How To Set Focus On An Input In React?
When you create a form in your React web application, often a developer wants to set focus on the first input. Think of any sign-in or sign-up form that you see on the Internet. Luckily, this is very simple to accomplish in React.
To set focus on an input in React, you can:
- Use a React reference with a useEffect hook.
- Use the autoFocus attribute on the input.
In this article, I will explain both solutions and show how to code them with real-life examples.
Let's get to it 😎.
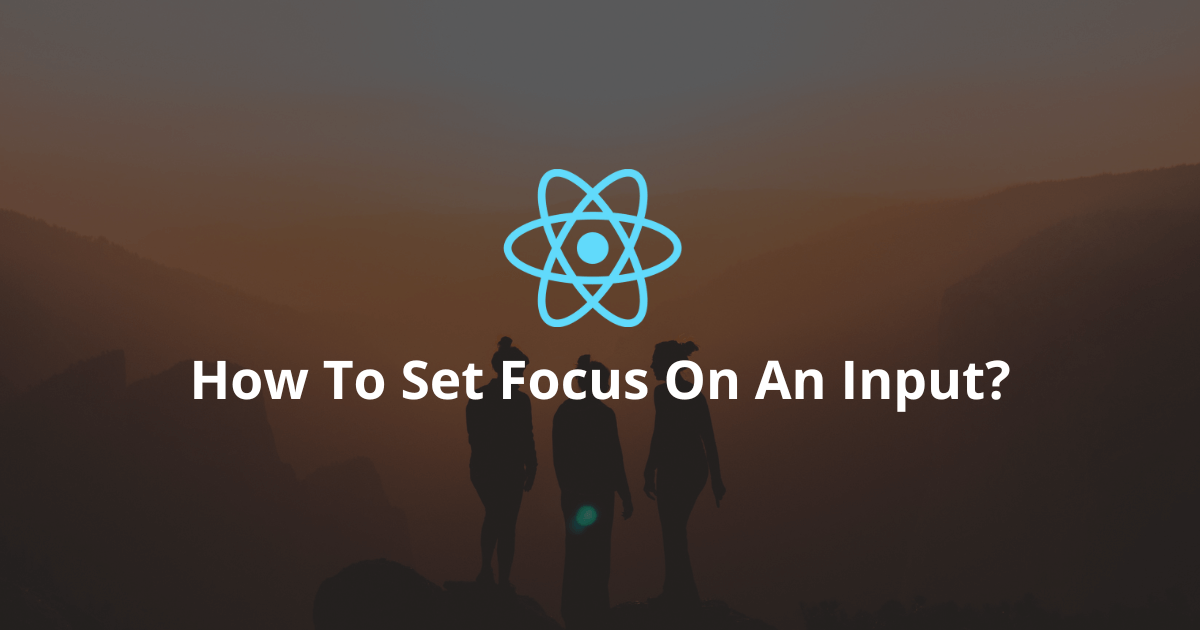
Method #1 - Set focus using a reference
One method to set focus on an input involves using a React reference.
Here is the process to follow:
- You create a React reference using the useRef hook and pass it to the input.
- When you need to focus the input, you call the focus function of the input's reference using its current property.
Here is an example of it:
javascriptimport { useEffect, useRef } from "react";
import { createRoot } from "react-dom/client";
const App = () => {
const ref = useRef(null);
useEffect(() => {
ref.current.focus();
}, []);
return <input type="text" ref={ref} />;
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
This example auto-focuses the input.
Note: We call the focus method inside the useEffect hook to ensure that the input element has been rendered.
Method #2 - Set focus using the autoFocus attribute
Another way to set focus on an input involves adding the autoFocus attribute to the input.
Here is how to do it:
javascriptimport { createRoot } from "react-dom/client";
const App = () => {
return <input type="text" autoFocus />;
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
In this example, React auto-focuses the input that it renders.
Note: If you do not capitalize the F when writing this attribute, React will not set focus on the input.
How to focus an input on click?
To focus an input on click, you need to create a React reference to the input and call the focus function inside the click callback.
Here is an example of it:
javascriptimport { useRef } from "react";
import { createRoot } from "react-dom/client";
const App = () => {
const ref = useRef(null);
const handleClick = () => {
ref.current.focus();
};
return (
<>
<button onClick={handleClick}>focus</button>
<input type="text" ref={ref} />
</>
);
};
const rootElement = document.getElementById("root");
const root = createRoot(rootElement);
root.render(<App />);
In this example, the input gets focused when the user clicks the button.
Final thoughts
As you can see, focusing an input is easy in React.
If you want to set the focus on an input conditionally or when an event happens, use the reference method.
Else, add the autoFocus attribute to the input.
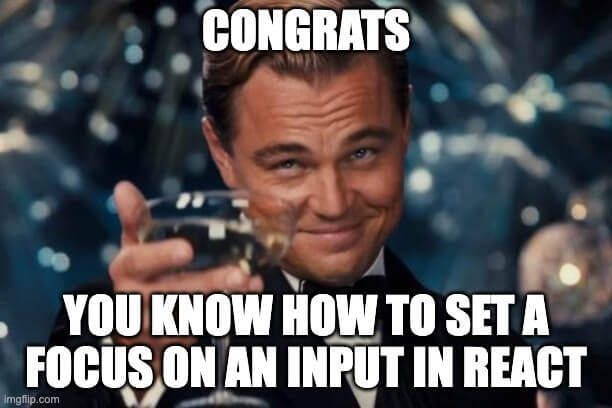
Here are some other React tutorials for you to enjoy: