How Does The Math.floor Function Work In JavaScript?
JavaScript has a lot of different useful built-in functions to help developers solve common problems (for example, parse a string into a number, round a number to two decimals place, and many more). One of those common tasks is when a developer needs to round a number down to its nearest integer. That's where the Math.floor function comes into play.
The JavaScript Math.floor function returns the largest integer less than or equal to the passed value.
javascript// Outputs: 2
console.log(Math.floor(2.03));
// Outputs: 3
console.log(Math.floor(3.6));
In this article, I will go over the Math.floor function, in detail, explain the difference between floor vs ceil, and show examples of how to use this function.
Let’s get to it 😎.
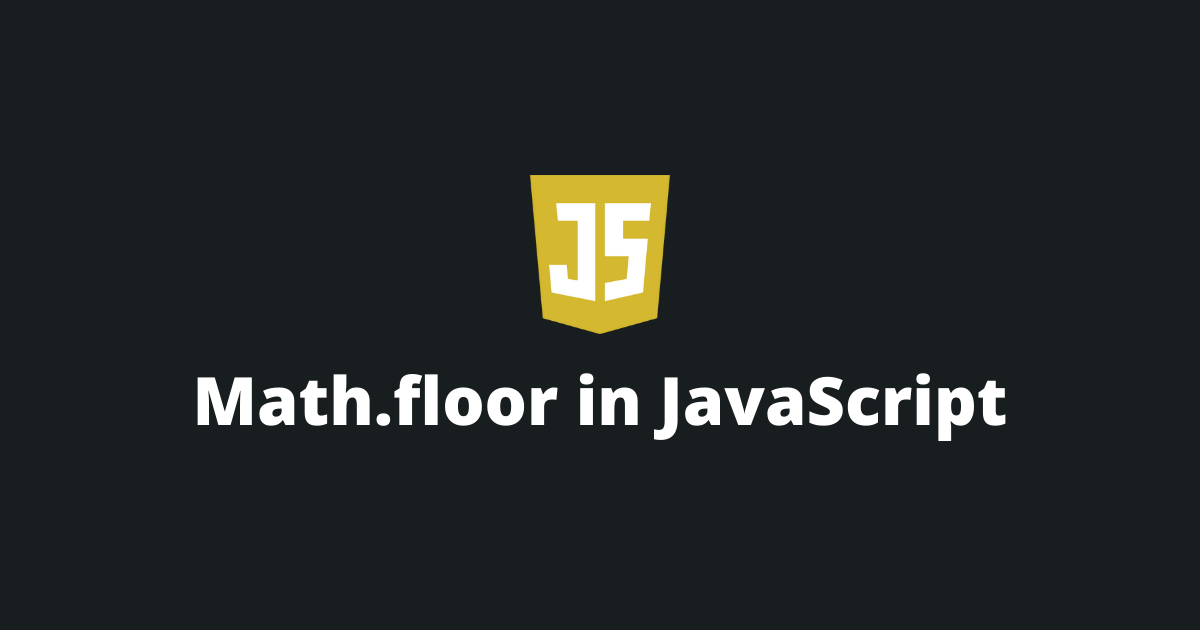
The definition
The JavaScript Math.floor function returns the greatest integer that is not greater than the passed value.
It is a built-in function of the Math object which contains a lot of mathematical helpers functions and constants.
The syntax
javascriptMath.floor(x)
This function accepts a number as the argument and returns an integer.
Browser support
All major browsers support the Math.floor function 🥳. It is an ECMAScript1 feature (1997).
Examples
Here is the Math.floor function in action:
javascript// Outputs: 2
console.log(Math.floor(2.032));
// Outputs: 5
console.log(Math.floor(5.99));
// Outputs: 2
console.log(Math.floor(2));
// It even works on negative numbers.
// Outputs: -3
console.log(Math.floor(-2.03));
Math.floor vs Math.ceil
In JavaScript Math.ceil is the opposite of Math.floor.
- Math.floor rounds DOWN to its nearest integer.
- Math.ceil rounds UP to its nearest integer.
Here is an example of how the behavior of those functions differs:
javascript// Outputs: 2
console.log(Math.floor(2.4));
// Outputs: 3
console.log(Math.ceil(2.4));
Math.floor vs Math.trunc
Even though those two functions can return the same results, they work completely differently.
- Math.floor rounds DOWN to its nearest integer.
- Math.trunc removes all the fractional digits from a number.
Here is an example of how the behavior of those functions differs:
javascript// Outputs: 2
console.log(Math.floor(2.4));
// Outputs: 2
console.log(Math.trunc(2.4));
// Outputs: 3
console.log(Math.floor(-2.4));
// Outputs: -2
console.log(Math.trunc(-2.4));
How to round a number to 2 decimal places
To round a number to 2 decimal places you can use this special formula.
Formula: Math.floor(x * 100) / 100
javascript// Outputs: 2.44
console.log(Math.floor(2.4425 * 100) / 100);
// Outputs: 3.49
console.log(Math.floor(3.49823 * 100) / 100);
Final thoughts
Well, here you have it.
Now you understand the Math.floor function, know the difference between ceil and floor, and will be able to use both functions when needed.
I have written more tutorials on JavaScript if you are interested in learning more about this language.
Thank you very much for reading this article.
Please share it with your fellow developers and colleagues.
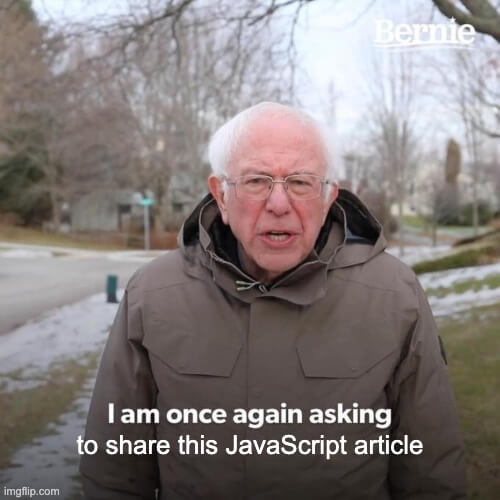