How To Use The JavaScript Power Operator?
JavaScript supports many different operators (for example, the concatenation operator) to help developers work with objects. One of those operators is the JavaScript power operator. Developers have two distinct ways of using the power operation.
Firstly, you can use the exponentiation operator.
javascript// Outputs: 625
console.log(5 ** 4);
Secondly, you can use the built-in Math.pow function.
javascript// Outputs: 625
console.log(Math.pow(5, 4));
In this article, we will go over the power operation, the exponentiation operator, the Math.pow function, and many more.
Let's get to it 😎.
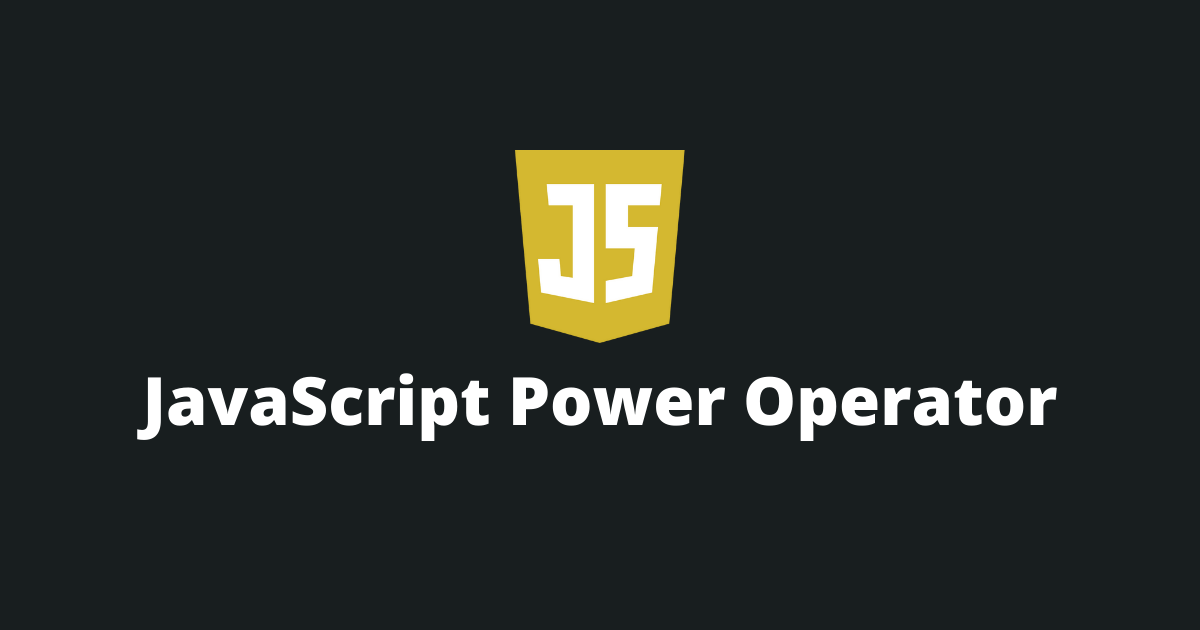
What is the power operation?
The power operation accepts two operands and returns the result of the first operand power the second operand.
The is the math syntax for this operation: xy
The exponentiation operator
The exponentiation operator raises the base argument to the power of the exponent argument. Two stars (**) represent the exponentiation operator.
Browser support
This is an ECMAScript2016 feature, so only new browsers will support it.
Examples
Here is the exponentiation operator in action:
javascript// Outputs: 8
console.log(2 ** 3);
// Outputs: 16
console.log(4 ** 2);
// Outputs: NaN
console.log(5 ** NaN);
// Outputs: 16
console.log((-2) ** 4);
// Outputs: 0.1111111111111111
console.log(3 ** -2);
The exponentiation assignment
You can also use the exponentiation operator as an assignment statement.
javascriptlet x = 4;
x **= 2;
// Outputs: 16
console.log(x);
The Math.pow function
This function returns the result of the first operand to the power of the second operand.
The syntax
javascriptMath.pow(x, y)
The x parameter is required and holds the base.
The y parameter is also required and holds the exponent.
Browser support
All major browsers support the Math.pow function 🥳 because it is an ECMAScript1 feature (1997).
Examples
Here is the Math.pow function in action:
javascript// Outputs: 8
console.log(Math.pow(2, 3));
// Outputs: 16
console.log(Math.pow(4, 2));
// Outputs: NaN
console.log(Math.pow(5, NaN));
// Outputs: 16
console.log(Math.pow(-2, 4));
// Outputs: 0.1111111111111111
console.log(Math.pow(3, -2));
The difference between the exponentiation operator vs Math.pow
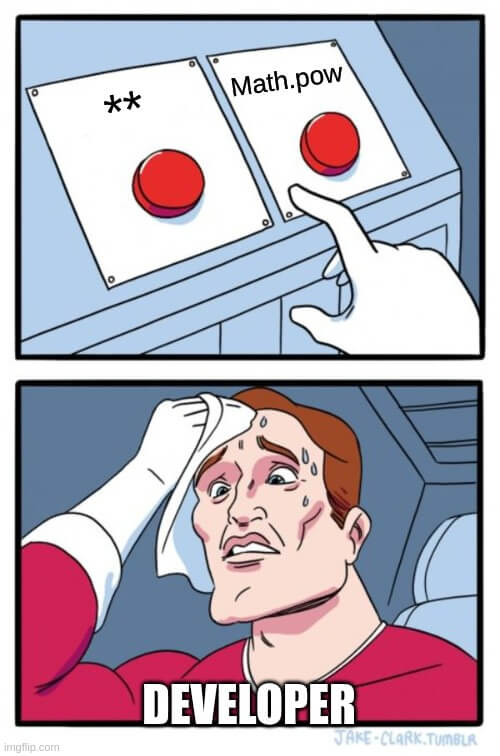
The exponentiation operator and the Math.pow function are equivalent, except that the exponentiation operator also accepts BigInts as arguments.
Final thoughts
In conclusion, using the JavaScript power operator is very easy, and you have multiple ways of using this operation. Most of the time, I use the Math.pow function because it is more verbose. However, I use the exponentiation operator if I need to work with BigInts.
Finally, here are some of my other JavaScript tutorials that I wrote: