How To Add The For Attribute On A Label In React?
In HTML, the label element defines a caption for an input. We use the "for" attribute to associate a label to a form input. However, if you try to add the "for" attribute on a label when using React, you may be surprised that React ignores this attribute.
In React, you need to use the "htmlFor" attribute instead of the "for" attribute.
In this article, I will go through the complete solution to add this attribute to a label with a React code example.
Let's get to it 😎.
Why use the "for" attribute in HTML?
When used on a label, the "for" attribute allows the user to link a label to a control element.
It means that:
- When a label receives a focus, it passes this focus to the associated input.
- The web page is easier to read by a speech-based user agent.
We need to pass the input id as the value for the "for" attribute.
Here is an example:
xml<label for="input-id">Label</label>
<input type="text" name="input-name" id="input-id">
In this case, the text input gets focused when the user clicks on the label.
Why is React ignoring the "for" attribute?
When React implemented its DOM implementation, it took the time to clean up some of DOM's issues for better performance and browser compatibility.
The "for" keyword is a reserved word in JavaScript.
That's why in React, it becomes the "htmlFor" attribute.
In newer versions of React, we may get this error: "Warning: Invalid DOM property `for`. Did you mean `htmlFor`?"
How to add the "for" attribute in React?
In React, since we are using JSX, we need to use the "htmlFor" attribute.
Here is a code example:
javascriptimport { createRoot } from "react-dom/client";
const App = () => (
<>
<label htmlFor="my-name">My Name:</label>
<input name="name" id="my-name" type="text" />
</>
);
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
We have successfully linked a label to an input in React.
Final thoughts
As you can see, adding the "for" attribute to a React label is easy.
You just need to use its JSX equivalent htmlFor.
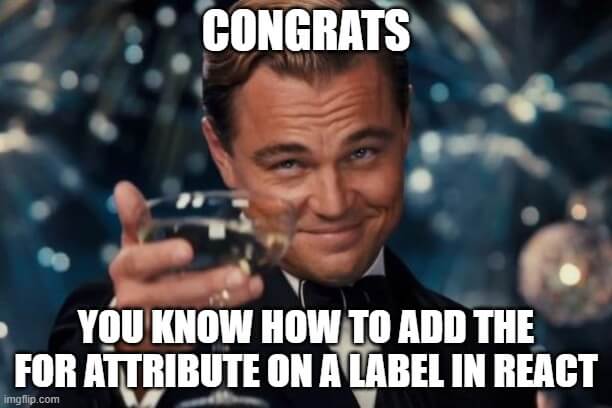
Here are some other React tutorials for you to enjoy: