How To Mock A React Component In Jest?
When it comes to React development, testing your code is almost as important as writing it. One of the best libraries for this task is Jest (developed by Facebook). When testing a piece of React code, a developer often needs to mock a component. But how do you do it in Jest?
This article explains mocking React components in Jest and shows multiple code examples.
Let's get to it 😎.
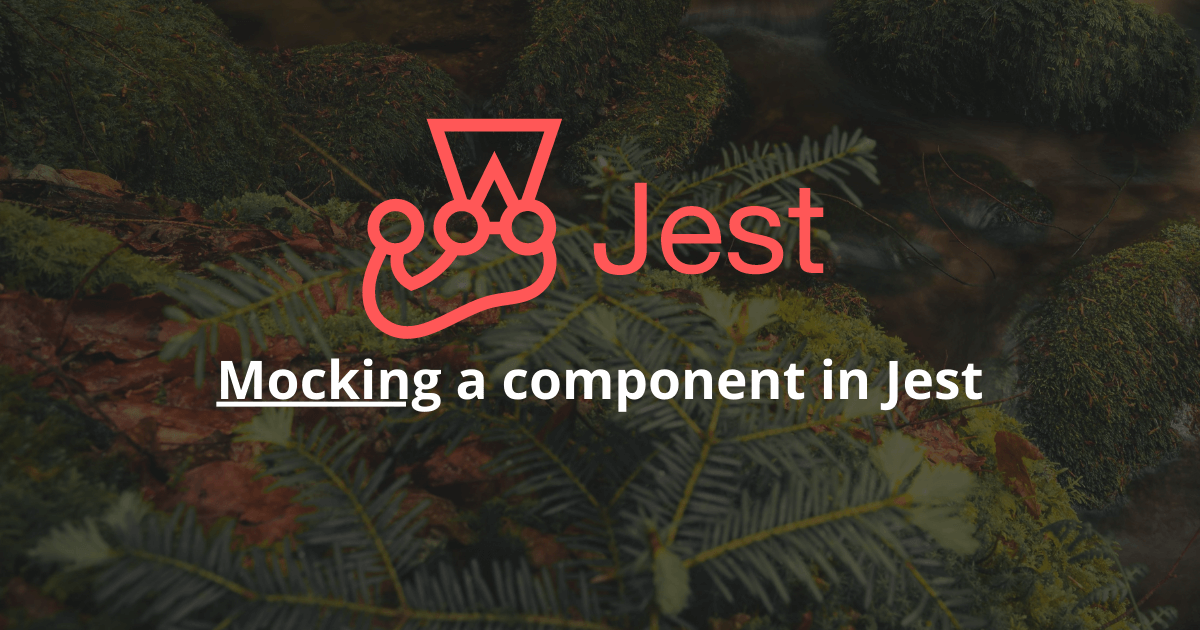
How to mock a component?
The easiest way to mock a React component is to use the jest.mock function.
However, the mock implementation is going to be different, depending on whether the component you want to mock is:
- A default export
- A named export
Mocking a React component (default export)
First, let's set up an example to understand mocking a React component exported via a default export.
javascriptimport React from "react";
import Modal from "./modal";
const Container = ({ open }) => <>{open && <Modal />}</>;
export default Container;
In this example, how do you mock the Modal component?
Here is how:
javascriptjest.mock("./modal", () => () => {
return <mock-modal data-testid="modal" />;
});
Easy right? The jest.mock function accepts two arguments, a component's path and a callback with the mock. The path must match the exact location of the component.
Here is the complete code with tests:
javascriptimport React from "react";
import { render } from "@testing-library/react";
import Container from "./container";
jest.mock("./modal", () => () => {
return <mock-modal data-testid="modal" />;
});
test("renders the Modal when Container is open.", () => {
const { queryByTestId } = render(<Container open />);
expect(queryByTestId("modal")).toBe(true);
});
test("doesn't render the Modal when Container is closed.", () => {
const { queryByTestId } = render(<Container />);
expect(queryByTestId("modal")).toBe(false);
});
As you can see, first, we mock the Modal component with a fake component that has a test id. Then, within our unit tests, we can query the mocked component using the test id.
Cool right?
Mocking a React component (named export)
Now let's see how to mock a React component exported via a named export.
First, let's adjust the above example to export the Modal component via a named export.
javascriptimport React from "react";
import { Modal } from "./modal";
const Container = ({ open }) => <>{open && <Modal />}</>;
export default Container;
To mock the Modal we once again need to use the jest.mock function.
Here is the full code:
javascriptimport React from "react";
import { render } from "@testing-library/react";
import Container from "./container";
jest.mock("./modal", () => ({
Modal: () => {
return <mock-modal data-testid="modal"/>;
},
}));
test("renders the Modal when Container is open.", () => {
const { queryByTestId } = render(<Container open />);
expect(queryByTestId("modal")).toBe(true);
});
test("doesn't render the Modal when Container is closed.", () => {
const { queryByTestId } = render(<Container />);
expect(queryByTestId("modal")).toBe(false);
});
As you can see, the jest.mock callback is the only thing that changed!
Note: If the mocked component is an ES6 module you need to set the __esModule property to true inside the jest.mock callback return object.
Final thoughts
As you can see, mocking a React component using Jest is easy!
Even though testing is not the most fun thing, be sure to always test your React application, because it will save you a lot of bugs and debugging time in the long run.
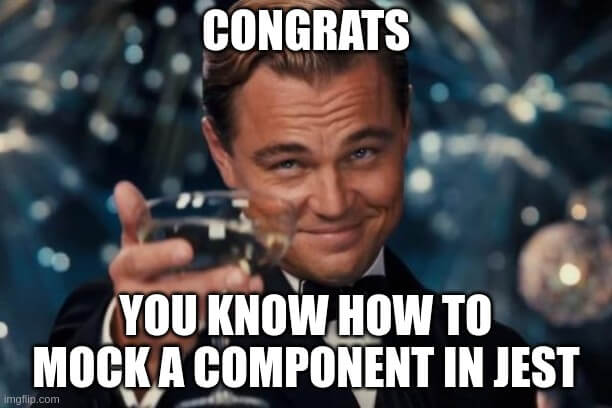
Here are some other React tutorials for you to enjoy: