How does typeof work in TypeScript?
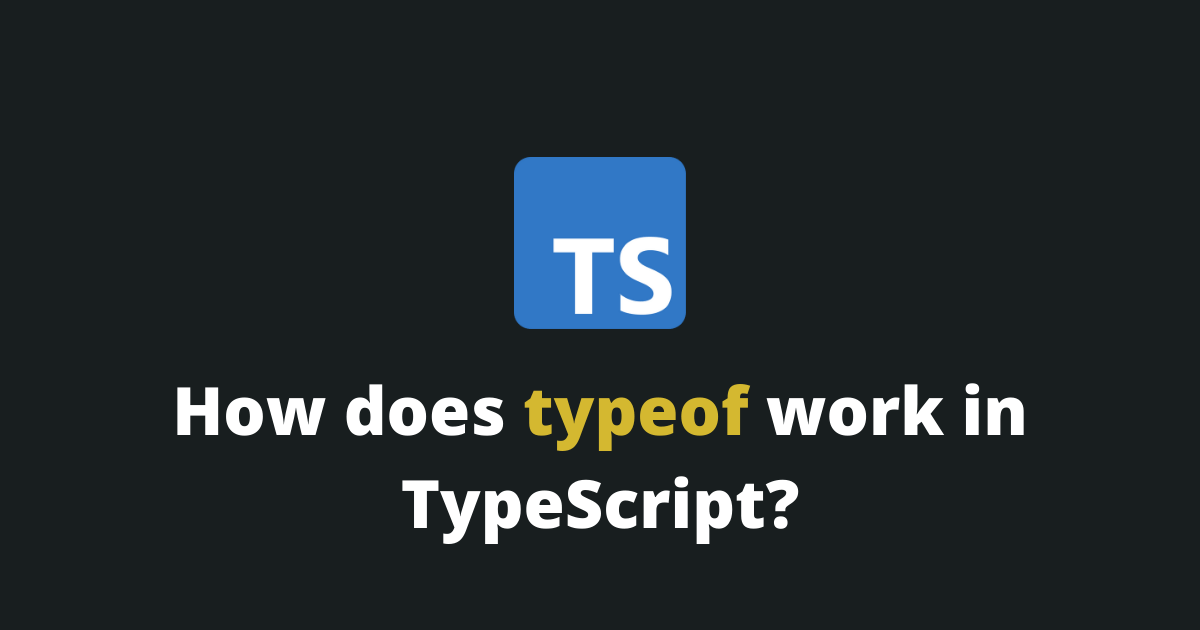
TypeScript just like JavaScript has a special typeof operator.
In TypeScript, the typeof operator is used to refer to the type of a variable in a type context.
javascriptconst v = 'This is a string';
const data: typeof v;
In that case, the type of data is string.
Maybe you have noticed, but, in this particular example, this is not very useful, since the type is a basic type... You are right. But, the typeof operator becomes very handy with complex objects.
typescriptconst person = {
age: 26,
name: 'Tim',
subList: {
element1: true
}
};
type Data = typeof person;
In that case, the Data type will have this structure:
typescripttype Data = {
age: number;
name: string;
subList: {
element1: boolean;
};
};
In that example, we create a type based on the structure of the person variable, that we can later use to match other variables.
P.S. You can only use the typeof operator on variable names or their properties.
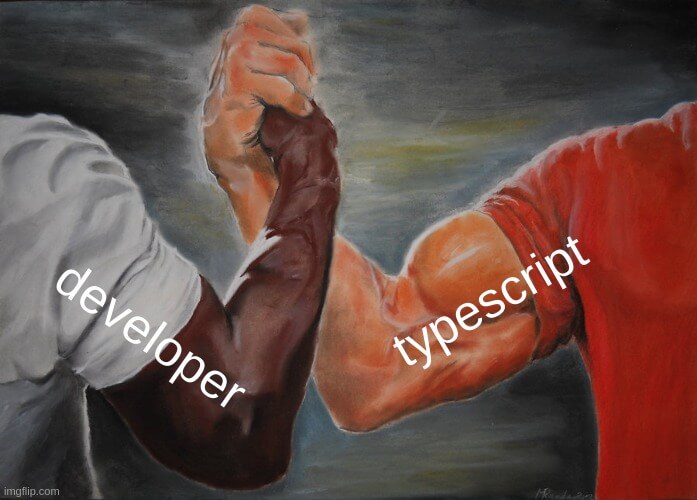
How to get the type from elements of an array?
In TypeScript, you can transform elements from an array into a union type using the const keyword.
typescriptconst animals = [ 'dog', 'cat', 'elephant' ] as const;
type Data = typeof animals[number];
In that case, the Data type will have this structure:
typescripttype Data = 'dog' | 'cat' | 'elephant';
This also work on an array of objects!
typescriptconst animals = [
{ age: 3, type: 'dog' },
{ age: 4, type: 'cat' }
];
type Data = (typeof animals)[number];
In that case, the Data type will have this structure:
typescripttype Data = {
age: number;
type: string;
};
How does keyof typeof work in TypeScript?
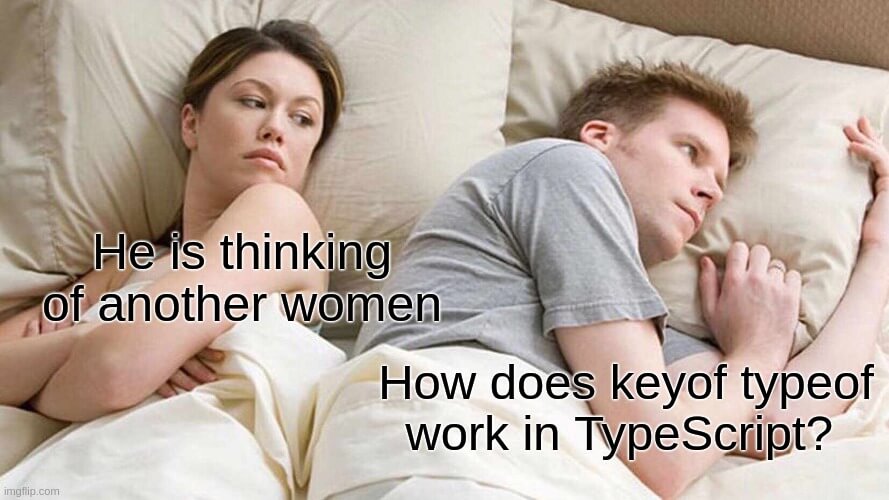
In TypeScript, the combination of the keyof operator with the typeof operator is used to get a union type of an object's keys.
typescriptconst person = {
age: 26,
name: 'Tim'
};
type Data = keyof typeof person;
In that case, the Data type will have this structure:
typescripttype Data = 'age' | 'name';
This also works on an enum!
typescriptenum Animal {
cat,
dog,
cow
}
type Data = keyof typeof Animal;
In that case, the Data type will have this structure:
typescripttype Data = 'cat' | 'dog' | 'cow';
How to get the return type of a function?
In TypeScript, you can get the return type of a function using the ReturnType predefined type, introduced in TypeScript v2.8. ReturnType will take a function type and return its return type.
typescriptconst createPerson = () => ({
age: 26,
firstName: 'Tim'
});
type Data = ReturnType<typeof createPerson>
In this example, the Data type will have this structure:
typescripttype Data = {
age: number;
firstName: string;
};
Final Thoughts
As a TypeScript developer, I use the typeof operator a lot. It is particularly useful when you need to type-check a parameter based on an object's structure or when you need to extract keys from an object as a type.
I hope you liked this article, please share it!