How to find the length of a string in JavaScript?
In JavaScript, developers oftentimes find themselves in situations where they need to find the length of a string. Whether it is for validation, to extract a substring, or for a conditional statement condition, developers need to know how to do it. Luckily for us, JavaScript provides a built-in property to easily find the length of a string.
To find the length of a string in JavaScript, use the length property.
javascriptconst str = 'Hello, my name is Tim';
// Outputs: 21
console.log(str.length);
In this article, I will explain, in detail, the String built-in length property, as well as show real-life examples.
Let’s get to it 😎.
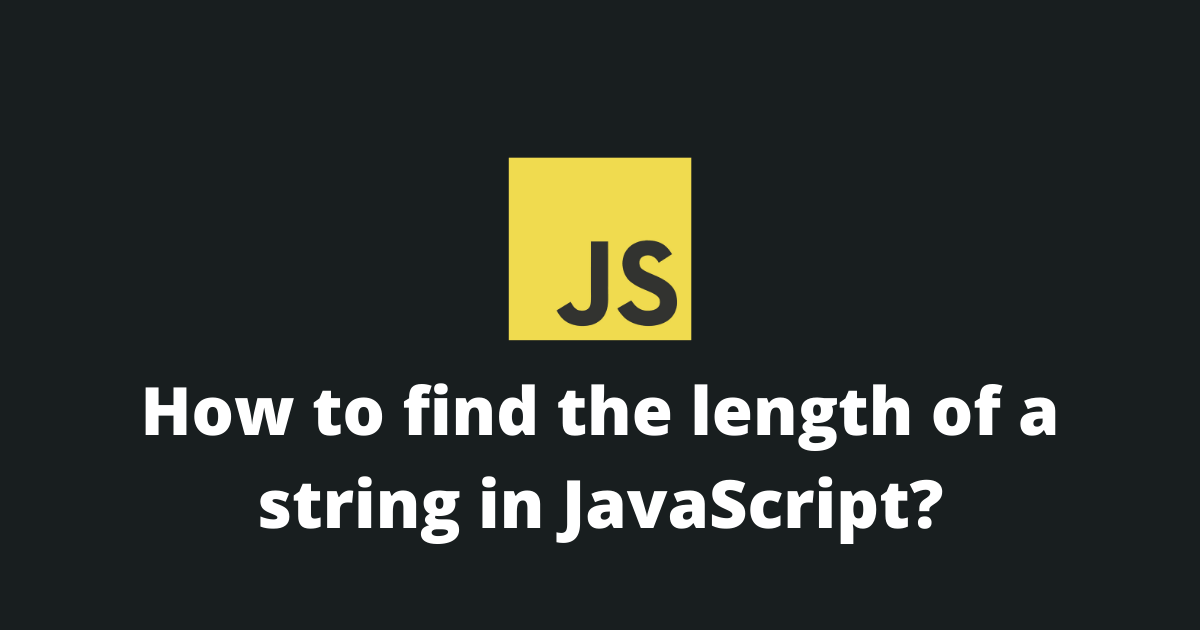
The definition
The length property returns the number of characters in a string (in UTF-16 code units).
If the string is empty, this property will return 0.
This is a read-only property.
Browser support
All major browsers support the length property 🥳. It is an ECMAScript1 feature (1997).
Example
Here is a small example of the length property in action:
javascriptconst str = 'Tim Mousk';
// Outputs: 9
console.log(str.length);
As you can see, the length property returns the number of characters in the str constant.
How to find the length without spaces?
To find the length of a string without spaces you can use a combination of the replace function with the length property.
javascriptconst str = 'This is a long statement';
// First, we remove all the spaces from the string.
const strWithoutSpaces = str.replace(/\s/g, '');
// Then, we use the length property.
// Outputs: 20
console.log(strWithoutSpaces.length);
Why does string length return undefined?
The only reason why the length property might return undefined is that the object on which you are calling this property IS NOT a string.
Make sure, that the object's type is a string by using the typeof operator.
Final thoughts
As you can see, finding the length of a string in JavaScript is super easy, you just need to use the length property, and that's it. The length value can then be used in your application logic, for example, to do validation.
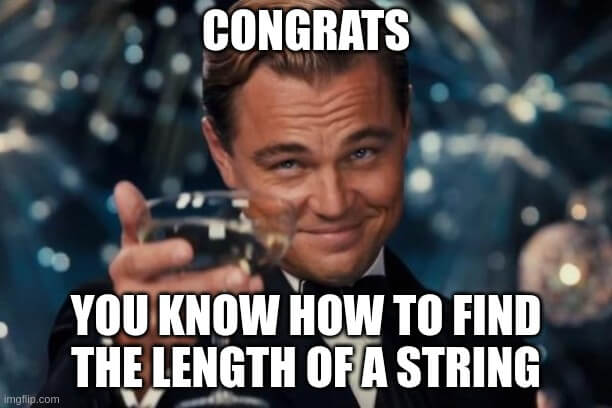
I have written more tutorials on JavaScript if you are interested in learning more about this language.
If you have any questions please ask them in the comments section.
I always check for new comments and answer them.
Thank you very much for reading this article.
Please share it with your fellow coders and colleagues.