Multiline String - The Ultimate JavaScript Guide
Often, developers need to create multiline strings in JavaScript. A multiline string provides better readability for developers and gives the ability to make edits more quickly. Since introducing template literals in the 2015 version of JavaScript, it has been easier than ever to create multiline strings.
To create a multiline string, create a template literal like so:
javascriptconst text = `line
new line2
new line3`;
// Outputs: "line\nnew line2\nnew line3"
console.log(text);
This article will explain how to create a multiline string in ES6 and ES5 and answer some of the most common questions.
Let’s get to it 😎.
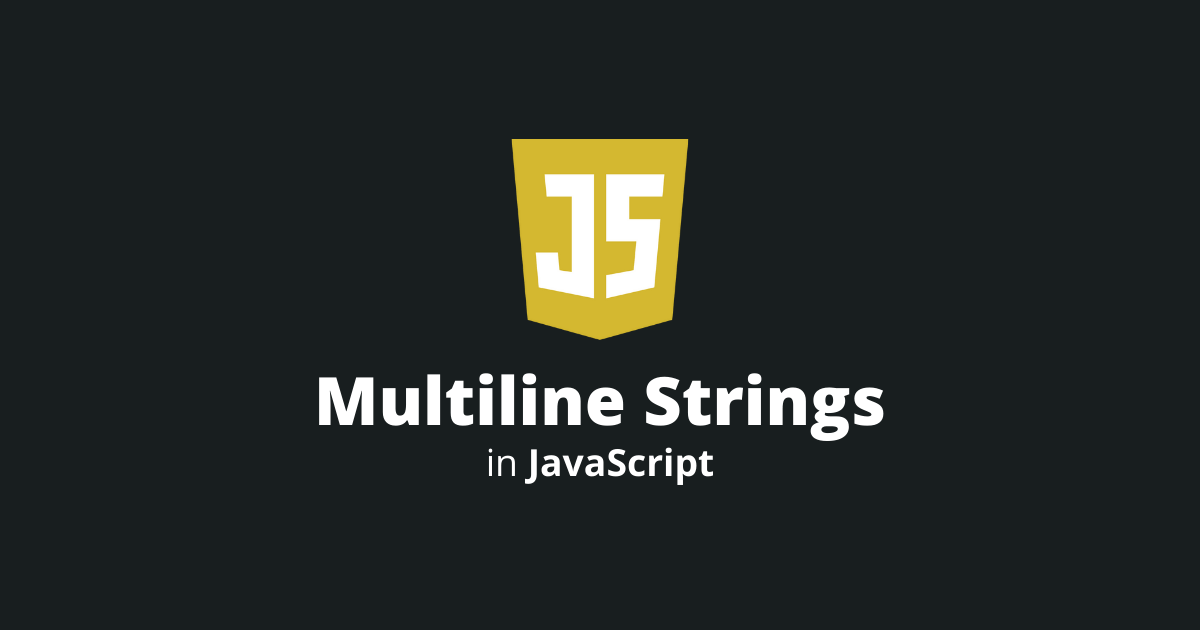
What is a template literal?
A template literal allows for:
- Multiline strings
- String interpolation
- Tagged templates
A template literal, also called template string, is delimited by backticks (`).
One significant advantage of template literals is the ability to use string interpolation.
String interpolation lets you use a placeholder in a template string. The syntax for a placeholder variable is ${variable}.
Here is an example of this:
javascriptconst name = "Tim";
const text = `My name is ${name}`;
// Outputs: "My name is Tim"
console.log(text);
Another advantage of using template literals is that developers do not need to escape single and double quotes.
How to create a multiline string?
In ES6, you can create a multiline string by using template strings.
Here is an example of this:
javascriptconst text = `line 1
line2
line3`;
// Outputs: "line 1\nline2\nline3"
console.log(text);
By default, it will add new lines at the end of each line.
How to create a multiline string without a newline?
To create a multiline string without newline characters, you need to use the line continuation \ character at the end of each line.
Here is an example of this:
javascriptconst text = `line \
new line 2 \
new line 3`;
// Outputs: "line new line 2 new line 3"
console.log(text);
How to create a multiline string without indentation?
To create a multiline string without indentation, you can use an external npm library.
For example, the dedent library.
Here is an example of the dedent library in action:
javascriptimport dedent from "dedent";
const first = dedent`
first line
* new item
* new item 2
end`;
The dedent library strips indentation from multiline strings.
How to create a multiline string in ES5?
Unfortunately, template literals are not supported in old browsers (for example, Internet Explorer).
Luckily, you have three other ways of creating multiline strings.
Newline escaping
You can create a multiline string by escaping newline characters with the \ character like so:
javascriptvar text = "line \
new line2 \
new line3";
// Outputs: 'line new line2 new line3'
console.log(text);
String concatenation
You can create a multiline string using string concatenation like so:
javascriptvar text = "line " +
"new line 2 " +
"new line 3";
// Outputs: 'line new line2 new line3'
console.log(text);
Array joining
You can create a multiline string using the Array built-in join function.
javascriptvar text = [
'line',
'new line 2',
'new line 3'
].join(' ');
// Outputs: 'line new line2 new line3'
console.log(text);
Final thoughts
As you can see, it is very easy to create a multiline string with template literals in ES6. If you are unlucky enough that you need to support Internet Explorer, use string concatenation to create a multiline string.
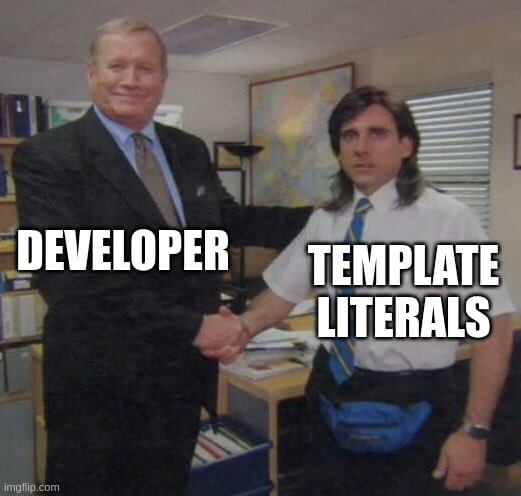
Here are other guides that I wrote (if you are interested in learning more about strings in JavaScript):