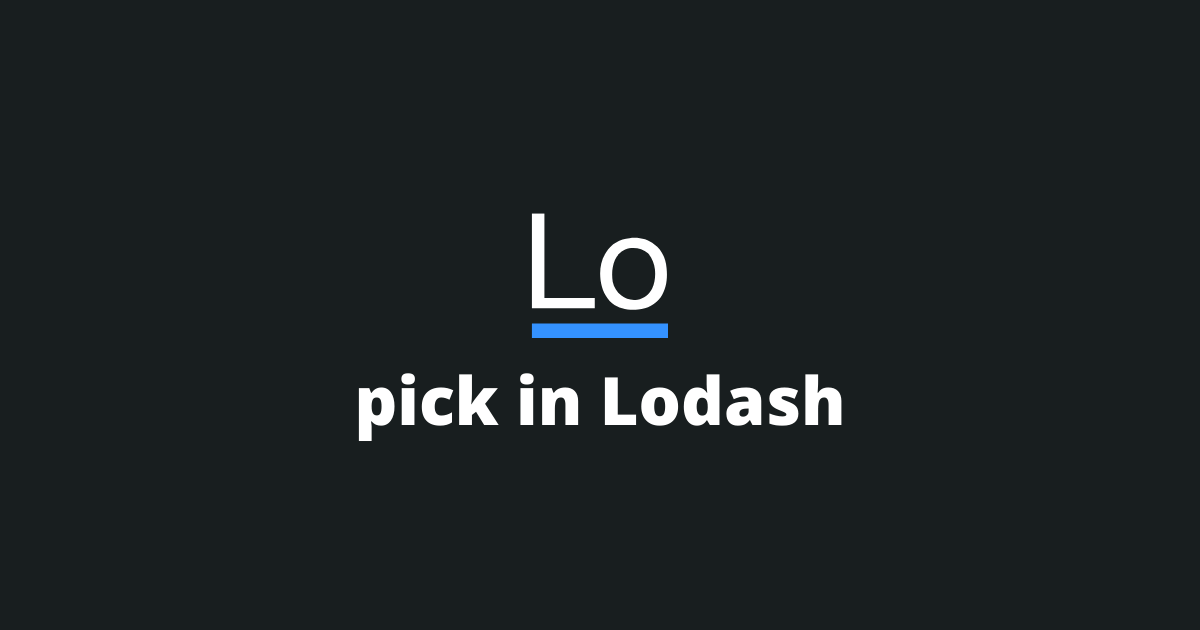
The definition
The Lodash pick function returns a new object with the picked properties.
The syntax
javascript_.pick(object, [paths])
The object parameter holds the source object.
The paths parameter holds an array of paths that represent properties to pick.
If a path does not correspond to a property it is ignored in the final object.
How to install Lodash pick with npm?
You have two ways of installing and using this function.
1. Install the whole Lodash package:
bashnpm i lodash
2. Install a package that only contains the pick method:
bashnpm i lodash.pick
How to use the pick function?
Here are a few examples of how to use this function.
javascriptimport { pick } from "lodash";
const person = {
year: 1995,
name: {
firstName: "Tim",
lastName: "Mousk"
},
status: true
};
const picked = pick(person, ["year", "status"]);
// Outputs: { year: 1995, status: true }
console.log(picked);
// pick will ignore unfound paths.
const picked2 = pick(person, ["name.firstName", "not_found"]);
// Outputs: { name: { firstName: 'Tim' }}
console.log(picked2);
How to pick from a nested object?
You can pick nested properties using the dotted paths notation.
javascriptimport { pick } from "lodash";
const person = {
a: {
b: 1,
c: 2
}
};
const picked = pick(person, ["a.b"]);
// Outputs: { a: { b: 1 } }
console.log(picked);
Is there an alternative to the pick function?
Yes, you can use the ES6 destructuring feature to get approximately the same behavior.
javascriptconst person = {
year: 1995,
name: {
firstName: "Tim",
lastName: "Mousk"
},
status: true
};
const { year, status } = person;
// Outputs: { year: 1995, status: true }
console.log({ year, status });
Final thoughts
Here you have it.
As you can see, the Lodash pick function gives the developer a convenient way of creating a new object from chosen properties, and it even works with nested properties.
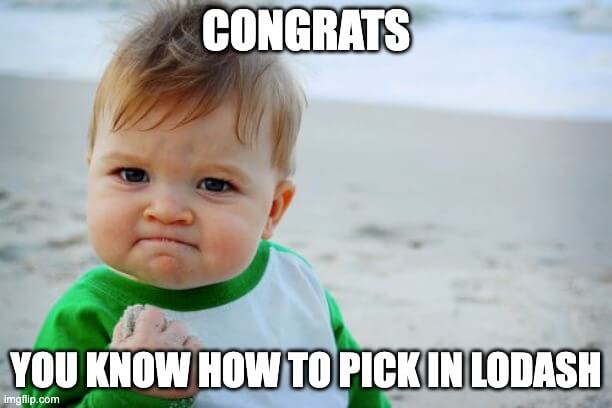
I have written more tutorials on Lodash on this website (if you are interested in learning more).
Thank you very much for reading this article.
Please share it with your fellow developers and colleagues.
Don’t hesitate to ask your questions about Lodash in the comments section. I always check for new comments and answer/respond to them.