How To Import JSON In React?
When creating a React web application or library, often a developer needs to import JSON data. A typical example is having a JSON file with country names used by the React web application. Luckily, it is easy to achieve.
To import JSON in React, you can:
- Use the json-loader plugin.
- Create a JavaScript/TypeScript constant with the JSON data.
This article will analyze both solutions and show code examples for each.
Let's get to it 😎.
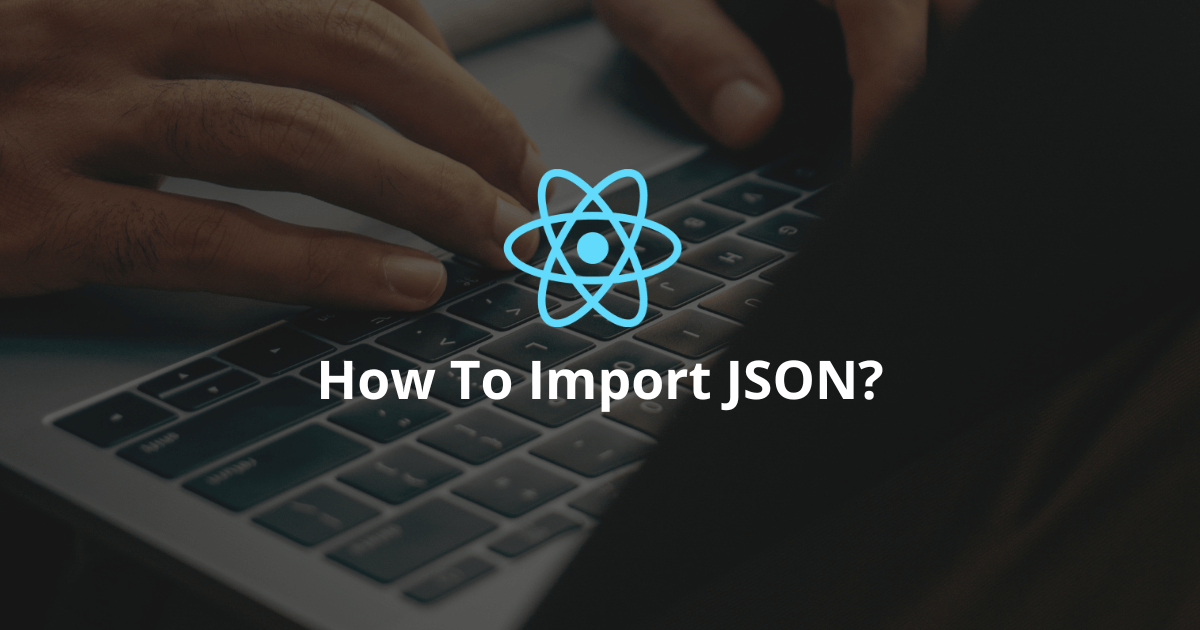
Let's say we have a JSON file with some data:
data.json[{ "country": "US" }, { "country": "CA" }]
We want to import this file into our React application and use it.
We have two different methods of achieving it.
Method #1 - Use the json-loader plugin
The first method of importing JSON data into your React application involves using the json-loader plugin.
Here is how to use it for various use cases.
1. If you are using create-react-app
In that case, the json-loader plugin is installed by default.
The only thing to do is to import the JSON file like so:
javascriptimport data from "/data.json";
Then, you can use the JSON data in your code.
2. If you are NOT using create-react-app
Here are the steps to follow:
1. Install the json-loader plugin, like so:
bashnpm i json-loader --save-dev
2. Add this loader configuration to your webpack.config.js file:
javascriptloaders: [
{
json: /\.json$/,
loader: 'json-loader'
}
]
3. In your application, import the JSON data, like so:
javascriptimport data from "/data.json";
Method #2 - Create a constant with the JSON data
Another option to import JSON in a React application is to transform the JSON file into a JS/TS file with a constant export.
This method is beneficial because you do not have to install external plugins.
Here is how to do it:
1. Let's say you have a JSON file, like so:
data.json[{ "country": "US" }, { "country": "CA" }]
2. You need to transform it into a JS file.
javascriptexport const data = [{ country: "US" }, { country: "CA" }];
3. Finally, import it into your React application, like so:
javascriptimport { data } from "/data";
Here is a small example:
javascriptimport React from "react";
import { createRoot } from "react-dom/client";
import { data } from "/data";
const App = () => {
return (
<div>
{data.map((x) => (
<div key={x.country}>{x.country}</div>
))}
</div>
);
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
This code maps through an array of countries and outputs a div for each.
Final thoughts
In conclusion, importing a JSON file is simple in React.
If you have a small JSON object, I recommend changing it to a JS constant.
However, if the JSON file is large, I recommend using the json-loader plugin.
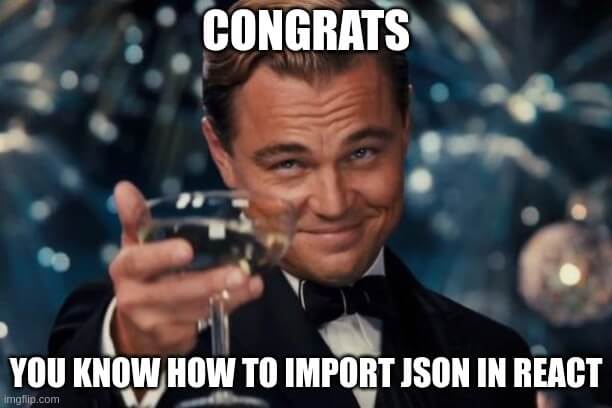
Here are some other React tutorials for you to enjoy: